forked from root/public
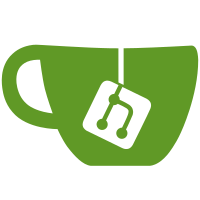
4 changed files with 96 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "lifetimes" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "lifetimes_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"lifetimes", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "lifetimes_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
lifetimes = { path = "../../../../rust-piscine-solutions/lifetimes"} |
@ -0,0 +1,34 @@
|
||||
// Create a struct called Person that has two fields: name of type
|
||||
// string slice (&str) and age of type u8
|
||||
// and create the associated function new which creates a new person
|
||||
// with age 0 and with the name given
|
||||
|
||||
use lifetimes::Person; |
||||
|
||||
fn main() { |
||||
let person = Person::new("Leo"); |
||||
|
||||
println!("Person = {:?}", person); |
||||
} |
||||
|
||||
#[cfg(test)] |
||||
mod tests { |
||||
use super::*; |
||||
|
||||
#[test] |
||||
fn fields() { |
||||
let person = Person { |
||||
name: "Dijkstra", |
||||
age: 10, |
||||
}; |
||||
assert_eq!(person.age, 10); |
||||
assert_eq!(person.name, "Dijkstra"); |
||||
} |
||||
|
||||
#[test] |
||||
fn create_person() { |
||||
let person = Person::new("Leo"); |
||||
assert_eq!(person.age, 0); |
||||
assert_eq!(person.name, "Leo"); |
||||
} |
||||
} |
@ -0,0 +1,40 @@
|
||||
## lifetimes |
||||
|
||||
### Instructions |
||||
|
||||
Create a struct called Person that has two fields: name of type string slice (&str) and age of type u8 and create the associated function new which creates a new person with age 0 and with the name given |
||||
|
||||
### Expected Functions and Data Structures |
||||
|
||||
```rust |
||||
#[derive(Debug)] |
||||
pub struct Person{ |
||||
pub name: &str, |
||||
pub age: u8, |
||||
} |
||||
|
||||
impl Person { |
||||
pub fn new(name: &str) -> Person { |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
fn main() { |
||||
let person = Person::new("Leo"); |
||||
|
||||
println!("Person = {:?}", person); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
Person = Person { name: "Leo", age: 0 } |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue