forked from root/public
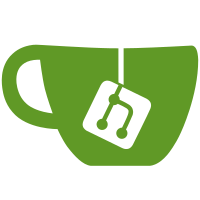
4 changed files with 265 additions and 0 deletions
@ -0,0 +1,93 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "cc" |
||||
version = "1.0.65" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "95752358c8f7552394baf48cd82695b345628ad3f170d607de3ca03b8dacca15" |
||||
|
||||
[[package]] |
||||
name = "fs_extra" |
||||
version = "1.2.0" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "2022715d62ab30faffd124d40b76f4134a550a87792276512b18d63272333394" |
||||
|
||||
[[package]] |
||||
name = "jemalloc-ctl" |
||||
version = "0.3.3" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "c502a5ff9dd2924f1ed32ba96e3b65735d837b4bfd978d3161b1702e66aca4b7" |
||||
dependencies = [ |
||||
"jemalloc-sys", |
||||
"libc", |
||||
"paste", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "jemalloc-sys" |
||||
version = "0.3.2" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "0d3b9f3f5c9b31aa0f5ed3260385ac205db665baa41d49bb8338008ae94ede45" |
||||
dependencies = [ |
||||
"cc", |
||||
"fs_extra", |
||||
"libc", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "jemallocator" |
||||
version = "0.3.2" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "43ae63fcfc45e99ab3d1b29a46782ad679e98436c3169d15a167a1108a724b69" |
||||
dependencies = [ |
||||
"jemalloc-sys", |
||||
"libc", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "libc" |
||||
version = "0.2.80" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "4d58d1b70b004888f764dfbf6a26a3b0342a1632d33968e4a179d8011c760614" |
||||
|
||||
[[package]] |
||||
name = "paste" |
||||
version = "0.1.18" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "45ca20c77d80be666aef2b45486da86238fabe33e38306bd3118fe4af33fa880" |
||||
dependencies = [ |
||||
"paste-impl", |
||||
"proc-macro-hack", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "paste-impl" |
||||
version = "0.1.18" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "d95a7db200b97ef370c8e6de0088252f7e0dfff7d047a28528e47456c0fc98b6" |
||||
dependencies = [ |
||||
"proc-macro-hack", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "proc-macro-hack" |
||||
version = "0.5.19" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "dbf0c48bc1d91375ae5c3cd81e3722dff1abcf81a30960240640d223f59fe0e5" |
||||
|
||||
[[package]] |
||||
name = "string_literal" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"jemalloc-ctl", |
||||
"jemallocator", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "string_literal_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"jemalloc-ctl", |
||||
"jemallocator", |
||||
"string_literal", |
||||
] |
@ -0,0 +1,12 @@
|
||||
[package] |
||||
name = "string_literal_test" |
||||
version = "0.1.0" |
||||
authors = ["lee <lee-dasilva@hotmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
jemalloc-ctl = "0.3.3" |
||||
jemallocator = "0.3.2" |
||||
string_literal = { path = "../../../../rust-piscine-solutions/string_literal"} |
@ -0,0 +1,107 @@
|
||||
/* |
||||
## string literal |
||||
|
||||
### Instructions |
||||
|
||||
Create the following functions: |
||||
|
||||
- `is_empty`, that returns true if a string is empty |
||||
- `is_ascii`, that returns true if all characters of a given string is in ASCII range |
||||
- `contains`, that returns true if the string contains a pattern given |
||||
- `split_at`, that divides a string in two returning a tuple |
||||
- `find', that returns the index if the first character of a given string that matches the pattern |
||||
|
||||
> This exercise will test the **heap allocation** of your function! |
||||
> So try your best to allocate the minimum data on the heap! (hit: &str) |
||||
|
||||
### Notions |
||||
|
||||
- https://doc.rust-lang.org/1.22.0/book/first-edition/the-stack-and-the-heap.html
|
||||
- https://doc.rust-lang.org/rust-by-example/primitives/literals.html
|
||||
|
||||
*/ |
||||
|
||||
#[global_allocator] |
||||
static ALLOC: jemallocator::Jemalloc = jemallocator::Jemalloc; |
||||
|
||||
#[allow(unused_imports)] |
||||
use jemalloc_ctl::{epoch, stats}; |
||||
#[allow(unused_imports)] |
||||
use string_literal::*; |
||||
|
||||
#[allow(dead_code)] |
||||
fn is_empty_sol(v: &str) -> bool { |
||||
v.is_empty() |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
fn is_ascii_sol(v: &str) -> bool { |
||||
v.is_ascii() |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
fn contains_sol(v: &str, pat: &str) -> bool { |
||||
v.contains(pat) |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
fn split_at_sol(v: &str, index: usize) -> (&str, &str) { |
||||
v.split_at(index) |
||||
} |
||||
|
||||
#[allow(dead_code)] |
||||
fn find_sol(v: &str, pat: char) -> usize { |
||||
v.find(pat).unwrap() |
||||
} |
||||
|
||||
#[test] |
||||
fn test_memory() { |
||||
// the statistics tracked by jemalloc are cached
|
||||
// The epoch controls when they are refreshed
|
||||
let e = epoch::mib().unwrap(); |
||||
// allocated: number of bytes allocated by the application
|
||||
let allocated = stats::allocated::mib().unwrap(); |
||||
|
||||
// sense we only use string literals (&str)
|
||||
// the heap will not be allocated, because
|
||||
// &str are preallocated text (saved on the binary file)
|
||||
is_empty_sol(""); |
||||
is_ascii_sol("rust"); |
||||
contains_sol("rust", "ru"); |
||||
split_at_sol("rust", 2); |
||||
find_sol("rust", 'u'); |
||||
// this will advance with the epoch giving the its old value
|
||||
// where we read the updated heap allocation using the `allocated.read()`
|
||||
e.advance().unwrap(); |
||||
let solution = allocated.read().unwrap(); |
||||
|
||||
is_empty(""); |
||||
is_ascii_sol("rust"); |
||||
contains("rust", "er"); |
||||
split_at("rust", 2); |
||||
find("rust", 'u'); |
||||
|
||||
e.advance().unwrap(); |
||||
let student = allocated.read().unwrap(); |
||||
|
||||
assert!( |
||||
student <= solution, |
||||
format!( |
||||
"your heap allocation is {}, and it must be less or equal to {}", |
||||
student, solution |
||||
) |
||||
); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_functions() { |
||||
assert!(is_empty("")); |
||||
assert!(!is_empty("something")); |
||||
assert!(is_ascii("rust")); |
||||
assert!(!is_ascii("rust¬")); |
||||
assert!(contains("rust", "ru")); |
||||
assert!(!contains("something", "mer")); |
||||
assert_eq!(split_at("rust", 2), ("ru", "st")); |
||||
assert_eq!(find("ru-st-e", '-'), 2); |
||||
assert_eq!(find("ru-st-e", 'e'), 6); |
||||
} |
@ -0,0 +1,53 @@
|
||||
## string literal |
||||
|
||||
### Instructions |
||||
|
||||
Create the following functions: |
||||
|
||||
- `is_empty`, that returns true if a string is empty |
||||
- `is_ascii`, that returns true if all characters of a given string is in ASCII range |
||||
- `contains`, that returns true if the string contains a pattern given |
||||
- `split_at`, that divides a string in two returning a tuple |
||||
- `find', that returns the index if the first character of a given string that matches the pattern |
||||
|
||||
> This exercise will test the **heap allocation** of your function! |
||||
> So try your best to allocate the minimum data on the heap! (hit: &str) |
||||
|
||||
### Notions |
||||
|
||||
- https://doc.rust-lang.org/1.22.0/book/first-edition/the-stack-and-the-heap.html |
||||
- https://doc.rust-lang.org/rust-by-example/primitives/literals.html |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
fn is_empty(v: &str) -> bool { |
||||
} |
||||
|
||||
fn is_ascii(v: &str) -> bool { |
||||
} |
||||
|
||||
fn contains(v: &str, pat: &str) -> bool { |
||||
} |
||||
|
||||
fn split_at(v: &str, index: usize) -> (&str, &str) { |
||||
} |
||||
|
||||
fn find(v: &str, pat: char) -> usize { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function |
||||
|
||||
```rust |
||||
|
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue