mirror of https://github.com/01-edu/public.git
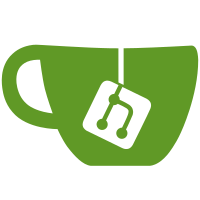
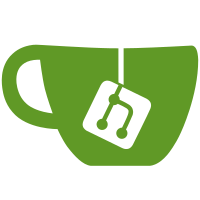
10 changed files with 530 additions and 0 deletions
@ -0,0 +1,56 @@
|
||||
## StarConstructors |
||||
|
||||
### Instructions |
||||
|
||||
We will now add two constructors : |
||||
|
||||
* The default one, with no parameter which inits the properties to the following values : |
||||
* `x` : `0.0` |
||||
* `y` : `0.0` |
||||
* `z` : `0.0` |
||||
* `name` : `"Soleil"` |
||||
* The second one take four parameters and sets the values of `name`, `x`, `y` and `z`. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
CelestialObject defaultStar = new CelestialObject(); |
||||
System.out.println(defaultStar.x); |
||||
System.out.println(defaultStar.y); |
||||
System.out.println(defaultStar.z); |
||||
System.out.println(defaultStar.name); |
||||
|
||||
CelestialObject earth = new CelestialObject("Terre", 0.43, 0.98, 1.43); |
||||
System.out.println(earth.x); |
||||
System.out.println(earth.y); |
||||
System.out.println(earth.z); |
||||
System.out.println(earth.name); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
0.0 |
||||
0.0 |
||||
0.0 |
||||
Soleil |
||||
0.43 |
||||
0.98 |
||||
1.43 |
||||
Terre |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Class](https://docs.oracle.com/javase/tutorial/java/javaOO/classdecl.html) |
||||
[Property](https://docs.oracle.com/javase/tutorial/java/javaOO/variables.html) |
@ -0,0 +1,57 @@
|
||||
## StarGalaxy |
||||
|
||||
### Instructions |
||||
|
||||
Create a new class `Galaxy` in a file named `Galaxy.java`. |
||||
|
||||
It has one private property : `celestialObjects` of type `List<CelestialObject>`. |
||||
|
||||
It has one constructor with no parameters, which instantiate an empty list in the `celestialObjects` property. |
||||
|
||||
We add a getter for the `celestialObjects` property (`getCelestialObjects`). |
||||
|
||||
We create a new method `addCelestialObject` with a `CelestialObject` argument. This method adds the object in parameter to the `celestialObjects` list. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Galaxy galaxy = new Galaxy(); |
||||
CelestialObject lune = new CelestialObject("Lune", -123.12, 392.238, 32.31); |
||||
Star betelgeuse = new Star("Betelgeuse", 128.23, -12.82, 32.328, 1289.3); |
||||
Planet naboo = new Planet("Naboo", 17.4389, 8349.1, 8943.92, betelgeuse); |
||||
|
||||
galaxy.addCelestialObject(lune); |
||||
galaxy.addCelestialObject(betelgeuse); |
||||
galaxy.addCelestialObject(naboo); |
||||
|
||||
List<CelestialObject> celestialObjects = galaxy.getCelestialObjects(); |
||||
|
||||
for (CelestialObject celestialObject : celestialObjects) { |
||||
System.out.println(celestialObject.toString()); |
||||
} |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Lune is positioned at (-123,120, 392,238, 32,310) |
||||
Betelgeuse shines at the 1289.300 magnitude |
||||
Naboo circles around Betelgeuse at the 12220.902 AU |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Polymorphism](https://docs.oracle.com/javase/tutorial/java/IandI/polymorphism.html) |
||||
|
@ -0,0 +1,59 @@
|
||||
## StarGetters |
||||
|
||||
### Instructions |
||||
|
||||
Now, we will update the accessibility of the properties. |
||||
|
||||
In order to still have access to them, we need to implement getters and setters for each property : |
||||
|
||||
- `getX` and `setX` for the `x` property |
||||
- `getY` and `setY` for the `y` property |
||||
- `getZ` and `setZ` for the `z` property |
||||
- `getName` and `setName` for the `name` property |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
CelestialObject defaultStar = new CelestialObject(); |
||||
System.out.println(defaultStar.getX()); |
||||
System.out.println(defaultStar.getY()); |
||||
System.out.println(defaultStar.getZ()); |
||||
System.out.println(defaultStar.getName()); |
||||
|
||||
defaultStar.setName("Terre"); |
||||
defaultStar.setX(0.43); |
||||
defaultStar.setY(0.98); |
||||
defaultStar.setZ(1.43); |
||||
System.out.println(defaultStar.getX()); |
||||
System.out.println(defaultStar.getY()); |
||||
System.out.println(defaultStar.getZ()); |
||||
System.out.println(defaultStar.getName()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
0.0 |
||||
0.0 |
||||
0.0 |
||||
Soleil |
||||
0.43 |
||||
0.98 |
||||
1.43 |
||||
Terre |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Method](https://docs.oracle.com/javase/tutorial/java/javaOO/methods.html) |
||||
[Property](https://docs.oracle.com/javase/tutorial/java/javaOO/variables.html) |
@ -0,0 +1,43 @@
|
||||
## StarInheritance |
||||
|
||||
### Instructions |
||||
|
||||
Create a new class `Star` in a file named `Star.java`. |
||||
|
||||
This class inherits from `CelestialObject`. We add a private double property `magnitude`, with public getter and setter. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Star star = new Star(); |
||||
|
||||
System.out.println(star.getName()); |
||||
System.out.println(star.getX()); |
||||
System.out.println(star.getY()); |
||||
System.out.println(star.getZ()); |
||||
System.out.println(star.getMagnitude()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Soleil |
||||
0.0 |
||||
0.0 |
||||
0.0 |
||||
0.0 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Subclasses](https://docs.oracle.com/javase/tutorial/java/IandI/subclasses.html) |
@ -0,0 +1,59 @@
|
||||
## StarMass |
||||
|
||||
### Instructions |
||||
|
||||
For this last exercise, let's compute the mass of all objects in a galaxy, according their type. |
||||
|
||||
Firstly, let's add a mass property (it will be an integer) to all objects. I let you guess in which class to put it ;) |
||||
You need to add the getter and setter for this property too. You will need to add a mass argument to all the constructors. |
||||
|
||||
In the `Galaxy` class, we will add a new method `computeMassRepartition` which returns a Map. This map will have for key the Strings `Star`, `Planet` or `Other`. The values will be the sum of the mass of the object by their type. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Galaxy galaxy = new Galaxy(); |
||||
CelestialObject charron = new CelestialObject("Charron", -123.12, 392.238, 32.31, 157); |
||||
CelestialObject lune = new CelestialObject("Lune", 3928.32, 327.239, -12.92, 3987); |
||||
Star betelgeuse = new Star("Betelgeuse", 128.23, -12.82, 32.328, 1289.3, 538595); |
||||
Star altair = new Star("Betelgeuse", 43894.34, -324.43, 9438.23, 123.54, 137273); |
||||
Star bellatrix = new Star("Bellatrix", 584.34, 2103.32, -102.43, 413.2, 5483724); |
||||
Planet naboo = new Planet("Naboo", 17.4389, 8349.1, 8943.92, betelgeuse, 32454); |
||||
Planet tatooine = new Planet("Tatooine", 17.4389, 8349.1, 8943.92, betelgeuse, 2345); |
||||
Planet mercure = new Planet("Mercure", 17.4389, 8349.1, 8943.92, altair, 19438); |
||||
Planet venus = new Planet("Venus", 17.4389, 8349.1, 8943.92, altair, 9283); |
||||
Planet mars = new Planet("Mars", 17.4389, 8349.1, 8943.92, altair, 2183); |
||||
|
||||
galaxy.addCelestialObject(lune); |
||||
galaxy.addCelestialObject(charron); |
||||
galaxy.addCelestialObject(mars); |
||||
galaxy.addCelestialObject(mercure); |
||||
galaxy.addCelestialObject(tatooine); |
||||
galaxy.addCelestialObject(altair); |
||||
galaxy.addCelestialObject(venus); |
||||
galaxy.addCelestialObject(betelgeuse); |
||||
galaxy.addCelestialObject(naboo); |
||||
galaxy.addCelestialObject(bellatrix); |
||||
|
||||
System.out.println(galaxy.computeMassRepartition().toString()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
{Star=6159592, Planet=65703, Other=4144} |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[InstanceOf](https://www.baeldung.com/java-instanceof) |
@ -0,0 +1,51 @@
|
||||
## StarOverride |
||||
|
||||
### Instructions |
||||
|
||||
In the `Star` class, let's add a new constructor with the following arguments : |
||||
* the `name` |
||||
* the position `x` |
||||
* the position `y` |
||||
* the position `z` |
||||
* the `magnitude` |
||||
It calls the constructor of the superclass `CelestialObject`. |
||||
|
||||
We will override the `hashCode` and `equals`, using the `magnitude` property. |
||||
|
||||
Finally, we rewrite the `toString` method. The returned String must have the following format : `<name> shines at the <magnitude> magnitude` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Star star = new Star(); |
||||
Star star2 = new Star(); |
||||
Star proxima = new Star("Proxima", 18.389, 832.32, 218, 0.4); |
||||
|
||||
System.out.println(star.toString()); |
||||
System.out.println(proxima.toString()); |
||||
System.out.println(star.equals(star2)); |
||||
System.out.println(star.equals(proxima)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Soleil shines at the 0.000 magnitude |
||||
Proxima shines at the 0.400 magnitude |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Override](https://docs.oracle.com/javase/tutorial/java/IandI/override.html) |
@ -0,0 +1,55 @@
|
||||
## StarPlanet |
||||
|
||||
### Instructions |
||||
|
||||
Create a new class `Planet` in a file named `Planet.java`. |
||||
|
||||
This class inherits `CelestialObject`. |
||||
It has one private property : `centerStar` of type `Star`. |
||||
|
||||
There are two constructors : |
||||
|
||||
- one with no parameters, which calls the superclass constructor with no parameters. In this case, the `centerStar` property is initialized with the default Star constructor. |
||||
- one with many parameters : |
||||
- `name` |
||||
- `x` |
||||
- `y` |
||||
- `z` |
||||
- `centerStar` |
||||
which calls the superclass constructor with full parameters. |
||||
|
||||
We add a getter and a setter for the centerStar property (`getCenterStar` and `setCenterStar`). |
||||
|
||||
We will override the `hashCode` and `equals`, using the `centerStar` property. |
||||
|
||||
Finally, we rewrite the `toString` method. The returned String must have the following format : `<name> circles around <centerStar.name> at the <distanceWithCenterStar> AU`. |
||||
The `distanceWithCenterStar` is computed using the `distanceBetween` method with the planet and its center star.## |
||||
|
||||
# Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Planet earth = new Planet(); |
||||
Planet naboo = new Planet("Naboo", 17.4389, 8349.1, 8943.92, new Star("Betelgeuse", 128.23, -12.82, 32.328, 1289.3)); |
||||
|
||||
System.out.println(naboo.toString()); |
||||
System.out.println(earth.toString()); |
||||
System.out.println(naboo.getCenterStar().toString()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Naboo circles around Betelgeuse at the 12220.902 AU |
||||
Soleil circles around Soleil at the 0.000 AU |
||||
Betelgeuse shines at the 1289.300 magnitude |
||||
$ |
||||
``` |
@ -0,0 +1,50 @@
|
||||
## StarProperties |
||||
|
||||
### Instructions |
||||
|
||||
In the following quest, we will work with the same files and classes. You should keep them from one exercise to the following. |
||||
|
||||
Create a file `CelestialObject.java`. |
||||
|
||||
Create a public class named `CelestialObject`. |
||||
The class must contains four properties : |
||||
* x (double) |
||||
* y (double) |
||||
* z (double) |
||||
* name (String) |
||||
|
||||
The (x, y, z) properties are the coordinates of the object. |
||||
|
||||
For the moment, you should declare the properties as public. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
CelestialObject celestialObject = new CelestialObject(); |
||||
System.out.println(celestialObject.x); |
||||
System.out.println(celestialObject.y); |
||||
System.out.println(celestialObject.z); |
||||
System.out.println(celestialObject.name); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
0.0 |
||||
0.0 |
||||
0.0 |
||||
null |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[Class](https://docs.oracle.com/javase/tutorial/java/javaOO/classdecl.html) |
||||
[Property](https://docs.oracle.com/javase/tutorial/java/javaOO/variables.html) |
@ -0,0 +1,48 @@
|
||||
## StarStatic |
||||
|
||||
### Instructions |
||||
|
||||
Let's add some computation. |
||||
|
||||
Our objective is to compute the distance between celestial objects. As you may have guessed, the x, y and z values are the coordinates of the object. Their unit is AU (Astronomical Unit) which is 150 000 000 km. |
||||
|
||||
We will add two `static` and `public` method : |
||||
|
||||
- `getDistanceBetween` which takes two CelestialObject as parameters and returns a double corresponding to the distance between the two objects. |
||||
- `getDistanceBetweenInKm` which takes two CelestialObject as parameters and returns a double corresponding to the distance in km between the two objects. |
||||
|
||||
We add a public constant double property, named `KM_IN_ONE_AU` with the value of 150 000 000. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
CelestialObject defaultStar = new CelestialObject(); |
||||
CelestialObject earth = new CelestialObject("Terre", 1.0, 2.0, 2.0); |
||||
|
||||
System.out.println(CelestialObject.getDistanceBetween(defaultStar, earth)); |
||||
System.out.println(CelestialObject.getDistanceBetweenInKm(defaultStar, earth)); |
||||
System.out.println(CelestialObject.KM_IN_ONE_AU); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
3.0 |
||||
4.5E8 |
||||
1.5E8 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Class Member / Static](https://docs.oracle.com/javase/tutorial/java/javaOO/classvars.html) |
||||
[Math](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/Math.html) |
@ -0,0 +1,52 @@
|
||||
## StarUtils |
||||
|
||||
### Instructions |
||||
|
||||
Now, some utils method for our class. |
||||
|
||||
First, the `toString` method which will return a literal version of our class. The format is the following : `<name> is positioned at (<x>, <y>, <z>)`. The printed double will be with 3 decimals. |
||||
|
||||
Then, the `equals(Object object)` method which will return trus if all properties of the object as parameters are equals to the current object. |
||||
|
||||
As we have rewritten the `equals` method, we need to rewrite the `hashCode` method. This method returns an integer. If two objects are equals (using the `equals` method), then the results of their hashCode method should be equals. |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
CelestialObject celestialObject = new CelestialObject(); |
||||
CelestialObject earth = new CelestialObject("Terre", 1.0, 2.0, 2.0); |
||||
CelestialObject earth1 = new CelestialObject("Terre", 1.0, 2.0, 2.0); |
||||
|
||||
System.out.println(earth.toString()); |
||||
System.out.println(earth.equals(earth1)); |
||||
System.out.println(earth.equals(celestialObject)); |
||||
|
||||
System.out.println(earth.hashCode()); |
||||
System.out.println(celestialObject.hashCode()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Terre is positioned at (1.000, 2.000, 2.000) |
||||
true |
||||
false |
||||
2129490293 |
||||
-1811995559 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Equals](<https://docs.oracle.com/javase/10/docs/api/java/lang/Object.html#equals(java.lang.Object)>) |
||||
[HashCode](<https://docs.oracle.com/javase/10/docs/api/java/lang/Object.html#hashCode()>) |
||||
[ToString](<https://docs.oracle.com/javase/10/docs/api/java/lang/Object.html#toString()>) |
Loading…
Reference in new issue