forked from root/public
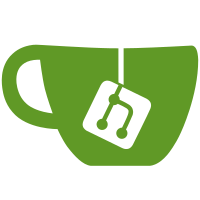
4 changed files with 231 additions and 0 deletions
@ -0,0 +1,38 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "borrow_me_the_reference" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"meval", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "borrow_me_the_reference_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"borrow_me_the_reference", |
||||
"meval", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "fnv" |
||||
version = "1.0.7" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "3f9eec918d3f24069decb9af1554cad7c880e2da24a9afd88aca000531ab82c1" |
||||
|
||||
[[package]] |
||||
name = "meval" |
||||
version = "0.2.0" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "f79496a5651c8d57cd033c5add8ca7ee4e3d5f7587a4777484640d9cb60392d9" |
||||
dependencies = [ |
||||
"fnv", |
||||
"nom", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "nom" |
||||
version = "1.2.4" |
||||
source = "registry+https://github.com/rust-lang/crates.io-index" |
||||
checksum = "a5b8c256fd9471521bcb84c3cdba98921497f1a331cbc15b8030fc63b82050ce" |
@ -0,0 +1,11 @@
|
||||
[package] |
||||
name = "borrow_me_the_reference_test" |
||||
version = "0.1.0" |
||||
authors = ["lee <lee-dasilva@hotmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
meval = "0.2" |
||||
borrow_me_the_reference = { path = "../../../../rust-piscine-solutions/borrow_me_the_reference"} |
@ -0,0 +1,127 @@
|
||||
/* |
||||
## borrowing_or_not_to_borrow |
||||
|
||||
### Instructions |
||||
|
||||
Ownership is Rust's most unique feature, and it enables Rust to make memory safety guarantees without |
||||
needing garbage collector. Therefore you must understand ownership in rust. |
||||
|
||||
Create the following functions : |
||||
|
||||
- `delete_and_backspace`, imagine that `-` represents the backspace key and the `+` represents the |
||||
delete key, this function must receive a borrowed string and turn this string into the string without |
||||
the backspaces and the deletes. Example: `delete_and_backspace("bpp--o+erroi-+cw") // output: "borrow"`
|
||||
- `is_correct` that borrows a Vector of string literals with some correct and incorrect math equations |
||||
and replaces the correct equations with `✔` and the wrong with `✘` and returns a `usize` with the percentage |
||||
of correct equations. |
||||
|
||||
|
||||
### Example |
||||
|
||||
```rust |
||||
fn main() { |
||||
let mut a = String::from("bpp--o+er+++sskroi-++lcw"); |
||||
let mut b: Vec<&str> = vec!["2+2=4", "3+2=5", "10-3=3", "5+5=10"]; |
||||
|
||||
// - If a value does **not implement Copy**, it must be **borrowed** and so will be passed by **reference**.
|
||||
delete_and_backspace(&mut a); // the reference of the value
|
||||
let per = is_correct(&mut b); // the reference of the value
|
||||
|
||||
println!("{:?}", (a, b, per)); |
||||
// output: ("borrow", ["✔", "✔", "✘", "✔"], 75)
|
||||
} |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- https://doc.rust-lang.org/book/ch04-00-understanding-ownership.html
|
||||
- https://docs.rs/meval/0.2.0/meval/
|
||||
|
||||
*/ |
||||
use borrow_me_the_reference::*; |
||||
//use meval::eval_str;
|
||||
|
||||
// fn d_a_b(s: String) -> String {
|
||||
// let mut new_string = String::new();
|
||||
// let mut count = 0;
|
||||
// for v in s.chars() {
|
||||
// if count != 0 && v != '+' {
|
||||
// count -= 1;
|
||||
// continue;
|
||||
// }
|
||||
// if v == '+' {
|
||||
// count += 1;
|
||||
// continue;
|
||||
// }
|
||||
// new_string.push(v);
|
||||
// if v == '-' {
|
||||
// new_string.pop();
|
||||
// new_string.pop();
|
||||
// }
|
||||
// }
|
||||
// new_string
|
||||
// }
|
||||
// - If a value does **not implement Copy**, it must be **borrowed** and so will be passed by **reference**.
|
||||
// this is not good in rust because strings are not to be manipulated like this
|
||||
// but its a good view for the students to see how memory works in rust
|
||||
// fn delete_and_backspace(s: &mut String) {
|
||||
// let a = d_a_b(s.clone());
|
||||
// s.clear();
|
||||
// s.push_str(&a);
|
||||
// }
|
||||
|
||||
// - If a value does **not implement Copy**, it must be **borrowed** and so will be passed by **reference**.
|
||||
// fn is_correct(v: &mut Vec<&str>) -> usize {
|
||||
// let mut percentage = 0;
|
||||
// for (i, equation) in v.clone().iter().enumerate() {
|
||||
// let a: Vec<&str> = equation.split('=').collect();
|
||||
// if eval_str(a[0]).unwrap() == a[1].parse::<f64>().unwrap() {
|
||||
// v[i] = "✔";
|
||||
// percentage += 1;
|
||||
// } else {
|
||||
// v[i] = "✘";
|
||||
// }
|
||||
// }
|
||||
// (percentage * 100) / v.len()
|
||||
// }
|
||||
|
||||
#[test] |
||||
fn reference_string() { |
||||
let mut a_1 = String::from("bpp--o+er+++sskroi-++lcw"); |
||||
let mut a_2 = String::from("hs-+deasdasd------l+++dsdp"); |
||||
let mut a_3 = String::from("pad-rtic+eulqw--+rar"); |
||||
delete_and_backspace(&mut a_1); |
||||
delete_and_backspace(&mut a_2); |
||||
delete_and_backspace(&mut a_3); |
||||
assert_eq!(a_1, "borrow".to_string()); |
||||
assert_eq!(a_2, "help".to_string()); |
||||
assert_eq!(a_3, "particular".to_string()); |
||||
} |
||||
#[test] |
||||
fn reference_vec() { |
||||
let mut b_1: Vec<&str> = vec!["2+2=4", "3+2=5", "10-3=3", "5+5=10"]; |
||||
let mut b_2: Vec<&str> = vec!["1+2=4", "0+2=5", "10-3=3", "41+5=10"]; |
||||
let mut b_3: Vec<&str> = vec!["2+2=4", "3+2=5", "10-3=7", "5+5=10"]; |
||||
let result_1 = is_correct(&mut b_1); |
||||
let result_2 = is_correct(&mut b_2); |
||||
let result_3 = is_correct(&mut b_3); |
||||
assert_eq!(result_1, 75); |
||||
assert_eq!(result_2, 0); |
||||
assert_eq!(result_3, 100); |
||||
|
||||
assert_eq!(b_1, vec!["✔", "✔", "✘", "✔"]); |
||||
assert_eq!(b_2, vec!["✘", "✘", "✘", "✘"]); |
||||
assert_eq!(b_3, vec!["✔", "✔", "✔", "✔"]); |
||||
} |
||||
|
||||
fn main() { |
||||
let mut a = String::from("bpp--o+er+++sskroi-++lcw"); |
||||
let mut b: Vec<&str> = vec!["2+2=4", "3+2=5", "10-3=3", "5+5=10"]; |
||||
|
||||
// - If a value does **not implement Copy**, it must be **borrowed** and so will be passed by **reference**.
|
||||
delete_and_backspace(&mut a); // the reference of the value
|
||||
let per = is_correct(&mut b); // the reference of the value
|
||||
|
||||
println!("{:?}", (a, b, per)); |
||||
// output: ("borrow", ["✔", "✔", "✘", "✔"], 75)
|
||||
} |
@ -0,0 +1,55 @@
|
||||
## borrow_me_the_reference |
||||
|
||||
### Instructions |
||||
|
||||
Ownership is Rust's most unique feature, and it enables Rust to make memory safety guarantees without |
||||
needing garbage collector. Therefore you must understand ownership in rust. |
||||
|
||||
Create the following functions : |
||||
|
||||
- `delete_and_backspace`, imagine that `-` represents the backspace key and the `+` represents the delete key, this function must receive a borrowed string and turn this string into the string without the backspaces and the deletes. |
||||
|
||||
- `is_correct` that borrows a Vector of string literals with some correct and incorrect math equations and replaces the correct equations with `✔` and the wrong with `✘` and returns a `usize` with the percentage of correct equations. |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
pub fn delete_and_backspace(s: &mut String) { |
||||
} |
||||
|
||||
pub fn is_correct(v: &mut Vec<&str>) -> usize { |
||||
} |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- https://doc.rust-lang.org/book/ch04-00-understanding-ownership.html |
||||
- https://docs.rs/meval/0.2.0/meval/ |
||||
|
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function |
||||
|
||||
```rust |
||||
use borrow_me_the_reference::{delete_and_backspace, is_correct}; |
||||
|
||||
fn main() { |
||||
let mut a = String::from("bpp--o+er+++sskroi-++lcw"); |
||||
let mut b: Vec<&str> = vec!["2+2=4", "3+2=5", "10-3=3", "5+5=10"]; |
||||
|
||||
// - If a value does **not implement Copy**, it must be **borrowed** and so will be passed by **reference**. |
||||
delete_and_backspace(&mut a); // the reference of the value |
||||
let per = is_correct(&mut b); // the reference of the value |
||||
|
||||
println!("{:?}", (a, b, per)); |
||||
// output: ("borrow", ["✔", "✔", "✘", "✔"], 75) |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue