forked from root/public
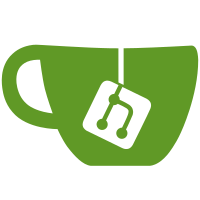
4 changed files with 110 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "groceries" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "groceries_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"groceries", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "groceries_test" |
||||
version = "0.1.0" |
||||
authors = ["MSilva95 <miguel-silva98@hotmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
groceries = { path = "../../../../rust-piscine-solutions/groceries" } |
@ -0,0 +1,43 @@
|
||||
// # Instructions
|
||||
|
||||
// Create a function called `insert`
|
||||
// fn insert(vec: &mut Vec<String>, val: String) that inserts a new element at the end of the Vec
|
||||
|
||||
#[allow(unused_imports)] |
||||
use groceries::*; |
||||
|
||||
#[allow(dead_code)] |
||||
fn main() { |
||||
let mut groceries = vec![ |
||||
"yogurt".to_string(), |
||||
"panetone".to_string(), |
||||
"bread".to_string(), |
||||
"cheese".to_string(), |
||||
]; |
||||
insert(&mut groceries, String::from("nuts")); |
||||
println!("The groceries list now = {:?}", &groceries); |
||||
println!( |
||||
"The second element of the grocery list is {:?}", |
||||
at_index(&groceries, 1) |
||||
); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_insertions() { |
||||
let mut groceries = Vec::new(); |
||||
insert(&mut groceries, "milk".to_string()); |
||||
assert_eq!(groceries, ["milk"]); |
||||
insert(&mut groceries, "bread".to_string()); |
||||
assert_eq!(groceries, ["milk", "bread"]); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_index() { |
||||
let groceries: Vec<String> = vec![ |
||||
"milk".to_string(), |
||||
"bread".to_string(), |
||||
"water".to_string(), |
||||
"wine".to_string(), |
||||
]; |
||||
assert_eq!(at_index(&groceries, 0), "milk"); |
||||
} |
@ -0,0 +1,45 @@
|
||||
## groceries |
||||
|
||||
### Instructions |
||||
|
||||
Create a function called `insert` that inserts a new element at the end of the Vec |
||||
|
||||
And another function `at_index` that returns the value found at the index passed as an argument |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
pub fn insert(vec: &mut Vec<String>, val: String) { |
||||
} |
||||
|
||||
pub fn at_index(vec: &Vec<String>, index: ) -> String { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
```rust |
||||
use groceries::{insert, at_index} |
||||
|
||||
fn main() { |
||||
let mut groceries = vec![ |
||||
"yogurt".to_string(), |
||||
"panetone".to_string(), |
||||
"bread".to_string(), |
||||
"cheese".to_string(), |
||||
]; |
||||
insert(&mut groceries, String::from("nuts")); |
||||
println!("The groceries list now = {:?}", &groceries); |
||||
println!( |
||||
"The second element of the grocery list is {:?}", |
||||
at_index(&groceries, 1) |
||||
); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```rust |
||||
The groceries list now = ["yogurt", "panetone", "bread", "cheese", "nuts"] |
||||
The second element of the grocery list is "panetone" |
||||
``` |
Loading…
Reference in new issue