mirror of https://github.com/01-edu/public.git
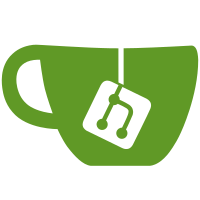
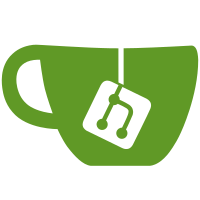
2 changed files with 129 additions and 0 deletions
@ -0,0 +1,69 @@
|
||||
## Let's Play |
||||
|
||||
### Objectives |
||||
|
||||
You will be developing a basic CRUD (Create, Read, Update, Delete) API using Spring Boot with MongoDB, and it should adhere to RESTful principles. The application will contain user management and product management functionalities. |
||||
|
||||
### Instructions |
||||
|
||||
#### 1. Database Design |
||||
|
||||
```mermaid |
||||
classDiagram |
||||
User "1" -- "n" Product : Owns |
||||
User : +String id |
||||
User : +String name |
||||
User : +String email |
||||
User : +String password |
||||
User : +String role |
||||
Product : +String id |
||||
Product : +String name |
||||
Product : +String description |
||||
Product : +Double price |
||||
Product : +String userId |
||||
``` |
||||
|
||||
#### 2. API Development |
||||
|
||||
You should provide a set of RESTful APIs to perform CRUD operations on both Users and Products. The APIs should be designed according to the REST standard. The "GET Products" API should be accessible without authentication. |
||||
|
||||
#### 3. Authentication & Authorization |
||||
|
||||
Implement a token-based authentication system. Only authenticated users can access the APIs. The users can have different roles (admin or user), and the API access should be controlled based on the user roles. |
||||
|
||||
> đź’ˇ Spring Security |
||||
|
||||
#### 4. Error Handling |
||||
|
||||
The API should not return any 5XX errors. You should handle any possible exceptions and return appropriate HTTP response codes and messages. |
||||
|
||||
#### 5. Security Measures |
||||
|
||||
Implement the following security measures: |
||||
|
||||
- Hash and salt passwords before storing them in the database. |
||||
- Validate inputs to prevent MongoDB injection attacks. |
||||
- Protect sensitive user information. Don't return passwords or other sensitive information in your API responses. |
||||
- Use HTTPS to protect data in transit. |
||||
|
||||
### Bonus |
||||
|
||||
As an additional challenge, you could consider implementing the following features. Note that these are not required for the completion of the project but would provide additional learning opportunities: |
||||
|
||||
- **Set appropriate CORS policies:** Implement Cross-Origin Resource Sharing (CORS) policies to manage the security of your application when it is accessed from different domains. |
||||
- **Implement rate limiting to prevent brute force attacks:** Use rate limiting to restrict the number of API requests a client can make in a given time. This can help prevent attacks and misuse of your application. |
||||
|
||||
### Testing |
||||
|
||||
Your project will be extensively tested for the following aspects: |
||||
|
||||
- Correctness of the APIs. |
||||
- Proper implementation of authentication and authorization. |
||||
- The absence of 5XX errors. |
||||
- Implementation of the above-mentioned security measures. |
||||
|
||||
In order for auditors to test your program, you will have to run your project using a code editor or provide a script to run it. |
||||
|
||||
### Resources |
||||
[Spring initializer](https://start.spring.io/) |
||||
[Rest Documentation](https://docs.github.com/en/rest?apiVersion=2022-11-28) |
@ -0,0 +1,60 @@
|
||||
#### Functional |
||||
|
||||
##### Download the project and start it according to the instructions provided by the student. Try accessing the API endpoints with Postman or similar API testing tool. |
||||
|
||||
###### Does the application run and respond to API requests? |
||||
|
||||
##### Check the implementation of CRUD operations for Users and Products. |
||||
|
||||
###### Are all CRUD operations correctly implemented for Users and Products? |
||||
|
||||
##### Try authenticating with a user. Then, try performing operations on Users and Products. |
||||
|
||||
###### Does the authentication work correctly? Do operations respect the user roles? |
||||
|
||||
##### Check the handling of exceptions. Try to create scenarios where the application could throw exceptions (like trying to update non-existing user). |
||||
|
||||
###### Does the application handle the exceptions and return appropriate HTTP response codes and messages? |
||||
|
||||
##### Check if the "GET Products" API is accessible without authentication. |
||||
|
||||
###### Can you access the "GET Products" API without being authenticated? |
||||
|
||||
#### Security |
||||
|
||||
##### Check the implementation of the mentioned security measures (hashed passwords, input validation, protection of sensitive information, HTTPS). |
||||
|
||||
###### Are all the mentioned security measures implemented? |
||||
|
||||
#### Code Quality and Standards |
||||
|
||||
##### Check the use of appropriate annotations in the data classes. |
||||
|
||||
###### Are the annotations @Document, @Id, and @Field used correctly in the data classes? |
||||
|
||||
##### Check the use of appropriate annotations in the controller classes. |
||||
|
||||
###### Are the annotations @RestController, @RequestMapping, @GetMapping, @PostMapping, @PutMapping, and @DeleteMapping used correctly in the controller classes? |
||||
|
||||
##### Check the use of the @Autowired annotation for dependency injection. |
||||
|
||||
###### Is the @Autowired annotation used correctly for dependency injection? |
||||
|
||||
##### Check the use of appropriate annotations for authentication and role-based access control. |
||||
|
||||
###### Are the annotations @EnableWebSecurity, @EnableGlobalMethodSecurity, @Secured, @PermitAll and @PreAuthorize used correctly? |
||||
|
||||
##### Check the use of validation annotations in the data classes. |
||||
|
||||
###### Are validation annotations like @NotNull, @Size, @Email, etc., used to validate input data? |
||||
|
||||
#### Bonus |
||||
|
||||
##### Check if CORS policies are set appropriately. |
||||
|
||||
###### +Are the CORS policies set appropriately? |
||||
|
||||
##### Check if rate limiting is implemented to prevent brute force attacks. |
||||
|
||||
###### +Is rate limiting implemented? |
||||
|
Loading…
Reference in new issue