forked from root/public
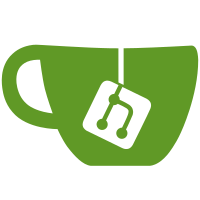
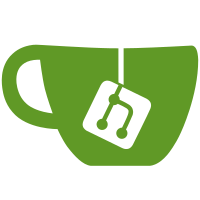
14 changed files with 2912 additions and 221 deletions
@ -1,42 +0,0 @@
|
||||
## ascii-art-reverse |
||||
|
||||
### Objectives |
||||
|
||||
Ascii-art-reverse consists on reversing the process, converting the graphic representation into a text. |
||||
|
||||
- You will have to create a text file with a graphic representation of a random phrase. |
||||
- The program will have **one** argument being the name of the file created. |
||||
- The program must print this phrase in **normal text**. |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ cat file.txt |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art file.txt |
||||
hello |
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
@ -0,0 +1,55 @@
|
||||
## ascii-art-fs |
||||
|
||||
### Objectives |
||||
|
||||
You must follow the same [instructions](https://github.com/01-edu/public/ascii-art.en.md) as in the first subject but the second argument must be the name of the template. |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
- You can see all about the **banners** [here](https://github.com/01-edu/public/ascii-art.en.md). |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" standard |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "Hello There" shadow |
||||
|
||||
_| _| _| _| _|_|_|_|_| _| _| |
||||
_| _| _|_| _| _| _|_| _| _|_|_| _|_| _| _|_| _|_| _| |
||||
_|_|_|_| _|_|_|_| _| _| _| _| _| _| _| _|_|_|_| _|_| _|_|_|_| _| |
||||
_| _| _| _| _| _| _| _| _| _| _| _| _| |
||||
_| _| _|_|_| _| _| _|_| _| _| _| _|_|_| _| _|_|_| _| |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "Hello There" thinkertoy |
||||
|
||||
o o o o o-O-o o |
||||
| | | | | | o |
||||
O--O o-o | | o-o | O--o o-o o-o o-o | |
||||
| | |-' | | | | | | | |-' | |-' o |
||||
o o o-o o o o-o o o o o-o o o-o |
||||
O |
||||
|
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
@ -0,0 +1,68 @@
|
||||
## ascii-art-justify |
||||
|
||||
### Objectives |
||||
|
||||
You must follow the same [instructions](https://github.com/01-edu/public/ascii-art.en.md) as in the first subject but the representation should be formatted using a **flag** `--align=<type>`, in which `type` can be : |
||||
|
||||
- center |
||||
- left |
||||
- right |
||||
- justify |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
|student@ubuntu:~/ascii-art$ go build | |
||||
|student@ubuntu:~/ascii-art$ ./ascii-art "hello" standard --align=center | |
||||
| _ _ _ | |
||||
| | | | | | | | |
||||
| | |__ ___ | | | | ___ | |
||||
| | _ \ / _ \ | | | | / _ \ | |
||||
| | | | | | __/ | | | | | (_) | | |
||||
| |_| |_| \___| |_| |_| \___/ | |
||||
| | |
||||
| | |
||||
|student@ubuntu:~/ascii-art$ ./ascii-art "Hello There" standard --align=left | |
||||
| _ _ _ _ _______ _ | |
||||
|| | | | | | | | |__ __| | | | |
||||
|| |__| | ___ | | | | ___ | | | |__ ___ _ __ ___ | |
||||
|| __ | / _ \ | | | | / _ \ | | | _ \ / _ \ | '__| / _ \ | |
||||
|| | | | | __/ | | | | | (_) | | | | | | | | __/ | | | __/ | |
||||
||_| |_| \___| |_| |_| \___/ |_| |_| |_| \___| |_| \___| | |
||||
| | |
||||
| | |
||||
|student@ubuntu:~/ascii-art$ ./ascii-art "hello" shadow --align=right | |
||||
| | |
||||
| _| _| _| | |
||||
| _|_|_| _|_| _| _| _|_| | |
||||
| _| _| _|_|_|_| _| _| _| _| | |
||||
| _| _| _| _| _| _| _| | |
||||
| _| _| _|_|_| _| _| _|_| | |
||||
| | |
||||
| | |
||||
|student@ubuntu:~/ascii-art$ ./ascii-art "hello" shadow --align=justify | |
||||
| | |
||||
|_| | |
||||
|_|_|_| _|_| _| _| _| _|_|_| _| _|_| _|_| _| _| _|_| _| _| | |
||||
|_| _| _| _| _| _| _| _| _| _|_| _|_|_|_| _| _| _| _| _| _| | |
||||
|_| _| _| _| _| _| _| _| _| _| _| _| _| _| _| _| _| _| | |
||||
|_| _| _|_| _| _| _|_|_| _| _|_|_| _|_|_| _|_| _|_|_| | |
||||
| _| | |
||||
| _|_| | |
||||
|student@ubuntu:~/ascii-art$ | |
||||
``` |
@ -0,0 +1,49 @@
|
||||
## ascii-art-output |
||||
|
||||
### Objectives |
||||
|
||||
- You must follow the same [instructions](https://github.com/01-edu/public/ascii-art.en.md) as in the first subject but writing the result into a file. |
||||
|
||||
- The file must be named by using the flag `--output=<fileName.txt>`, in which `--output` is the flag and `<fileName.txt>` is the file name. |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Learning about the choice of outputs. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" standard --output=banner.txt |
||||
student@ubuntu:~/ascii-art$ cat banner.txt |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "Hello There!" shadow --output=banner.txt |
||||
student@ubuntu:~/ascii-art$ cat banner.txt |
||||
|
||||
_| _| _| _| _|_|_|_|_| _| _| |
||||
_| _| _|_| _| _| _|_| _| _|_|_| _|_| _| _|_| _|_| _| |
||||
_|_|_|_| _|_|_|_| _| _| _| _| _| _| _| _|_|_|_| _|_| _|_|_|_| _| |
||||
_| _| _| _| _| _| _| _| _| _| _| _| _| |
||||
_| _| _|_|_| _| _| _|_| _| _| _| _|_|_| _| _|_|_| _| |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
@ -0,0 +1,59 @@
|
||||
## ascii-reverse-examples |
||||
|
||||
- Create your file and copy the examples into it. |
||||
|
||||
### example00 |
||||
|
||||
```console |
||||
_ _ _ _ __ __ _ _ |
||||
| | | | | | | | \ \ / / | | | | |
||||
| |__| | ___ | | | | ___ \ \ /\ / / ___ _ __ | | __| | |
||||
| __ | / _ \ | | | | / _ \ \ \/ \/ / / _ \ | '__| | | / _` | |
||||
| | | | | __/ | | | | | (_) | \ /\ / | (_) | | | | | | (_| | |
||||
|_| |_| \___| |_| |_| \___/ \/ \/ \___/ |_| |_| \__,_| |
||||
|
||||
|
||||
|
||||
``` |
||||
|
||||
### example01 |
||||
|
||||
```console |
||||
|
||||
_ ____ _____ |
||||
/ | |___ \ |___ / |
||||
| | __) | |_ \ |
||||
| | / __/ ___) | |
||||
|_| |_____| |____/ |
||||
|
||||
|
||||
|
||||
``` |
||||
|
||||
### example02 |
||||
|
||||
```console |
||||
___ |
||||
_ _ __ | _| |
||||
_| || |_ ______ \ \ | | |
||||
|_ __ _| |______| \ \ | | |
||||
_| || |_ ______ \ \ | | |
||||
|_ __ _| |______| \ \ | |_ |
||||
|_||_| \_\ |___| |
||||
|
||||
|
||||
``` |
||||
|
||||
### example03 |
||||
|
||||
```console |
||||
__ _ _ _ __ |
||||
/ / | | | | (_) __ _ ___ ____ _____ _ _ \ \ |
||||
| | ___ ___ _ __ | |_ | |__ _ _ __ / _` | ( _ ) |___ \ |___ / | || | | | |
||||
| | / __| / _ \ | '_ \ | __| | _ \ | | | '_ \ | (_| | / _ \/\ __) | |_ \ | || |_ | | |
||||
| | \__ \ | (_) | | | | | \ |_ | | | | | | | | | | \__, | | (_> < / __/ ___) | |__ _| | | |
||||
| | |___/ \___/ |_| |_| \__| |_| |_| |_| |_| |_| __/ | \___/\/ |_____| |____/ |_| | | |
||||
\_\ |___/ /_/ |
||||
|
||||
|
||||
``` |
@ -0,0 +1,44 @@
|
||||
## ascii-reverse |
||||
|
||||
### Objectives |
||||
|
||||
You must follow the same [instructions](https://github.com/01-edu/public/ascii-art.en.md) as in the first subject. |
||||
|
||||
- Ascii-reverse consists on reversing the process, converting the graphic representation into a text. |
||||
- You will have to create a text file containing a graphic representation of a random `string`. |
||||
- The argument will be a **flag**, `--reverse=<fileName>`, in which `--reverse` is the flag and `<fileName>` is the file name. |
||||
- The program must print this `string` in **normal text**. |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ cat file.txt |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art --reverse=file.txt |
||||
hello |
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
@ -0,0 +1,855 @@
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
_| |
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_|_|_|_|_| |
||||
_| _| |
||||
_|_|_|_|_| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_|_|_| |
||||
_|_| |
||||
_|_| |
||||
_|_|_| |
||||
_| |
||||
|
||||
|
||||
|
||||
_|_| _| |
||||
_|_| _| |
||||
_| |
||||
_| _|_| |
||||
_| _|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| _| |
||||
_|_| _| |
||||
_| _| |
||||
_|_| _| |
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
_| _| _| |
||||
_|_|_| |
||||
_|_|_|_|_| |
||||
_|_|_| |
||||
_| _| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_|_|_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_| |
||||
_| |
||||
_|_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_|_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_|_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_| |
||||
_| |
||||
_|_|_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_|_|_| |
||||
_| _| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_|_| |
||||
_| _| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_|_|_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
|
||||
|
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
|
||||
|
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
|
||||
_|_|_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| |
||||
_|_| |
||||
|
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
_| _| |
||||
_| _|_|_| _| |
||||
_| _| _| _| |
||||
_| _|_|_|_| |
||||
_| |
||||
_|_|_|_|_|_| |
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_|_|_|_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_|_|_| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_| |
||||
_| |
||||
_|_|_| |
||||
_| |
||||
_|_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_| |
||||
_| |
||||
_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_| _|_| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_|_|_|_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| _| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_|_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_|_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_|_| _|_| |
||||
_| _| _| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_|_| _| |
||||
_| _| _| |
||||
_| _|_| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_| _|_| |
||||
_| _| |
||||
_|_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_|_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| _| _| |
||||
_| _| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_|_|_|_|_| |
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_|_| |
||||
|
||||
|
||||
_| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_|_|_| |
||||
|
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_|_|_|_| |
||||
_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| |
||||
_|_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
_| |
||||
_|_| |
||||
|
||||
|
||||
_| |
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
_| |
||||
_| _| |
||||
_|_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| _|_| |
||||
_| _| _| |
||||
_| _| _| |
||||
_| _| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
_| _|_| |
||||
_|_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_|_|_| |
||||
_|_| |
||||
_|_| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
_| |
||||
_|_|_|_| |
||||
_| |
||||
_| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| _| _| |
||||
_| _| _| |
||||
_| _| _| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_|_| |
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
_| _| |
||||
_|_|_| |
||||
_| |
||||
_|_| |
||||
|
||||
|
||||
|
||||
_|_|_|_| |
||||
_| |
||||
_| |
||||
_|_|_|_| |
||||
|
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
_| |
||||
|
||||
|
||||
_| _| |
||||
_| _| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
@ -0,0 +1,856 @@
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
| | |
||||
| | |
||||
|_| |
||||
(_) |
||||
|
||||
|
||||
|
||||
_ _ |
||||
( | ) |
||||
V V |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ _ |
||||
_| || |_ |
||||
|_ __ _| |
||||
_| || |_ |
||||
|_ __ _| |
||||
|_||_| |
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
/ __) |
||||
\__ \ |
||||
( / |
||||
|_| |
||||
|
||||
|
||||
|
||||
_ __ |
||||
(_) / / |
||||
/ / |
||||
/ / |
||||
/ / _ |
||||
/_/ (_) |
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
( _ ) |
||||
/ _ \/\ |
||||
| (_> < |
||||
\___/\/ |
||||
|
||||
|
||||
|
||||
_ |
||||
( ) |
||||
|/ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
__ |
||||
/ / |
||||
| | |
||||
| | |
||||
| | |
||||
| | |
||||
\_\ |
||||
|
||||
|
||||
__ |
||||
\ \ |
||||
| | |
||||
| | |
||||
| | |
||||
| | |
||||
/_/ |
||||
|
||||
|
||||
_ |
||||
/\| |/\ |
||||
\ ` ' / |
||||
|_ _| |
||||
/ , . \ |
||||
\/|_|\/ |
||||
|
||||
|
||||
|
||||
|
||||
_ |
||||
_| |_ |
||||
|_ _| |
||||
|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ |
||||
( ) |
||||
|/ |
||||
|
||||
|
||||
|
||||
|
||||
______ |
||||
|______| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ |
||||
(_) |
||||
|
||||
|
||||
|
||||
__ |
||||
/ / |
||||
/ / |
||||
/ / |
||||
/ / |
||||
/_/ |
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
/ _ \ |
||||
| | | | |
||||
| |_| | |
||||
\___/ |
||||
|
||||
|
||||
|
||||
|
||||
_ |
||||
/ | |
||||
| | |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
|
||||
____ |
||||
|___ \ |
||||
__) | |
||||
/ __/ |
||||
|_____| |
||||
|
||||
|
||||
|
||||
|
||||
_____ |
||||
|___ / |
||||
|_ \ |
||||
___) | |
||||
|____/ |
||||
|
||||
|
||||
|
||||
|
||||
_ _ |
||||
| || | |
||||
| || |_ |
||||
|__ _| |
||||
|_| |
||||
|
||||
|
||||
|
||||
|
||||
____ |
||||
| ___| |
||||
|___ \ |
||||
__) | |
||||
|____/ |
||||
|
||||
|
||||
|
||||
|
||||
__ |
||||
/ / |
||||
| '_ \ |
||||
| (_) | |
||||
\___/ |
||||
|
||||
|
||||
|
||||
|
||||
_____ |
||||
|___ | |
||||
/ / |
||||
/ / |
||||
/_/ |
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
( _ ) |
||||
/ _ \ |
||||
| (_) | |
||||
\___/ |
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
/ _ \ |
||||
| (_) | |
||||
\__, | |
||||
/ / |
||||
/_/ |
||||
|
||||
|
||||
|
||||
_ |
||||
(_) |
||||
|
||||
_ |
||||
(_) |
||||
|
||||
|
||||
|
||||
|
||||
_ |
||||
(_) |
||||
|
||||
_ |
||||
( ) |
||||
|/ |
||||
|
||||
|
||||
__ |
||||
/ / |
||||
/ / |
||||
< < |
||||
\ \ |
||||
\_\ |
||||
|
||||
|
||||
|
||||
|
||||
______ |
||||
|______| |
||||
______ |
||||
|______| |
||||
|
||||
|
||||
|
||||
|
||||
__ |
||||
\ \ |
||||
\ \ |
||||
> > |
||||
/ / |
||||
/_/ |
||||
|
||||
|
||||
|
||||
___ |
||||
|__ \ |
||||
) | |
||||
/ / |
||||
|_| |
||||
(_) |
||||
|
||||
|
||||
|
||||
|
||||
____ |
||||
/ __ \ |
||||
/ / _` | |
||||
| | (_| | |
||||
\ \__,_| |
||||
\____/ |
||||
|
||||
|
||||
|
||||
/\ |
||||
/ \ |
||||
/ /\ \ |
||||
/ ____ \ |
||||
/_/ \_\ |
||||
|
||||
|
||||
|
||||
____ |
||||
| _ \ |
||||
| |_) | |
||||
| _ < |
||||
| |_) | |
||||
|____/ |
||||
|
||||
|
||||
|
||||
_____ |
||||
/ ____| |
||||
| | |
||||
| | |
||||
| |____ |
||||
\_____| |
||||
|
||||
|
||||
|
||||
_____ |
||||
| __ \ |
||||
| | | | |
||||
| | | | |
||||
| |__| | |
||||
|_____/ |
||||
|
||||
|
||||
|
||||
______ |
||||
| ____| |
||||
| |__ |
||||
| __| |
||||
| |____ |
||||
|______| |
||||
|
||||
|
||||
|
||||
______ |
||||
| ____| |
||||
| |__ |
||||
| __| |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
_____ |
||||
/ ____| |
||||
| | __ |
||||
| | |_ | |
||||
| |__| | |
||||
\_____| |
||||
|
||||
|
||||
|
||||
_ _ |
||||
| | | | |
||||
| |__| | |
||||
| __ | |
||||
| | | | |
||||
|_| |_| |
||||
|
||||
|
||||
|
||||
|
||||
_____ |
||||
|_ _| |
||||
| | |
||||
| | |
||||
_| |_ |
||||
|_____| |
||||
|
||||
|
||||
_ |
||||
| | |
||||
| | |
||||
_ | | |
||||
| |__| | |
||||
\____/ |
||||
|
||||
|
||||
|
||||
_ __ |
||||
| |/ / |
||||
| ' / |
||||
| < |
||||
| . \ |
||||
|_|\_\ |
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
| | |
||||
| | |
||||
| |____ |
||||
|______| |
||||
|
||||
|
||||
|
||||
__ __ |
||||
| \/ | |
||||
| \ / | |
||||
| |\/| | |
||||
| | | | |
||||
|_| |_| |
||||
|
||||
|
||||
|
||||
_ _ |
||||
| \ | | |
||||
| \| | |
||||
| . ` | |
||||
| |\ | |
||||
|_| \_| |
||||
|
||||
|
||||
|
||||
____ |
||||
/ __ \ |
||||
| | | | |
||||
| | | | |
||||
| |__| | |
||||
\____/ |
||||
|
||||
|
||||
|
||||
_____ |
||||
| __ \ |
||||
| |__) | |
||||
| ___/ |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
____ |
||||
/ __ \ |
||||
| | | | |
||||
| | | | |
||||
| |__| | |
||||
\___\_\ |
||||
|
||||
|
||||
|
||||
_____ |
||||
| __ \ |
||||
| |__) | |
||||
| _ / |
||||
| | \ \ |
||||
|_| \_\ |
||||
|
||||
|
||||
|
||||
_____ |
||||
/ ____| |
||||
| (___ |
||||
\___ \ |
||||
____) | |
||||
|_____/ |
||||
|
||||
|
||||
|
||||
_______ |
||||
|__ __| |
||||
| | |
||||
| | |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
_ _ |
||||
| | | | |
||||
| | | | |
||||
| | | | |
||||
| |__| | |
||||
\____/ |
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \ / / |
||||
\ \ / / |
||||
\ \/ / |
||||
\ / |
||||
\/ |
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \ / / |
||||
\ \ /\ / / |
||||
\ \/ \/ / |
||||
\ /\ / |
||||
\/ \/ |
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \ / / |
||||
\ V / |
||||
> < |
||||
/ . \ |
||||
/_/ \_\ |
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \ / / |
||||
\ \_/ / |
||||
\ / |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
______ |
||||
|___ / |
||||
/ / |
||||
/ / |
||||
/ /__ |
||||
/_____| |
||||
|
||||
|
||||
|
||||
___ |
||||
| _| |
||||
| | |
||||
| | |
||||
| | |
||||
| |_ |
||||
|___| |
||||
|
||||
|
||||
__ |
||||
\ \ |
||||
\ \ |
||||
\ \ |
||||
\ \ |
||||
\_\ |
||||
|
||||
|
||||
|
||||
___ |
||||
|_ | |
||||
| | |
||||
| | |
||||
| | |
||||
_| | |
||||
|___| |
||||
|
||||
|
||||
/\ |
||||
|/\| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
______ |
||||
|______| |
||||
|
||||
_ |
||||
( ) |
||||
\| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
__ _ |
||||
/ _` | |
||||
| (_| | |
||||
\__,_| |
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
| |__ |
||||
| '_ \ |
||||
| |_) | |
||||
|_.__/ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
/ __| |
||||
| (__ |
||||
\___| |
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
__| | |
||||
/ _` | |
||||
| (_| | |
||||
\__,_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
/ _ \ |
||||
| __/ |
||||
\___| |
||||
|
||||
|
||||
|
||||
__ |
||||
/ _| |
||||
| |_ |
||||
| _| |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
|
||||
__ _ |
||||
/ _` | |
||||
| (_| | |
||||
\__, | |
||||
__/ | |
||||
|___/ |
||||
|
||||
|
||||
_ |
||||
| | |
||||
| |__ |
||||
| _ \ |
||||
| | | | |
||||
|_| |_| |
||||
|
||||
|
||||
|
||||
_ |
||||
(_) |
||||
_ |
||||
| | |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
_ |
||||
(_) |
||||
_ |
||||
| | |
||||
| | |
||||
| | |
||||
_/ | |
||||
|__/ |
||||
|
||||
|
||||
_ |
||||
| | _ |
||||
| |/ / |
||||
| < |
||||
|_|\_\ |
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
| | |
||||
| | |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ __ ___ |
||||
| '_ ` _ \ |
||||
| | | | | | |
||||
|_| |_| |_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ __ |
||||
| '_ \ |
||||
| | | | |
||||
|_| |_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
/ _ \ |
||||
| (_) | |
||||
\___/ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ __ |
||||
| '_ \ |
||||
| |_) | |
||||
| .__/ |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
__ _ |
||||
/ _` | |
||||
| (_| | |
||||
\__, | |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
_ __ |
||||
| '__| |
||||
| | |
||||
|_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
___ |
||||
/ __| |
||||
\__ \ |
||||
|___/ |
||||
|
||||
|
||||
|
||||
_ |
||||
| | |
||||
| |_ |
||||
| __| |
||||
\ |_ |
||||
\__| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ _ |
||||
| | | | |
||||
| |_| | |
||||
\__,_| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \ / / |
||||
\ V / |
||||
\_/ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \ /\ / / |
||||
\ V V / |
||||
\_/\_/ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
__ __ |
||||
\ \/ / |
||||
> < |
||||
/_/\_\ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
_ _ |
||||
| | | | |
||||
| |_| | |
||||
\__, | |
||||
__/ / |
||||
|___/ |
||||
|
||||
|
||||
|
||||
____ |
||||
|_ / |
||||
/ / |
||||
/___| |
||||
|
||||
|
||||
|
||||
|
||||
__ |
||||
/ / |
||||
| | |
||||
/ / |
||||
\ \ |
||||
| | |
||||
\_\ |
||||
|
||||
|
||||
_ |
||||
| | |
||||
| | |
||||
| | |
||||
| | |
||||
| | |
||||
| | |
||||
|_| |
||||
|
||||
__ |
||||
\ \ |
||||
| | |
||||
\ \ |
||||
/ / |
||||
| | |
||||
/_/ |
||||
|
||||
|
||||
/\/| |
||||
|/\/ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
@ -0,0 +1,855 @@
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
o |
||||
|
||||
O |
||||
|
||||
|
||||
o o |
||||
| | |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
| | |
||||
-O-O- |
||||
| | |
||||
-O-O- |
||||
| | |
||||
|
||||
|
||||
|
||||
| | |
||||
-O-O- |
||||
o | | |
||||
-O-O- |
||||
| | o |
||||
-O-O- |
||||
| | |
||||
|
||||
|
||||
|
||||
O |
||||
o / |
||||
/ |
||||
/ o |
||||
O |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
/| |
||||
o-O- |
||||
| |
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
/ |
||||
o |
||||
| |
||||
o |
||||
\ |
||||
|
||||
|
||||
|
||||
|
||||
\ |
||||
o |
||||
| |
||||
o |
||||
/ |
||||
|
||||
|
||||
|
||||
|
||||
o | o |
||||
\|/ |
||||
--O-- |
||||
/|\ |
||||
o | o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
| |
||||
-o- |
||||
| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
O |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
/ |
||||
o |
||||
/ |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
o /o |
||||
| / | |
||||
o/ o |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
0 |
||||
/| |
||||
o | |
||||
| |
||||
o-o-o |
||||
|
||||
|
||||
|
||||
|
||||
-- |
||||
o o |
||||
/ |
||||
/ |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
oo |
||||
| |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
o--O |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| |
||||
o-o |
||||
| |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
/ |
||||
O--o |
||||
o | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o---o |
||||
/ |
||||
o |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| | |
||||
o-o |
||||
| | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| o |
||||
o--O |
||||
/ |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
O |
||||
|
||||
O |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
|
||||
o |
||||
| |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
/ |
||||
O |
||||
\ |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
\ |
||||
O |
||||
/ |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
o o |
||||
/ |
||||
o |
||||
|
||||
O |
||||
|
||||
|
||||
|
||||
o |
||||
/ \ |
||||
o O-o |
||||
\ |
||||
o- |
||||
|
||||
|
||||
|
||||
|
||||
O |
||||
/ \ |
||||
o---o |
||||
| | |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| | |
||||
O--o |
||||
| | |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
/ |
||||
O |
||||
\ |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| \ |
||||
| O |
||||
| / |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| |
||||
O-o |
||||
| |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| |
||||
O-o |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
o |
||||
| -o |
||||
o | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
O--O |
||||
| | |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o-O-o |
||||
| |
||||
| |
||||
| |
||||
o-O-o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
| |
||||
\ o |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| / |
||||
OO |
||||
| \ |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
| |
||||
| |
||||
O---o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
|\ /| |
||||
| O | |
||||
| | |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
|\ | |
||||
| \ | |
||||
| \| |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
o o |
||||
| | |
||||
o o |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| | |
||||
O--o |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
o o |
||||
| | |
||||
o O |
||||
o-O\ |
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| | |
||||
O-Oo |
||||
| \ |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
o-o |
||||
| |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
o-O-o |
||||
| |
||||
| |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
| | |
||||
| | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
o o |
||||
\ / |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
o o o |
||||
\ / \ / |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
\ / |
||||
O |
||||
/ \ |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
\ / |
||||
O |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o---o |
||||
/ |
||||
-O- |
||||
/ |
||||
o---o |
||||
|
||||
|
||||
|
||||
|
||||
O-o |
||||
| |
||||
| |
||||
| |
||||
O-o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
\ |
||||
o |
||||
\ |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-O |
||||
| |
||||
| |
||||
| |
||||
o-O |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
/ \ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o---o |
||||
|
||||
|
||||
|
||||
|
||||
0 |
||||
| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
oo |
||||
| | |
||||
o-o- |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
O-o |
||||
| | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
o-O |
||||
| | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
|-' |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
-O- |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o--o |
||||
| | |
||||
o--O |
||||
| |
||||
o--o |
||||
|
||||
|
||||
o |
||||
| |
||||
O--o |
||||
| | |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
|
||||
| |
||||
| |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
|
||||
o |
||||
| |
||||
o o |
||||
o-o |
||||
|
||||
|
||||
o |
||||
| / |
||||
OO |
||||
| \ |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
| |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-O-o |
||||
| | | |
||||
o o o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| | |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| | |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| | |
||||
O-o |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| | |
||||
o-O |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
\ |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
-o- |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
\ / |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o o o |
||||
\ / \ / |
||||
o o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
\ / |
||||
o |
||||
/ \ |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
o o |
||||
| | |
||||
o--O |
||||
| |
||||
o--o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
/ |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
o-O |
||||
| |
||||
o-o |
||||
|
||||
|
||||
|
||||
|
||||
o |
||||
| |
||||
o |
||||
| |
||||
o |
||||
|
||||
|
||||
|
||||
|
||||
o-o |
||||
| |
||||
O-o |
||||
| |
||||
o-o |
||||
|
||||
|
||||
|
||||
o_ / |
||||
/ o |
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
@ -1,46 +0,0 @@
|
||||
## ascii-art-fs |
||||
|
||||
### Objectives |
||||
|
||||
You must follow the same [instructions](https://github.com/01-edu/public/ascii-art.en.md) as the first subject but the second argument must be the name of the template. |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" standard |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" shadow |
||||
oooo oooo oooo |
||||
`888 `888 `888 |
||||
888 .oo. .ooooo. 888 888 .ooooo. |
||||
888P"Y88b d88' `88b 888 888 d88' `88b |
||||
888 888 888ooo888 888 888 888 888 |
||||
888 888 888 .o 888 888 888 888 |
||||
o888o o888o `Y8bod8P' o888o o888o `Y8bod8P' |
||||
|
||||
student@ubuntu:~/ascii-art$ |
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
@ -1,60 +0,0 @@
|
||||
## ascii-art-justify |
||||
|
||||
### Objectives |
||||
|
||||
You must follow the same [instructions](https://github.com/01-edu/public/ascii-art.en.md) as the first subject but the representation should be formatted using the second argument, that can be: |
||||
|
||||
- center |
||||
- justify |
||||
- align-left |
||||
- align-right |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
- You should build your one files with the templates you desire. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" standard center |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "Hello There" standard align-right |
||||
_ _ _ _ _______ _ |
||||
| | | | | | | | |__ __| | | |
||||
| |__| | ___ | | | | ___ | | | |__ ___ _ __ ___ |
||||
| __ | / _ \ | | | | / _ \ | | | _ \ / _ \ | '__| / _ \ |
||||
| | | | | __/ | | | | | (_) | | | | | | | | __/ | | | __/ |
||||
|_| |_| \___| |_| |_| \___/ |_| |_| |_| \___| |_| \___| |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" shadow center |
||||
oooo oooo oooo |
||||
`888 `888 `888 |
||||
888 .oo. .ooooo. 888 888 .ooooo. |
||||
888P"Y88b d88' `88b 888 888 d88' `88b |
||||
888 888 888ooo888 888 888 888 888 |
||||
888 888 888 .o 888 888 888 888 |
||||
o888o o888o `Y8bod8P' o888o o888o `Y8bod8P' |
||||
|
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
@ -1,51 +0,0 @@
|
||||
## ascii-art-output |
||||
|
||||
### Objectives |
||||
|
||||
Ascii-art-output consists on receiving two strings. The first argument will be converted into a graphic representation of ASCII and written into a file named by using the second argument. |
||||
|
||||
- In case the second argument is not present it should print the graphic representation. |
||||
|
||||
This project will help you learn about : |
||||
|
||||
- Client utilities. |
||||
- The Go file system(**fs**) API. |
||||
- Ways to receive data. |
||||
- Ways to output data. |
||||
- Manipulation of strings. |
||||
- Learning about the choice of outputs. |
||||
- Manipulation of structures. |
||||
|
||||
### Instructions |
||||
|
||||
- Your project must be written in **Go**. |
||||
- The code must respect the [**good practices**](https://github.com/01-edu/public/good-practices.en.md). |
||||
- It is recommended that the code should present a **test file**. |
||||
- You may use the same `banner` file. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/ascii-art$ go build |
||||
student@ubuntu:~/ascii-art$ ./ascii-art "hello" banner.txt |
||||
student@ubuntu:~/ascii-art$ cat banner.txt |
||||
_ _ _ |
||||
| | | | | | |
||||
| |__ ___ | | | | ___ |
||||
| _ \ / _ \ | | | | / _ \ |
||||
| | | | | __/ | | | | | (_) | |
||||
|_| |_| \___| |_| |_| \___/ |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ ./ascii-art "Hello There" banner.txt |
||||
student@ubuntu:~/ascii-art$ cat banner.txt |
||||
_ _ _ _ _______ _ |
||||
| | | | | | | | |__ __| | | |
||||
| |__| | ___ | | | | ___ | | | |__ ___ _ __ ___ |
||||
| __ | / _ \ | | | | / _ \ | | | _ \ / _ \ | '__| / _ \ |
||||
| | | | | __/ | | | | | (_) | | | | | | | | __/ | | | __/ |
||||
|_| |_| \___| |_| |_| \___/ |_| |_| |_| \___| |_| \___| |
||||
|
||||
|
||||
student@ubuntu:~/ascii-art$ |
||||
``` |
Loading…
Reference in new issue