forked from root/public
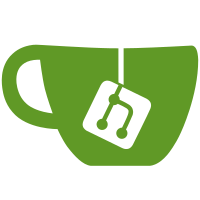
4 changed files with 114 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "hashing" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "hashing_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"hashing", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "hashing_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
hashing = { path = "../../../../rust-piscine-solutions/hashing"} |
@ -0,0 +1,41 @@
|
||||
// Given a list of integers (Vec<i32>) write three functions
|
||||
// Write a function called `mean` that calculates the `mean` (the average value) of all the values in the list
|
||||
// Write a function called `median` that calculates the `median` (for a sorted list is the value in the middle)
|
||||
// Write a function called `mode` that calculates the mode (the value
|
||||
// that appears more often)
|
||||
|
||||
use hashing::*; |
||||
|
||||
#[allow(dead_code)] |
||||
fn main() { |
||||
println!("Hello, world!"); |
||||
let v = vec![4, 7, 5, 2, 5, 1, 3]; |
||||
println!("mean {}", mean(&v)); |
||||
println!("median {}", median(&v)); |
||||
println!("mode {}", mode(&v)); |
||||
} |
||||
|
||||
use std::f64; |
||||
|
||||
#[allow(dead_code)] |
||||
fn approx_eq(a: f64, b: f64) -> bool { |
||||
(a - b).abs() < f64::EPSILON |
||||
} |
||||
|
||||
#[test] |
||||
fn test_mean() { |
||||
let v = vec![4, 7, 5, 2, 5, 1, 3]; |
||||
assert!(approx_eq(mean(&v), 3.857142857142857)); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_median() { |
||||
let v = vec![4, 7, 5, 2, 5, 1, 3]; |
||||
assert_eq!(median(&v), 4); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_mode() { |
||||
let v = vec![4, 7, 5, 2, 5, 1, 3]; |
||||
assert_eq!(mode(&v), 5); |
||||
} |
@ -0,0 +1,51 @@
|
||||
## hashing |
||||
|
||||
### Instructions |
||||
|
||||
Given a list of integers (Vec<i32>) write three functions |
||||
|
||||
Write a function called `mean` that calculates the `mean` (the average value) of all the values in the list |
||||
|
||||
Write a function called `median` that calculates the `median` (for a sorted list is the value in the middle) |
||||
|
||||
Write a function called `mode` that calculates the mode (the value |
||||
that appears more often) |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
fn mean(list: &Vec<i32>) -> f64 { |
||||
} |
||||
|
||||
fn median(list: &Vec<i32>) -> i32 { |
||||
} |
||||
|
||||
fn mode(list: &Vec<i32>) -> i32 { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
use hashing; |
||||
|
||||
fn main() { |
||||
println!("Hello, world!"); |
||||
let v = vec![4, 7, 5, 2, 5, 1, 3]; |
||||
println!("mean {}", hashing::mean(&v)); |
||||
println!("median {}", hashing::median(&v)); |
||||
println!("mode {}", hashing::mode(&v)); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
mean 3.857142857142857 |
||||
median 4 |
||||
mode 5 |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue