forked from root/public
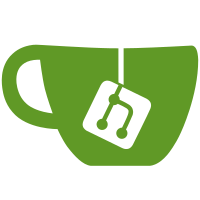
4 changed files with 84 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "collect" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "collect_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"collect", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "collect_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
collect = { path = "../../../../rust-piscine-solutions/collect"} |
@ -0,0 +1,25 @@
|
||||
// Implement the function bubble_sort which receives a vector Vec<i32>
|
||||
// and return the same vector but in increasing order using the bubble
|
||||
// sort algorithm
|
||||
|
||||
use collect::*; |
||||
|
||||
fn main() { |
||||
let ref mut v = vec![3, 2, 4, 5, 1, 7]; |
||||
let mut b = v.clone(); |
||||
bubble_sort(v); |
||||
println!("{:?}", v); |
||||
|
||||
b.sort(); |
||||
println!("{:?}", b); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_ordering() { |
||||
let ref mut v = vec![3, 2, 4, 5, 1, 7]; |
||||
let mut b = v.clone(); |
||||
|
||||
b.sort(); |
||||
bubble_sort(v); |
||||
assert_eq!(*v, b); |
||||
} |
@ -0,0 +1,37 @@
|
||||
## collect |
||||
|
||||
### Instructions |
||||
|
||||
Implement the function bubble_sort which receives a vector Vec<i32> and return the same vector but in increasing order using the bubble sort algorithm |
||||
|
||||
### Expected Function |
||||
|
||||
```rust |
||||
fn bubble_sort(vec: &mut Vec<i32>) { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
fn main() { |
||||
let ref mut v = vec![3, 2, 4, 5, 1, 7]; |
||||
let mut b = v.clone(); |
||||
bubble_sort(v); |
||||
println!("{:?}", v); |
||||
|
||||
b.sort(); |
||||
println!("{:?}", b); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
[1, 2, 3, 4, 5, 7] |
||||
[1, 2, 3, 4, 5, 7] |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue