forked from root/public
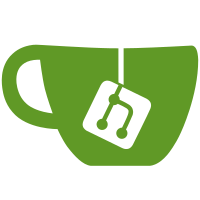
4 changed files with 184 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "copy" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "copy_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"copy", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "copy_test" |
||||
version = "0.1.0" |
||||
authors = ["lee <lee-dasilva@hotmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
copy = { path = "../../../../rust-piscine-solutions/copy"} |
@ -0,0 +1,105 @@
|
||||
/* |
||||
## copy |
||||
|
||||
### Instructions |
||||
|
||||
Your objective is to fix the program so that all functions work. |
||||
|
||||
- `nbr_function`, returns a tuple with the original value, the exponential and logarithm of that value |
||||
- `str_function`, returns a tuple with the original value and the exponential of that value as a string |
||||
- `vec_function`, returns a tuple with the original value and the logarithm of each value |
||||
|
||||
The objective is to now how ownership works with different types. |
||||
|
||||
### Example |
||||
|
||||
```rust |
||||
fn main() { |
||||
let a = String::from("1 2 4 5 6"); |
||||
let b = vec![1, 2, 4, 5]; |
||||
let c: u32 = 0; |
||||
|
||||
println!("{:?}", nbr_function(c)); |
||||
// output: (12, 162754.79141900392, 2.4849066497880004)
|
||||
println!("{:?}", vec_function(b)); |
||||
// output: ([1, 2, 4], [0.0, 0.6931471805599453, 1.3862943611198906])
|
||||
println!("{:?}", str_function(a)); |
||||
// output: ("1 2 4", "2.718281828459045 7.38905609893065 54.598150033144236")
|
||||
} |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- https://doc.rust-lang.org/rust-by-example/scope/move.html
|
||||
*/ |
||||
|
||||
use copy::*; |
||||
|
||||
#[allow(dead_code)] |
||||
fn main() { |
||||
let a = String::from("1 2 4 5 6"); |
||||
let b = vec![1, 2, 4, 5]; |
||||
let c: u32 = 0; |
||||
|
||||
println!("{:?}", nbr_function(c)); |
||||
// output: (12, 162754.79141900392, 2.4849066497880004)
|
||||
println!("{:?}", vec_function(b)); |
||||
// output: ([1, 2, 4], [0.0, 0.6931471805599453, 1.3862943611198906])
|
||||
println!("{:?}", str_function(a)); |
||||
// output: ("1 2 4", "2.718281828459045 7.38905609893065 54.598150033144236")
|
||||
} |
||||
|
||||
#[test] |
||||
fn ownership_nbr_test() { |
||||
assert_eq!( |
||||
nbr_function(12), |
||||
(12, 162754.79141900392, 2.4849066497880004) |
||||
); |
||||
assert_eq!(nbr_function(1), (1, 2.718281828459045, 0.0)); |
||||
assert_eq!(nbr_function(0), (0, 1.0, std::f64::INFINITY)); |
||||
} |
||||
|
||||
#[test] |
||||
fn ownership_vec_test() { |
||||
assert_eq!( |
||||
vec_function(vec![1, 2, 4]), |
||||
( |
||||
vec![1, 2, 4], |
||||
vec![0.0, 0.6931471805599453, 1.3862943611198906] |
||||
) |
||||
); |
||||
assert_eq!( |
||||
vec_function(vec![0, 1]), |
||||
(vec![0, 1], vec![std::f64::INFINITY, 0.0]) |
||||
); |
||||
assert_eq!( |
||||
vec_function(vec![1, 2, 4, 5]), |
||||
( |
||||
vec![1, 2, 4, 5], |
||||
vec![ |
||||
0.0, |
||||
0.6931471805599453, |
||||
1.3862943611198906, |
||||
1.6094379124341003 |
||||
] |
||||
) |
||||
); |
||||
} |
||||
|
||||
#[test] |
||||
fn ownership_str_test() { |
||||
assert_eq!( |
||||
str_function(String::from("1 2 4")), |
||||
( |
||||
"1 2 4".to_string(), |
||||
"2.718281828459045 7.38905609893065 54.598150033144236".to_string() |
||||
) |
||||
); |
||||
assert_eq!( |
||||
str_function(String::from("1 0")), |
||||
(("1 0".to_string(), "2.718281828459045 1".to_string())) |
||||
); |
||||
assert_eq!(str_function( |
||||
String::from("1 2 4 5 6")), |
||||
(("1 2 4 5 6".to_string(), "2.718281828459045 7.38905609893065 54.598150033144236 148.4131591025766 403.4287934927351".to_string()))); |
||||
} |
@ -0,0 +1,57 @@
|
||||
## copy |
||||
|
||||
### Instructions |
||||
|
||||
Your objective is to fix the program so that all functions work. |
||||
|
||||
- `nbr_function`, returns a tuple with the original value, the exponential and logarithm of that value |
||||
- `str_function`, returns a tuple with the original value and the exponential of that value as a string |
||||
- `vec_function`, returns a tuple with the original value and the logarithm of each value |
||||
|
||||
The objective is to now how ownership works with different types. |
||||
|
||||
### Notions |
||||
|
||||
- https://doc.rust-lang.org/rust-by-example/scope/move.html |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
pub fn nbr_function(c: u32) -> (u32, f64, f64) { |
||||
} |
||||
|
||||
pub fn str_function(a: String) -> (String, String) { |
||||
} |
||||
|
||||
pub fn vec_function(b: Vec<u32>) -> (Vec<u32>, Vec<f64>) { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```rust |
||||
fn main() { |
||||
let a = String::from("1 2 4 5 6"); |
||||
let b = vec![1, 2, 4, 5]; |
||||
let c: u32 = 0; |
||||
|
||||
println!("{:?}", nbr_function(c)); |
||||
// output: (12, 162754.79141900392, 2.4849066497880004) |
||||
println!("{:?}", vec_function(b)); |
||||
// output: ([1, 2, 4], [0.0, 0.6931471805599453, 1.3862943611198906]) |
||||
println!("{:?}", str_function(a)); |
||||
// output: ("1 2 4", "2.718281828459045 7.38905609893065 54.598150033144236") |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
(0, 1.0, inf) |
||||
([1, 2, 4, 5], [0.0, 0.6931471805599453, 1.3862943611198906, 1.6094379124341003]) |
||||
("1 2 4 5 6", "2.718281828459045 7.38905609893065 54.598150033144236 148.4131591025766 403.4287934927351") |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue