forked from root/public
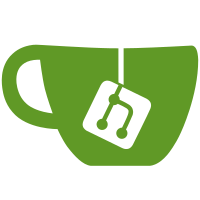
4 changed files with 106 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "tuples" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "tuples_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"tuples", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "tuples_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
tuples = { path = "../../../../rust-piscine-solutions/tuples"} |
@ -0,0 +1,35 @@
|
||||
// Define a tuple to represent a student
|
||||
// Each student is identified by an id number of type i32
|
||||
// Its name and its last name as a string
|
||||
// Print the content of the tuple to stdout
|
||||
|
||||
use tuples::*; |
||||
|
||||
fn main() { |
||||
let student = Student(20, "Pedro".to_string(), "Domingos".to_string()); |
||||
println!("Student: {:?}", student); |
||||
// After print only the first name
|
||||
println!("Student first name: {}", first_name(&student)); |
||||
// After print only the last name
|
||||
println!("Student last name: {}", last_name(&student)); |
||||
// After print only the id
|
||||
println!("Student Id: {}", id(&student)); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_id() { |
||||
let student = Student(20, String::from("Pedro"), String::from("Domingos")); |
||||
assert_eq!(id(&student), 20); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_first_name() { |
||||
let student = Student(20, String::from("Pedro"), String::from("Domingos")); |
||||
assert_eq!(first_name(&student), "Pedro".to_string()); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_last_name() { |
||||
let student = Student(20, String::from("Pedro"), String::from("Domingos")); |
||||
assert_eq!(last_name(&student), "Domingos".to_string()); |
||||
} |
@ -0,0 +1,49 @@
|
||||
## tuples |
||||
|
||||
### Instructions |
||||
|
||||
- Define a tuple structure to represent a student. |
||||
|
||||
- Each student is identified by an id number of type i32, his/her name and his/her last name as a string Print the content of the tuple to stdout |
||||
|
||||
- Then define three functions to return the id, first name and last name. |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
pub fn id(student: &Student) -> i32 { |
||||
} |
||||
|
||||
pub fn first_name(student: &Student) -> String { |
||||
} |
||||
|
||||
pub fn last_name(student: &Student) -> String { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test you're functions |
||||
|
||||
```rust |
||||
use tuples::*; |
||||
|
||||
fn main() { |
||||
let student = Student(20, "Pedro".to_string(), "Domingos".to_string()); |
||||
println!("Student: {:?}", student); |
||||
println!("Student first name: {}", first_name(&student)); |
||||
println!("Student last name: {}", last_name(&student)); |
||||
println!("Student Id: {}", id(&student)); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
Student: Student(20, "Pedro", "Domingos") |
||||
Student first name: Pedro |
||||
Student last name: Domingos |
||||
Student Id: 20 |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue