forked from root/public
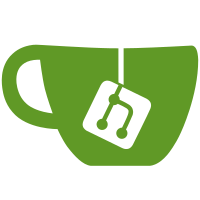
4 changed files with 232 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "vehicles" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "vehicles_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"vehicles", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "vehicles_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
vehicles = { path = "../../../../rust-piscine-solutions/vehicles"} |
@ -0,0 +1,131 @@
|
||||
// Create the trait Vehicle with the model and year
|
||||
|
||||
// In a border cross you want to keep a list of all the vehicles that
|
||||
// are waiting to enter the country. You want to keep a waiting list
|
||||
// of the vehicle but the vehicles can be of two types: Car or Truck
|
||||
// With the following structure:
|
||||
|
||||
// create a function that receives a vector of structures that
|
||||
// implement the Vehicle trait
|
||||
|
||||
use vehicles::{all_models, Car, Truck, Vehicle}; |
||||
|
||||
#[allow(dead_code)] |
||||
fn main() { |
||||
let vehicles: Vec<&dyn Vehicle> = vec![ |
||||
&Car { |
||||
plate_nbr: "A3D5C7", |
||||
model: "Model 3", |
||||
horse_power: 325, |
||||
year: 2010, |
||||
}, |
||||
&Truck { |
||||
plate_nbr: "V3D5CT", |
||||
model: "Ranger", |
||||
horse_power: 325, |
||||
year: 2010, |
||||
load_tons: 40, |
||||
}, |
||||
]; |
||||
println!("{:?}", all_models(vehicles)); |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
fn setup_cars<'a>() -> Vec<&'a dyn Vehicle> { |
||||
let cars: Vec<&dyn Vehicle> = vec![ |
||||
&Car { |
||||
plate_nbr: "A3D5C7", |
||||
model: "Model 3", |
||||
horse_power: 325, |
||||
year: 2010, |
||||
}, |
||||
&Car { |
||||
plate_nbr: "A785P7", |
||||
model: "S", |
||||
horse_power: 500, |
||||
year: 1980, |
||||
}, |
||||
&Car { |
||||
plate_nbr: "D325C7", |
||||
model: "300", |
||||
horse_power: 300, |
||||
year: 2000, |
||||
}, |
||||
&Car { |
||||
plate_nbr: "Z3KCH4", |
||||
model: "Montana", |
||||
horse_power: 325, |
||||
year: 2020, |
||||
}, |
||||
]; |
||||
cars |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
fn setup_trucks<'a>() -> Vec<&'a dyn Vehicle> { |
||||
let trucks: Vec<&dyn Vehicle> = vec![ |
||||
&Truck { |
||||
plate_nbr: "V3D5CT", |
||||
model: "Ranger", |
||||
horse_power: 325, |
||||
year: 2010, |
||||
load_tons: 40, |
||||
}, |
||||
&Truck { |
||||
plate_nbr: "V785PT", |
||||
model: "Silverado", |
||||
horse_power: 500, |
||||
year: 1980, |
||||
load_tons: 40, |
||||
}, |
||||
&Truck { |
||||
plate_nbr: "DE2SC7", |
||||
model: "Sierra", |
||||
horse_power: 300, |
||||
year: 2000, |
||||
load_tons: 40, |
||||
}, |
||||
&Truck { |
||||
plate_nbr: "3DH432", |
||||
model: "Cybertruck", |
||||
horse_power: 325, |
||||
year: 2020, |
||||
load_tons: 40, |
||||
}, |
||||
]; |
||||
trucks |
||||
} |
||||
|
||||
#[test] |
||||
fn all_car_models() { |
||||
let cars = setup_cars(); |
||||
assert_eq!(all_models(cars), ["Model 3", "S", "300", "Montana"]); |
||||
} |
||||
|
||||
#[test] |
||||
fn all_truck_models() { |
||||
let trucks = setup_trucks(); |
||||
assert_eq!( |
||||
all_models(trucks), |
||||
["Ranger", "Silverado", "Sierra", "Cybertruck"] |
||||
); |
||||
} |
||||
|
||||
#[test] |
||||
fn cars_and_trucks_models() { |
||||
let mut vehicles = setup_cars(); |
||||
vehicles.extend(setup_trucks()); |
||||
assert_eq!( |
||||
all_models(vehicles), |
||||
[ |
||||
"Model 3", |
||||
"S", |
||||
"300", |
||||
"Montana", |
||||
"Ranger", |
||||
"Silverado", |
||||
"Sierra", |
||||
"Cybertruck" |
||||
] |
||||
); |
||||
} |
@ -0,0 +1,79 @@
|
||||
## vehicles |
||||
|
||||
### Instructions |
||||
|
||||
- Create the trait Vehicle with the model and year |
||||
|
||||
- In a border cross you want to keep a list of all the vehicles that are waiting to enter the country. You want to keep a waiting list of the vehicle but the vehicles can be of two types: Car or Truck |
||||
|
||||
- Create a function that receives a vector of structures that implements the Vehicle trait and returns all the models. |
||||
|
||||
With the following structure: |
||||
|
||||
### Expected Functions and Structures |
||||
|
||||
```rust |
||||
#[allow(dead_code)] |
||||
pub struct Car<'a> { |
||||
pub plate_nbr: &'a str, |
||||
pub model: &'a str, |
||||
pub horse_power: u32, |
||||
pub year: u32, |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
pub struct Truck<'a> { |
||||
pub plate_nbr: &'a str, |
||||
pub model: &'a str, |
||||
pub horse_power: u32, |
||||
pub year: u32, |
||||
pub load_tons: u32, |
||||
} |
||||
|
||||
pub trait Vehicle { |
||||
fn model(&self) -> &str; |
||||
fn year(&self) -> u32; |
||||
} |
||||
|
||||
impl Vehicle for Truck<'_> { |
||||
} |
||||
|
||||
impl Vehicle for Car<'_> { |
||||
} |
||||
|
||||
fn all_models(list: Vec<&Vehicle>) -> Vec<&str> { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
fn main() { |
||||
let vehicles: Vec<&dyn Vehicle> = vec![ |
||||
&Car { |
||||
plate_nbr: "A3D5C7", |
||||
model: "Model 3", |
||||
horse_power: 325, |
||||
year: 2010, |
||||
}, |
||||
&Truck { |
||||
plate_nbr: "V3D5CT", |
||||
model: "Ranger", |
||||
horse_power: 325, |
||||
year: 2010, |
||||
load_tons: 40, |
||||
}, |
||||
]; |
||||
println!("{:?}", all_models(vehicles)); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
["Model 3", "Ranger"] |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue