forked from root/public
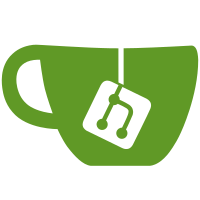
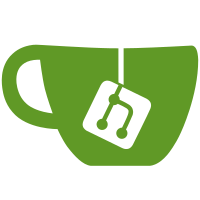
17 changed files with 440 additions and 63 deletions
@ -0,0 +1,281 @@
|
||||
# THE ADDITION OF EXERCISE PROCEDURE |
||||
|
||||
|
||||
##### This is for a go exercise in the piscine-go |
||||
|
||||
## **1. Writing the subject and / or writing the solution** |
||||
|
||||
Always address each exceptional cases. |
||||
|
||||
Example: [fprime](https://github.com/01-edu/public/blob/master/subjects/fprime.en.md). |
||||
|
||||
The exceptional cases in the `usage` part. |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/test$ go build |
||||
student@ubuntu:~/piscine-go/test$ ./test 225225 |
||||
3*3*5*5*7*11*13 |
||||
student@ubuntu:~/piscine-go/test$ ./test 8333325 |
||||
3*3*5*5*7*11*13*37 |
||||
|
||||
... |
||||
|
||||
student@ubuntu:~/piscine-go/test$ ./test 0 |
||||
|
||||
student@ubuntu:~/piscine-go/test$ ./test 1 |
||||
1 |
||||
student@ubuntu:~/piscine-go/test$ |
||||
``` |
||||
|
||||
The subject states that only **positive integer** will be tested, however, 0 and 1 are not primes. |
||||
|
||||
The subject writer made a mistake because of forgetting that fact. |
||||
|
||||
During the exam, the test was testing the `1` case and expecting a `1\n` to be printed. The real result should only have been a `\n`. |
||||
|
||||
Some students found this mistake. An update of the subject during the exam treating that special case was immediately necessary. |
||||
|
||||
1. Try to avoid the “you” and contracted “language” |
||||
|
||||
2. Always check the formating md |
||||
|
||||
------ |
||||
|
||||
### fprime <span style="color:#ff3234">(Title of the exercise)</span> |
||||
|
||||
#### Instructions <span style="color:#ff3234">(Instructions of the exercise)</span> |
||||
|
||||
|
||||
Write a program that takes a positive `int` and displays its prime factors, followed by a newline (`'\n'`). <span style="color:#ff3234">(general guidelines, notice the imperative style tense and the avoidance of “you”. “You” is authorized in the case of a presence of a back story where the player is immersed)</span> |
||||
|
||||
- Factors must be displayed in ascending order and separated by `*`. <span style="color:#ff3234">(formating requirement) </span> |
||||
|
||||
- If the number of parameters is different from 1, the program displays a newline. <span style="color:#ff3234">(special case requirement, this case will need to be tested)</span> |
||||
|
||||
- The input, when there is one, will always be valid. <span style="color:#ff3234">(Clarification on what the tester will do, hence giving the student guidelines on the cases to be handled, the tests have to reflect this instruction as well)</span> |
||||
|
||||
- In this exercise the primes factor of 1 is considered as 1. <span style="color:#ff3234">(Handling of exceptional case: THIS Happens to be a mistake, we will see uses this example for the “UPDATING A SUBJECT/TEST PROCEDURE”)</sapn> |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/test$ go build |
||||
student@ubuntu:~/piscine-go/test$ ./test 225225 |
||||
3*3*5*5*7*11*13 |
||||
student@ubuntu:~/piscine-go/test$ ./test 8333325 |
||||
3*3*5*5*7*11*13*37 |
||||
student@ubuntu:~/piscine-go/test$ ./test 9539 |
||||
9539 |
||||
student@ubuntu:~/piscine-go/test$ ./test 804577 |
||||
804577 |
||||
student@ubuntu:~/piscine-go/test$ ./test 42 |
||||
2*3*7 |
||||
student@ubuntu:~/piscine-go/test$ ./test a |
||||
|
||||
student@ubuntu:~/piscine-go/test$ ./test 0 |
||||
|
||||
student@ubuntu:~/piscine-go/test$ ./test 1 |
||||
1 |
||||
student@ubuntu:~/piscine-go/test$ |
||||
``` |
||||
|
||||
------ |
||||
|
||||
## **2. Creating the files for tests (4 main cases)** |
||||
|
||||
### always in -> *all/tests/go/* |
||||
|
||||
### **Folder organization** |
||||
|
||||
- Function exercise in a Quest `(strlen)` |
||||
- 2 files: |
||||
- strlen_test.go |
||||
- solutions/strlen.goz |
||||
|
||||
```console |
||||
go |
||||
| strlen_test.go |
||||
| |
||||
| __ solutions |
||||
| |-strlen.go (package solutions) |
||||
| |
||||
| __ student (the same thing as the solutions, just run "cp -aT solutions/ student/") |
||||
``` |
||||
|
||||
- Program exercise in a Quest `(doop)` |
||||
- 2 files |
||||
- doop_test.go |
||||
- solutions/doop/main.go |
||||
|
||||
```console |
||||
go |
||||
| doop_test.go |
||||
| |
||||
| |
||||
| __ solutions |
||||
| |__doop |
||||
| |-main.go (package main) |
||||
| |
||||
| __ student (the same thing as the solutions, just run "cp -aT solutions/ student/") |
||||
``` |
||||
|
||||
- Program exercise in the exam `(dooprog)` |
||||
- 2 files |
||||
- solutions/doopprog/main.go |
||||
- solutions/doopprog/doopprog_test.go |
||||
|
||||
```console |
||||
go |
||||
| |
||||
| __ solutions |
||||
| | __ doopprog |
||||
| |-main.go (package main) |
||||
| |-doopprog_test.go |
||||
| |
||||
| __ student (the same thing as the solutions, just run "cp -aT solutions/ student/") |
||||
``` |
||||
|
||||
- Function exercise in the exam `(atoiprog)` |
||||
- 3 files |
||||
- solutions/atoi.go |
||||
- solutions/atoiprog/main.go |
||||
- solutions/atoiprog/atoiprog_test.go |
||||
|
||||
```console |
||||
go |
||||
| |
||||
| __ solutions |
||||
| | |
||||
| |-atoi.go (package solutions) |
||||
| |__atoiprog |
||||
| |-main.go (package main)(func main(){} stays empty) |
||||
| |-atoiprog_test.go |
||||
| |
||||
| __ student (the same thing as the solutions, just run "cp -aT solutions/ student/") |
||||
``` |
||||
|
||||
------ |
||||
|
||||
## **3. Writing a file_test.go (test file for go)** |
||||
|
||||
### <span style="color:#00bae6">**RULE 1**</span> |
||||
|
||||
- Make the test as independent as possible (no self-made functions imported) |
||||
|
||||
- **If** the source is not in the import section, copy and paste the function, with **lowercase** for the first letter of its name. |
||||
|
||||
- Example: addprimesum_test.go |
||||
|
||||
 |
||||
|
||||
The func isAPrime is fully copied to the file. |
||||
|
||||
### <span style="color:#00bae6">**RULE 2**</span> |
||||
|
||||
Every special case in the subject should be tested. Preferably first. Before the randoms tests |
||||
|
||||
### <span style="color:#00bae6">**RULE 3**</span> |
||||
|
||||
Whenever possible do at least 1 random test! This is to avoid cheating by predictability of the tests. If the tests are fixed, then the student may create a forest of ifs program to bypass the tester. |
||||
|
||||
### z01.functions to be used by tester |
||||
|
||||
- Function exercise in a Quest (strlen)  |
||||
|
||||
```go |
||||
z01.Challenge(t, studentSol, studentStu) // if the program doesn’t have arguments |
||||
|
||||
z01.Challenge(t, studentSol, studentStu, args...) //if the program has arguments |
||||
``` |
||||
|
||||
- Program exercise in a Quest (doop) <- Screenshots to be added. |
||||
|
||||
```go |
||||
z01.ChallengeMain(t) // if the program doesn’t have arguments |
||||
|
||||
z01.ChallengeMain(t, args...) // if the program has arguments |
||||
``` |
||||
|
||||
- Program exercise in the exam (dooprog) Screenshots to be added. |
||||
|
||||
```go |
||||
z01.ChallengeMainExam(t) // if the program doesn’t have arguments |
||||
|
||||
z01.ChallengeMainExam (t, args...) // if the program has arguments |
||||
``` |
||||
|
||||
- Function exercise in the exam (Atoiprog) Screenshots to be added. |
||||
|
||||
```go |
||||
z01.Challenge(t, studentSol, studentStu) // if the program doesn’t have arguments |
||||
|
||||
z01.Challenge(t, studentSol, studentStu, args...) //if the program has arguments |
||||
``` |
||||
|
||||
------ |
||||
|
||||
## **4. Testing locally (`go test` or `go test -run=Test\<nameOfTheFunction\>`)** |
||||
|
||||
### you do -run=... because you have many test files, so you need to run just one |
||||
|
||||
### Before every PR : a go test has to be executed (in several situations) in the folder(s) of the exercise(s) worked on |
||||
|
||||
**First thing first** |
||||
|
||||
```console |
||||
rm -r student |
||||
cp -aT solutions/ student |
||||
``` |
||||
|
||||
### Execute a go test in the appropriate folder |
||||
|
||||
- Function exercise in a Quest `(strlen)`  |
||||
|
||||
`all/test/go` |
||||
|
||||
```console |
||||
go test -run=TestStrlen |
||||
``` |
||||
|
||||
- Program exercise in a Quest `(doop)` <- Screenshots to be added. |
||||
|
||||
`all/test/go/` |
||||
|
||||
```console |
||||
go test -run=TestDoop |
||||
``` |
||||
|
||||
- Program exercise in the exam `(dooprog)` Screenshots to be added. |
||||
|
||||
Here you can do just *go test*, because there's only one test file |
||||
|
||||
`all/test/go/student/dooprog` |
||||
|
||||
```console |
||||
go test |
||||
``` |
||||
|
||||
- Function exercise in the exam `(atoiprog)` Screenshots to be added. |
||||
|
||||
`all/test/go/student/atoiprog` |
||||
|
||||
```console |
||||
go test |
||||
``` |
||||
|
||||
|
||||
### **NOTE:** If a go test gives a (cached) result, use this type of command (example with raid3): |
||||
|
||||
```go test count=1 raid3_test.go``` |
||||
|
||||
The result should be an OK message: |
||||
|
||||
- This means that the test is running correctly when the correct solution is given. If this does not work, the test file is likely to have errors inside. |
||||
|
||||
- Time should be under 5-6 seconds. If longer, remove some of the iteration of the random tests (for example, less random tests) |
||||
|
||||
- Be watchful of exercises with challenge function: Always test a copy of the variable and not the same variable for both the student and the solution function. |
||||
|
||||
- Introduce errors in the student solution.go file in order to see the errors message and compare them to the subject examples. |
||||
|
||||
- Error messages for structures exercises (linked lists, binary trees) need to be tailored accordingly. |
@ -0,0 +1,14 @@
|
||||
#!/usr/bin/env bash |
||||
|
||||
# Install Docker |
||||
|
||||
script_dir="$(cd -P "$(dirname "$BASH_SOURCE")" && pwd)" |
||||
cd $script_dir |
||||
. set.sh |
||||
|
||||
apt-get -y install apt-transport-https ca-certificates curl gnupg-agent software-properties-common |
||||
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | apt-key add - |
||||
add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu disco stable" |
||||
apt-get update |
||||
apt-get -y install docker-ce docker-ce-cli containerd.io |
||||
adduser student docker |
@ -1,62 +1,83 @@
|
||||
#### Functional Project Questions |
||||
|
||||
##### Try passing as arguments `"left standard --align=right"` |
||||
###### Does it displays the correct result at the right side? |
||||
|
||||
###### Does it display the correct result at the right side? |
||||
|
||||
##### Try passing as arguments `"right standard --align=left"` |
||||
###### Does it displays the correct result at the left side? |
||||
|
||||
###### Does it display the correct result at the left side? |
||||
|
||||
##### Try passing as arguments `"hello shadow --align=center"` |
||||
###### Does it displays the correct result at the center? |
||||
|
||||
###### Does it display the correct result at the center? |
||||
|
||||
##### Try passing as arguments `""1 Two 4" shadow --align=justify"` |
||||
###### Does it displays the correct result justified? |
||||
|
||||
###### Does it display the correct result justified? |
||||
|
||||
##### Try passing as arguments `"23/32 standard --align=right"` |
||||
###### Does it displays the correct result at the right side? |
||||
|
||||
###### Does it display the correct result at the right side? |
||||
|
||||
##### Try passing as arguments `"ABCabc123 thinkertoy--align=right"` |
||||
###### Does it displays the correct result at the right side? |
||||
|
||||
###### Does it display the correct result at the right side? |
||||
|
||||
##### Try passing as arguments `"!#$%&" thinkertoy --align=cente"` |
||||
###### Does it displays the correct result at the center? |
||||
|
||||
###### Does it display the correct result at the center? |
||||
|
||||
##### Try passing as arguments `""23Hello World!" standard --align=left"` |
||||
###### Does it displays the correct result at the left side? |
||||
|
||||
###### Does it display the correct result at the left side? |
||||
|
||||
##### Try passing as arguments `""HELLO there HOW are YOU?!" thinkertoy --align=justify"` |
||||
###### Does it displays the correct result justified? |
||||
|
||||
###### Does it display the correct result justified? |
||||
|
||||
##### Try passing as arguments `""a -> A b -> B c -> C" shadow --align=right"` |
||||
###### Does it displays the correct result at the right side? |
||||
|
||||
###### Does it display the correct result at the right side? |
||||
|
||||
##### Try reducing the terminal window and run `"abcd shadow --align=right"` |
||||
|
||||
###### Does the representation adapt to the terminal size displaying the right result in the right side? |
||||
|
||||
##### Try reducing the terminal window and run `"ola standard --align=center"` |
||||
|
||||
###### Does the representation adapt to the terminal size displaying the right result in the center? |
||||
|
||||
##### Try passing as arguments a random string with lower and upper case letters, and the align flag ("--align=") followed by a random alignment (left, right, center or justify). |
||||
|
||||
###### Does it display the expected result? |
||||
|
||||
##### Try passing as arguments a random string with lower case letters, numbers and spaces, and the align flag ("--align=") followed by a random alignment (left, right, center or justify). |
||||
|
||||
###### Does it display the expected result? |
||||
|
||||
##### Try passing as arguments a random string with special characters, and the align flag ("--align=") followed by a random alignment (left, right, center or justify). |
||||
|
||||
###### Does it display the expected result? |
||||
|
||||
##### Try passing as arguments a random string with lower, upper case, spaces and numbers letters, and the align flag ("--align=") followed by a random alignment (left, right, center or justify). |
||||
|
||||
###### Does it display the expected result? |
||||
|
||||
#### Basic |
||||
|
||||
###### +Does the project runs quickly and effectively (Favoring of recursive, no unnecessary data requests, etc.)? |
||||
|
||||
###### +Is the output of the program well structured? Does any letter seems to be out of line? |
||||
|
||||
###### +Is there a test file for this code? |
||||
|
||||
###### +Are the tests checking each possible case? |
||||
|
||||
###### +Does the code obey the [good practices](https://public.01-edu.org/subjects/good-practices.en)? |
||||
|
||||
#### Social |
||||
|
||||
###### +Did you learn anything from this project? |
||||
|
||||
###### +Would you recommend/nominate this program as an example for the rest of the school? |
||||
|
@ -1,62 +1,81 @@
|
||||
#### Functional |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with no arguments. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the arguments: `<file name>`. |
||||
###### Does it displays the same file? |
||||
|
||||
###### Does it display the same file? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the arguments: `<directory name>`. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-l`. |
||||
###### Does it displays the same files and/or folders with the same display? |
||||
|
||||
###### Does it display the same files and/or folders with the same display? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the arguments: `-l <file name>`. |
||||
###### Does it displays the same file with the same display? |
||||
|
||||
###### Does it display the same file with the same display? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the arguments: `-l <directory name>`. |
||||
###### Does it displays the same files and/or folders with the same display? |
||||
|
||||
###### Does it display the same files and/or folders with the same display? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-l /usr/bin`. |
||||
###### Does it displays the same files and/or folders with the same display? Be aware of symbolic links. |
||||
|
||||
###### Does it display the same files and/or folders with the same display? Be aware of symbolic links. |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-R`, in a directory with folders in it. |
||||
###### Does it displays the same files and/or folders? |
||||
|
||||
###### Does it display the same files and/or folders? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-a`. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-r`. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-t`. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the flag: `-la`. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the arguments: `-l -t <directory name>`. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
##### Run both my-ls-1 and the system command `ls` with the arguments: `-lRr <directory name>`, in which the directory chosen contains folders. |
||||
###### Does it displays the same files and/or folders in the same order? |
||||
|
||||
###### Does it display the same files and/or folders in the same order? |
||||
|
||||
#### General |
||||
|
||||
###### +Does the program runs with colors as in the ls command? |
||||
|
||||
###### +Does the program has other flags except for the mandatory ones? |
||||
|
||||
##### Try running the program with `-R ~` and with the command time before the program name (ex: "time ./my-ls-1 -R ~"). |
||||
|
||||
###### +Is the real time less than 1,5 seconds? |
||||
|
||||
#### Basic |
||||
|
||||
###### +Does the code obey the [good practices](https://public.01-edu.org/subjects/good-practices.en)? |
||||
|
||||
###### +Is there a test file for this code? |
||||
|
||||
###### +Are the tests checking each possible case? |
||||
|
||||
#### Social |
||||
|
||||
###### +Did you learn anything from this project? |
||||
|
||||
###### +Would you recommend/nominate this program as an example for the rest of the school? |
||||
|
@ -1,72 +1,100 @@
|
||||
#### Functional |
||||
|
||||
##### Try to run `"./push_swap"`. |
||||
|
||||
###### Does it display nothing? |
||||
|
||||
##### Try to run `"./push_swap 2 1 3 6 5 8"`. |
||||
|
||||
###### Does it display a valid solution and less than 9 instructions? |
||||
|
||||
##### Try to run `"./push_swap 0 1 2 3 4 5"`. |
||||
|
||||
###### Does it display nothing? |
||||
|
||||
##### Try to run `"./push_swap 0 one 2 3"`. |
||||
|
||||
``` |
||||
Error |
||||
``` |
||||
|
||||
###### Does it display the right result as above? |
||||
|
||||
##### Try to run `"./push_swap 1 2 2 3"`. |
||||
|
||||
``` |
||||
Error |
||||
``` |
||||
|
||||
###### Does it display the right result as above? |
||||
|
||||
##### Try to run `"./push_swap <5 random numbers>"` with 5 random numbers instead of the tag. |
||||
###### Does it displays a valid solution and less than 12 instructions? |
||||
|
||||
###### Does it display a valid solution and less than 12 instructions? |
||||
|
||||
##### Try to run `"./push_swap <5 random numbers>"` with 5 different random numbers instead of the tag. |
||||
###### Does it still displays a valid solution and less than 12 instructions? |
||||
|
||||
##### Try to run `"./checker "` and input nothing. |
||||
|
||||
###### Does it display nothing? |
||||
|
||||
##### Try to run `"./checker 0 one 2 3"`. |
||||
|
||||
``` |
||||
Error |
||||
``` |
||||
|
||||
###### Does it display the right result as above? |
||||
|
||||
##### Try to run `"echo -e "sa\npb\nrrr\n" | ./checker 0 9 1 8 2 7 3 6 4 5"`. |
||||
|
||||
``` |
||||
KO |
||||
``` |
||||
|
||||
###### Does it display the right result as above? |
||||
|
||||
##### Try to run `"echo -e "pb\nra\npb\nra\nsa\nra\npa\npa\n" | ./checker 0 9 1 8 2"`. |
||||
|
||||
``` |
||||
OK |
||||
``` |
||||
|
||||
###### Does it display the right result as above? |
||||
|
||||
##### Try to run `"ARG = "4 67 3 87 23"; ./push_swap $ARG | ./checker $ARG"`. |
||||
|
||||
``` |
||||
OK |
||||
``` |
||||
|
||||
###### Does it display the right result as above? |
||||
|
||||
#### General |
||||
|
||||
##### Try to run `"$ARG= "<100 random numbers>"; ./push_swap $ARG"` with 100 random different numbers instead of the tag. |
||||
###### +Does it displays less than 700 commands? |
||||
|
||||
###### +Does it display less than 700 commands? |
||||
|
||||
##### Try to run `"$ARG= "<100 random numbers>; ./push_swap $ARG | ./checker $ARG"` with the same 100 random different numbers as before instead of the tag. |
||||
|
||||
``` |
||||
OK |
||||
``` |
||||
###### +Does it displays the right result as above? |
||||
|
||||
###### +Does it display the right result as above? |
||||
|
||||
#### Basic |
||||
|
||||
###### +Does the code obey the [good practices](https://public.01-edu.org/subjects/good-practices.en)? |
||||
|
||||
###### +Is there a test file for this code? |
||||
|
||||
###### +Are the tests checking each possible case? |
||||
|
||||
#### Social |
||||
|
||||
###### +Did you learn anything from this project? |
||||
|
||||
###### +Would you recommend/nominate this program as an example for the rest of the school? |
||||
|
Loading…
Reference in new issue