mirror of https://github.com/01-edu/public.git
Browse Source
* docs: adding subjects for quest1 of the java piscine * style: fix folders naming * style: fix heading * style: fix format error * docs(CleanExtract Exercice): Highlight the s variable to avoid understanding mistakespull/2048/head
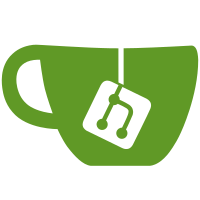
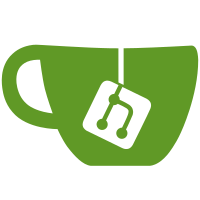
12 changed files with 641 additions and 0 deletions
@ -0,0 +1,63 @@
|
||||
## Chifoumi |
||||
|
||||
### Prerequisite |
||||
|
||||
Create a file `ChifoumiAction.java` and paste the following content : |
||||
|
||||
```java |
||||
public enum ChifoumiAction { |
||||
ROCK, PAPER, SCISSOR |
||||
} |
||||
``` |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `Chifoumi.java`. |
||||
|
||||
Write a function `getActionBeatenBy` that returns the action beaten by the action in parameter : |
||||
|
||||
- `ROCK` will return `SCISSOR` |
||||
- `PAPER` will return `ROCK` |
||||
- `SCISSOR` will return `PAPER` |
||||
|
||||
Use the `switch` operator. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class Chifoumi { |
||||
public static ChifoumiAction getActionBeatenBy(ChifoumiAction chifoumiAction) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(Chifoumi.getActionBeatenBy(ChifoumiAction.ROCK)); |
||||
System.out.println(Chifoumi.getActionBeatenBy(ChifoumiAction.PAPER)); |
||||
System.out.println(Chifoumi.getActionBeatenBy(ChifoumiAction.SCISSOR)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
SCISSOR |
||||
ROCK |
||||
PAPER |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Switch statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/switch.html) |
||||
[Enum](https://docs.oracle.com/javase/tutorial/java/javaOO/enum.html) |
@ -0,0 +1,51 @@
|
||||
## CleanExtract |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `CleanExtract.java`. |
||||
|
||||
You are given a single string **S** consisting of several substrings separated by the '|' character. Unfortunately, some of these substrings contain unnecessary characters that need to be removed in order to form a well-structured output string. |
||||
|
||||
In particular, for each substring sub in **S**, you need to extract the portion of the string that is between the first '.' and the last '.' characters, and remove any leading or trailing white space from the extracted string. These extracted substrings should then be concatenated together using the ' ' character as a separator to form the output string. |
||||
|
||||
Write a function `CleanExtract` to solve this problem. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class CleanExtract { |
||||
public static String extract(String s) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(CleanExtract.extract("The|. quick brown. | what do you ..| .fox .|. Jumps over the lazy dog. .")); |
||||
System.out.println(CleanExtract.extract(" | Who am .I | .love coding, | |. Coding is fun . | ... ")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
The quick brown fox Jumps over the lazy dog. |
||||
I love coding, Coding is fun. |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
||||
[Array](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/arrays.html) |
||||
[Conditional statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/if.html) |
||||
[Loop statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/for.html) |
@ -0,0 +1,51 @@
|
||||
## ComputeArray |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `ComputeArray.java`. |
||||
|
||||
Write a function `computeArray` that returns an array computed using the array of integer as parameter as followed : |
||||
|
||||
- if the item is a multiple of 3, the item is multiplied by 5 |
||||
- if the item is a multiple of 3 + 1 (e.g. 1, 4, 7, ...), the item is incremented by 7 |
||||
- if the item is a multiple of 3 + 2 (e.g. 2, 5, 8, ...), the item stay as it is. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class ComputeArray { |
||||
public static int[] computeArray(int[] array) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
int[] array = ComputeArray.computeArray(new int[]{9, 13, 8, 23, 1, 0, 89}); |
||||
for (int i : array) { |
||||
System.out.print(i + " "); |
||||
} |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
45 20 8 23 8 0 89 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Array](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/arrays.html) |
||||
[Conditional statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/if.html) |
||||
[Loop statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/for.html) |
@ -0,0 +1,58 @@
|
||||
## FloatOperations |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `FloatOperation.java`. |
||||
|
||||
Write a function `divideTwoFloats` that returns the float division of two floats passed as parameter. |
||||
Write a function `addTwoFloats` that returns the sum of two floats passed as parameter. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class FloatOperation { |
||||
public static float addTwoFloats(float a, float b) { |
||||
// your code here |
||||
} |
||||
public static float divideTwoFloats(float a, float b) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println("Add"); |
||||
System.out.println(FloatOperation.addTwoFloats(1.0f, 2.5f)); |
||||
System.out.println(FloatOperation.addTwoFloats(15.18f, 68.28347f)); |
||||
|
||||
System.out.println("Divide"); |
||||
System.out.println(FloatOperation.divideTwoFloats(7.0f, 2.0f)); |
||||
System.out.println(FloatOperation.divideTwoFloats(5.6f, 6.9f)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Add |
||||
3.5 |
||||
83.46347 |
||||
Divide |
||||
3.5 |
||||
0.8115942 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Primitive Links](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html) |
||||
[Operations](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op1.html) |
@ -0,0 +1,62 @@
|
||||
## HelloWorld |
||||
|
||||
### Setup |
||||
|
||||
Install the JDK of Java 17 [here](https://www.oracle.com/java/technologies/javase/jdk17-archive-downloads.html) |
||||
|
||||
Create dedicated folder to create your files for this exercise. |
||||
|
||||
If you want to test your code, you need to compile your code : |
||||
|
||||
```shell |
||||
javac *.java -d build |
||||
``` |
||||
|
||||
Then run the following command : |
||||
|
||||
```shell |
||||
java -cp build ExerciseRunner |
||||
``` |
||||
|
||||
to get the output of your function in your console. |
||||
|
||||
To edit your code, you can use any IDE or text editor, though IDEA IntelliJ or JetBrains are specially dedicated and recommended. |
||||
|
||||
On Gitea, create a repository named `((ROOT))`, and push every java files on the root folder. |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `HelloWorld.java`. |
||||
|
||||
Write a function `helloworld` that return the string 'Hello World !'. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class HelloWorld { |
||||
public static String helloWorld() { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(HelloWorld.helloWorld()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Hello World ! |
||||
$ |
||||
``` |
@ -0,0 +1,80 @@
|
||||
## IntOperations |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `IntOperation.java`. |
||||
|
||||
Write a function `addTwoIntegers` that returns the sum of two integers passed as parameter. |
||||
Write a function `substractTwoIntegers` that returns subtraction of two integers passed as parameter. |
||||
Write a function `multiplyTwoIntegers` that returns the multiplication of two integers passed as parameter. |
||||
Write a function `divideTwoIntegers` that returns the Euclidian division of two integers passed as parameter. We ignore the divide by 0 case. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class IntOperation { |
||||
public static int addTwoIntegers(int a, int b) { |
||||
// your code here |
||||
} |
||||
public static int subtractTwoIntegers(int a, int b) { |
||||
// your code here |
||||
} |
||||
public static int multiplyTwoIntegers(int a, int b) { |
||||
// your code here |
||||
} |
||||
public static int divideTwoIntegers(int a, int b) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println("Add"); |
||||
System.out.println(IntOperation.addTwoIntegers(1, 2)); |
||||
System.out.println(IntOperation.addTwoIntegers(15, 68)); |
||||
|
||||
System.out.println("Subtract"); |
||||
System.out.println(IntOperation.subtractTwoIntegers(4, 1)); |
||||
System.out.println(IntOperation.subtractTwoIntegers(10, 23)); |
||||
|
||||
System.out.println("Multiply"); |
||||
System.out.println(IntOperation.multiplyTwoIntegers(3, 6)); |
||||
System.out.println(IntOperation.multiplyTwoIntegers(12, 11)); |
||||
|
||||
System.out.println("Divide"); |
||||
System.out.println(IntOperation.divideTwoIntegers(8, 2)); |
||||
System.out.println(IntOperation.divideTwoIntegers(13, 4)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Add |
||||
3 |
||||
83 |
||||
Subtract |
||||
3 |
||||
-13 |
||||
Multiply |
||||
18 |
||||
132 |
||||
Divide |
||||
4 |
||||
3 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Primitive Links](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html) |
||||
[Operations](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op1.html) |
@ -0,0 +1,49 @@
|
||||
## IsEven |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `IsEven.java`. |
||||
|
||||
Write a function `isEven` that returns true if the int passed in parameter is even, otherwise returns false. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class IsEven { |
||||
public static boolean isEven(int a) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
System.out.println(IsEven.isEven(2)); |
||||
System.out.println(IsEven.isEven(26)); |
||||
System.out.println(IsEven.isEven(57)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Primitive Links](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html) |
||||
[Logic operations](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op2.html) |
||||
[Operations](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op1.html) |
@ -0,0 +1,46 @@
|
||||
## Palindrome |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `Palindrome.java`. |
||||
|
||||
Write a function `isPalindrome` that returns true if the String as parameter is a palindrome, i.e. can be read in both direction (e.g. 'kayak'). |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class Palindrome { |
||||
public static boolean isPalindrome(String s) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(Palindrome.isPalindrome("ressasser")); |
||||
System.out.println(Palindrome.isPalindrome("Bonjour")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
||||
[Conditional statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/if.html) |
||||
[Loop statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/for.html) |
@ -0,0 +1,44 @@
|
||||
## StringConcat |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StringConcat.java`. |
||||
|
||||
Write a function `concat` that returns the concatenation of the two strings passed as parameters. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class StringConcat { |
||||
public static String concat(String s1, String s2) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(StringConcat.concat("Hello ", "étudiant !")); |
||||
System.out.println(StringConcat.concat("", "Hello World !")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Hello étudiant ! |
||||
Hello World ! |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
@ -0,0 +1,47 @@
|
||||
## StringContain |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StringContain.java`. |
||||
|
||||
Write a function `isStringContainedIn` that returns true if the first String as parameter is contained in the second String as parameter. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class StringContain { |
||||
public static boolean isStringContainedIn(String subString, String s) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(StringContain.isStringContainedIn("Hell", "Highway to Hell")); |
||||
System.out.println(StringContain.isStringContainedIn("Hell", "Hello World !")); |
||||
System.out.println(StringContain.isStringContainedIn("Bonjour", "hello World !")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
||||
[Conditional statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/if.html) |
@ -0,0 +1,44 @@
|
||||
## StringLength |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StringLength.java`. |
||||
|
||||
Write a function `getStringLength` that returns the length of the string passed as parameter. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class StringLength { |
||||
public static int getStringLength(String s) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(StringLength.getStringLength("Hello World !")); |
||||
System.out.println(StringLength.getStringLength("")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
13 |
||||
0 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
@ -0,0 +1,46 @@
|
||||
## StringReplace |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StringReplace.java`. |
||||
|
||||
Write a function called `StringReplace` that takes in three parameters: `original_string s`, `target`, and `replacement`. The function should replace all occurrences of target in `original_string s` with `replacement` and return the modified string. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class StringReplace { |
||||
public static String replace(String s, target, replacement) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(StringContain.replace("javatpoint is a very good website", 'a', 'e')); |
||||
System.out.println(StringContain.replace("my name is khan my name is java", "is","was")); |
||||
System.out.println(StringContain.replace("hey i'am java", "I'am","was")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
jevetpoint is e very good website |
||||
my name was khan my name was java |
||||
hey i'am java |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
Loading…
Reference in new issue