forked from root/public
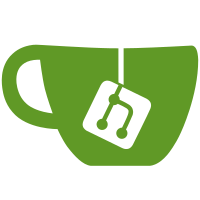
4 changed files with 136 additions and 0 deletions
@ -0,0 +1,19 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "lalgebra_scalar" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "matrix" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"lalgebra_scalar", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "matrix_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"matrix", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "matrix_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
matrix = { path = "../../../../rust-piscine-solutions/matrix"} |
@ -0,0 +1,51 @@
|
||||
// In this exercise you will define the basic operations with a matrix
|
||||
// starting by implementing the `std::ops::Add` trait
|
||||
|
||||
// Define the operation + (by defining the trait std::ops::Add) for
|
||||
// two matrices remember that two matrices can only be added if they
|
||||
// have the same size. Therefore the add method must handle the
|
||||
// possibility of failure by returning an Option<T>
|
||||
|
||||
use matrix::Matrix; |
||||
|
||||
fn main() { |
||||
let matrix = Matrix(vec![vec![8, 1], vec![9, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
println!("{:?}", matrix + matrix_2); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 3], vec![2, 5]]); |
||||
let matrix_2 = Matrix(vec![vec![3, 1], vec![1, 1]]); |
||||
println!("{:?}", matrix - matrix_2); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1, 3], vec![1, 1]]); |
||||
println!("{:?}", matrix - matrix_2); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 3], vec![9, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1, 3], vec![1, 1]]); |
||||
println!("{:?}", matrix + matrix_2); |
||||
} |
||||
|
||||
#[test] |
||||
fn addition() { |
||||
let matrix = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let expected = Matrix(vec![vec![2, 2], vec![2, 2]]); |
||||
assert_eq!(matrix + matrix_2, Some(expected)); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1, 3], vec![1, 1]]); |
||||
assert_eq!(matrix + matrix_2, None); |
||||
} |
||||
|
||||
#[test] |
||||
fn subtraction() { |
||||
let matrix = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let expected = Matrix(vec![vec![0, 0], vec![0, 0]]); |
||||
assert_eq!(matrix - matrix_2, Some(expected)); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1, 3], vec![1, 1]]); |
||||
assert_eq!(matrix - matrix_2, None); |
||||
} |
@ -0,0 +1,56 @@
|
||||
## matrix_ops |
||||
|
||||
### Instructions |
||||
|
||||
In this exercise you will define the basic operations with a matrix starting by implementing the `std::ops::Add` trait |
||||
|
||||
Define the operation + (by defining the trait std::ops::Add) for two matrices remember that two matrices can only be added if they have the same size. Therefore the add method must handle the possibility of failure by returning an Option<T> |
||||
|
||||
### Expected Function |
||||
|
||||
```rust |
||||
use std::ops::{ Add, Sub }; |
||||
|
||||
impl Add for Matrix { |
||||
|
||||
} |
||||
|
||||
impl Sub for Matrix { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function |
||||
|
||||
```rust |
||||
fn main() { |
||||
let matrix = Matrix(vec![vec![8, 1], vec![9, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
println!("{:?}", matrix + matrix_2); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 3], vec![2, 5]]); |
||||
let matrix_2 = Matrix(vec![vec![3, 1], vec![1, 1]]); |
||||
println!("{:?}", matrix - matrix_2); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 1], vec![1, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1, 3], vec![1, 1]]); |
||||
println!("{:?}", matrix - matrix_2); |
||||
|
||||
let matrix = Matrix(vec![vec![1, 3], vec![9, 1]]); |
||||
let matrix_2 = Matrix(vec![vec![1, 1, 3], vec![1, 1]]); |
||||
println!("{:?}", matrix + matrix_2); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
Some(Matrix([[9, 2], [10, 2]])) |
||||
Some(Matrix([[-2, 2], [1, 4]])) |
||||
None |
||||
None |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue