forked from root/public
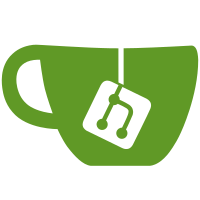
4 changed files with 132 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "slices_to_map" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "slices_to_map_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"slices_to_map", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "slices_to_map_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
slices_to_map = { path = "../../../../rust-piscine-solutions/slices_to_map"} |
@ -0,0 +1,78 @@
|
||||
// # Instructions:
|
||||
// Create a function that borrows two slices (&[T]) and returns a hashmap where
|
||||
// the first slice represents the keys and the second represents the values.
|
||||
|
||||
// The signature of the function is the following
|
||||
// fn slices_to_map<'a, T: Hash + Eq, U>(keys: &'a [T], values: &'a [U]) -> HashMap<&'a T, &'a U> {
|
||||
|
||||
// # Example:
|
||||
// for the slices &["hello", "how", "are", "you"] &[1, 3, 5, 8]
|
||||
// returns the hashmap ["hello": 1, "how": 3, "are": 5, "you":8]
|
||||
|
||||
use slices_to_map::*; |
||||
#[allow(unused_imports)] |
||||
use std::collections::HashMap; |
||||
|
||||
#[allow(dead_code)] |
||||
fn main() { |
||||
let keys = ["Olivia", "Liam", "Emma", "Noah", "James"]; |
||||
let values = [1, 3, 23, 5, 2]; |
||||
println!("{:?}", slices_to_map(&keys, &values)); |
||||
} |
||||
|
||||
#[cfg(test)] |
||||
mod tests { |
||||
use super::*; |
||||
|
||||
#[test] |
||||
fn test_same_length() { |
||||
let keys = ["Olivia", "Liam", "Emma", "Noah", "James"]; |
||||
let values = [1, 3, 23, 5, 2]; |
||||
let mut expected = HashMap::new(); |
||||
expected.insert(&"Olivia", &1); |
||||
expected.insert(&"Liam", &3); |
||||
expected.insert(&"Emma", &23); |
||||
expected.insert(&"Noah", &5); |
||||
expected.insert(&"James", &2); |
||||
assert_eq!(slices_to_map(&keys, &values), expected); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_different_length() { |
||||
let keys = ["Olivia", "Liam", "Emma", "Noah", "James"]; |
||||
let values = [1, 3, 23, 5, 2, 9]; |
||||
let mut expected = HashMap::new(); |
||||
expected.insert(&"Olivia", &1); |
||||
expected.insert(&"Liam", &3); |
||||
expected.insert(&"Emma", &23); |
||||
expected.insert(&"Noah", &5); |
||||
expected.insert(&"James", &2); |
||||
assert_eq!(slices_to_map(&keys, &values), expected); |
||||
|
||||
let keys = ["Olivia", "Liam", "Emma", "Noah", "James", "Isabella"]; |
||||
let values = [1, 3, 23, 5, 2]; |
||||
assert_eq!(slices_to_map(&keys, &values), expected); |
||||
} |
||||
|
||||
#[test] |
||||
fn it_works_for_vecs() { |
||||
let mut expected = HashMap::new(); |
||||
expected.insert(&"Olivia", &1); |
||||
expected.insert(&"Liam", &3); |
||||
expected.insert(&"Emma", &23); |
||||
expected.insert(&"Noah", &5); |
||||
expected.insert(&"James", &2); |
||||
|
||||
let keys = ["Olivia", "Liam", "Emma", "Noah", "James"]; |
||||
let values = [1, 3, 23, 5, 2]; |
||||
|
||||
assert_eq!(slices_to_map(&keys, &values), expected); |
||||
let keys = vec!["Olivia", "Liam", "Emma", "Noah", "James"]; |
||||
let values = vec![1, 3, 23, 5, 2, 9]; |
||||
assert_eq!(slices_to_map(&keys, &values), expected); |
||||
|
||||
let keys = vec!["Olivia", "Liam", "Emma", "Noah", "James", "Isabella"]; |
||||
let values = vec![1, 3, 23, 5, 2]; |
||||
assert_eq!(slices_to_map(&keys, &values), expected); |
||||
} |
||||
} |
@ -0,0 +1,32 @@
|
||||
## slices_to_map |
||||
|
||||
### Instructions: |
||||
|
||||
Create a function that borrows two slices and returns a hashmap where the first slice represents the keys and the second represents the values. |
||||
|
||||
### Expected Function |
||||
|
||||
```rust |
||||
pub fn slices_to_map(&[T], &[U]) -> HashMap<&T, &U> { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
fn main() { |
||||
let keys = ["Olivia", "Liam", "Emma", "Noah", "James"]; |
||||
let values = [1, 3, 23, 5, 2]; |
||||
println!("{:?}", slices_to_map(&keys, &values)); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
{"Liam": 3, "James": 2, "Emma": 23, "Noah": 5, "Olivia": 1} |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue