mirror of https://github.com/01-edu/public.git
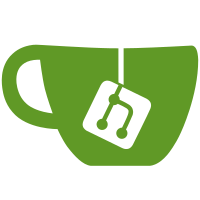
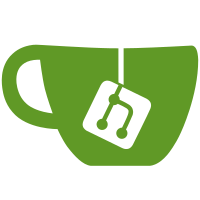
75 changed files with 2616 additions and 0 deletions
@ -0,0 +1,42 @@
|
||||
## abort |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the the value in the middle of 5 five arguments. |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Abort(a, b, c, d, e int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
middle := student.Abort(2, 3, 8, 5, 7) |
||||
fmt.Println(middle) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/abort$ go build |
||||
student@ubuntu:~/student/abort$ ./abort |
||||
5 |
||||
student@ubuntu:~/student/abort$ |
||||
``` |
@ -0,0 +1,42 @@
|
||||
## activebits |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, ActiveBitsthat, that returns the number of active bits (bits with the value 1) in the binary representation of an integer number. |
||||
|
||||
The function must have the next signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ActiveBits(n int) uint { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
nbits := student.ActiveBits(7) |
||||
fmt.Println(nbits) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/activebits$ go build |
||||
student@ubuntu:~/student/activebits$ ./activebits |
||||
10 |
||||
student@ubuntu:~/student/activebits$ |
||||
``` |
@ -0,0 +1,20 @@
|
||||
## addprimesum |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a positive integer as argument and displays the sum of all prime numbers inferior or equal to it followed by a newline. |
||||
|
||||
- If the number of arguments is not 1, or if the argument is not a positive number, the program displays 0 followed by a newline. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test 5 |
||||
10 |
||||
student@ubuntu:~/piscine/test$ ./test 7 |
||||
17 |
||||
student@ubuntu:~/piscine/test$ ./test 57 |
||||
0 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1 @@
|
||||
## advancedsortwordtab |
@ -0,0 +1,29 @@
|
||||
## alphamirror |
||||
|
||||
### Instructions |
||||
|
||||
Write a program called alphamirror that takes a string as argument and displays this string |
||||
after replacing each alphabetical character with the opposite alphabetical |
||||
character. |
||||
|
||||
The case of the letter stays the same, for example : |
||||
|
||||
'a' becomes 'z', 'Z' becomes 'A' |
||||
'd' becomes 'w', 'M' becomes 'N' |
||||
|
||||
The final result will be followed by a newline. |
||||
|
||||
If the number of arguments is not 1, the program will display only a newline. |
||||
|
||||
Example of output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/alphamirror$ go build |
||||
student@ubuntu:~/student/alphamirror$ ./alphamirror "abc" |
||||
zyx |
||||
student@ubuntu:~/student/alphamirror$ ./alphamirror "My horse is Amazing." | cat -e |
||||
Nb slihv rh Znzarmt.$ |
||||
student@ubuntu:~/student/alphamirror$ ./alphamirror | cat -e |
||||
$ |
||||
student@ubuntu:~/student/alphamirror$ |
||||
``` |
@ -0,0 +1,46 @@
|
||||
## Boolean |
||||
|
||||
### Instructions |
||||
|
||||
Create a `.go` file and copy the code below into our file |
||||
|
||||
- The main task is to return a working program. |
||||
|
||||
```go |
||||
func printStr(str string) { |
||||
arrayStr := []rune(str) |
||||
|
||||
for i := 0; i < len(arrayStr); i++ { |
||||
z01.PrintRune(arrayStr[i]) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
func isEven(nbr int) boolean { |
||||
if even(nbr) == 1 { |
||||
return yes |
||||
} else { |
||||
return no |
||||
} |
||||
} |
||||
|
||||
func main() { |
||||
if isEven(lengthOfArg) == 1 { |
||||
printStr(EvenMsg) |
||||
} else { |
||||
printStr(OddMsg) |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
I have an even number of arguments |
||||
``` |
||||
|
||||
### Or |
||||
|
||||
```console |
||||
I have an odd number of arguments |
||||
``` |
@ -0,0 +1,36 @@
|
||||
## brackets |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes an undefined number of strings in arguments. For each |
||||
argument, the program prints on the standard output "OK" followed by a newline |
||||
if the expression is correctly bracketed, otherwise it prints "Error" followed by |
||||
a newline. |
||||
|
||||
|
||||
Symbols considered as `brackets` are brackets `(` and `)`, square brackets `[` |
||||
and `]`and braces `{` and `}`. Every other symbols are simply ignored. |
||||
|
||||
An opening bracket must always be closed by the good closing bracket in the |
||||
correct order. A string which do not contains any bracket is considered as a |
||||
correctly bracketed string. |
||||
|
||||
If there is no arguments, the program must print only a newline. |
||||
|
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/brackets$ go build |
||||
student@ubuntu:~/student/brackets$ ./brackets '(johndoe)' | cat -e |
||||
OK$ |
||||
student@ubuntu:~/student/brackets$ ./brackets '([)]' | cat -e |
||||
Error$ |
||||
student@ubuntu:~/student/brackets$ ./brackets '' '{[(0 + 0)(1 + 1)](3*(-1)){()}}' | cat -e |
||||
OK$ |
||||
OK$ |
||||
student@ubuntu:~/student/brackets$ ./brackets | cat -e |
||||
$ |
||||
student@ubuntu:~/student/brackets$ |
||||
|
||||
``` |
@ -0,0 +1,37 @@
|
||||
## brainfuck |
||||
|
||||
### Instructions |
||||
|
||||
Write a Brainfuck interpreter program. |
||||
The source code will be given as first parameter. |
||||
The code will always be valid, with no more than 4096 operations. |
||||
Brainfuck is a minimalist language. It consists of an array of bytes |
||||
(in our case, let's say 2048 bytes) initialized to zero, |
||||
and a pointer to its first byte. |
||||
|
||||
Every operator consists of a single character : |
||||
|
||||
- '>' increment the pointer ; |
||||
- '<' decrement the pointer ; |
||||
- '+' increment the pointed byte ; |
||||
- '-' decrement the pointed byte ; |
||||
- '.' print the pointed byte on standard output ; |
||||
- '[' go to the matching ']' if the pointed byte is 0 (while start) ; |
||||
- ']' go to the matching '[' if the pointed byte is not 0 (while end). |
||||
|
||||
Any other character is a comment. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/brainfuck$ go build |
||||
student@ubuntu:~/student/brainfuck$ ./brainfuck "++++++++++[>+++++++>++++++++++>+++>+<<<<-]>++.>+.+++++++..+++.>++.<<+++++++++++++++.>.+++.------.--------.>+.>." | cat -e |
||||
Hello World!$ |
||||
student@ubuntu:~/student/brainfuck$ ./brainfuck "+++++[>++++[>++++H>+++++i<<-]>>>++\n<<<<-]>>--------.>+++++.>." | cat -e |
||||
Hi$ |
||||
student@ubuntu:~/student/brainfuck$ ./brainfuck "++++++++++[>++++++++++>++++++++++>++++++++++<<<-]>---.>--.>-.>++++++++++." | cat -e |
||||
abc$ |
||||
student@ubuntu:~/student/brainfuck$ ./brainfuck | cat -e |
||||
$ |
||||
student@ubuntu:~/student/brainfuck$ |
||||
``` |
@ -0,0 +1,48 @@
|
||||
## btreeapplybylevel |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, BTreeApplyByLevel, that applies the function given by fn to each node of the tree given by root. |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeApplyByLevel(root *TreeNode, fn interface{}) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
student.BTreeApplyByLevel(root, fmt.Println) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreeapplybylevel$ go build |
||||
student@ubuntu:~/student/btreeapplybylevel$ ./btreeapplybylevel |
||||
4 |
||||
1 |
||||
7 |
||||
5 |
||||
student@ubuntu:~/student/btreeapplybylevel$ |
||||
``` |
@ -0,0 +1,48 @@
|
||||
## btreeinsertdata |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that applies a function in order to each element in the tree |
||||
(see in order tree walks) |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeApplyInorder(root *piscine.TreeNode, f func(...interface{}) (int, error)) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine "." |
||||
) |
||||
|
||||
func main() { |
||||
root := &piscine.TreeNode{Data: "4"} |
||||
piscine.BTreeInsertData(root, "1") |
||||
piscine.BTreeInsertData(root, "7") |
||||
piscine.BTreeInsertData(root, "5") |
||||
BTreeApplyInorder(root, fmt.Println) |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/btreeinsertdata$ go build |
||||
student@ubuntu:~/piscine/btreeinsertdata$ ./btreeinsertdata |
||||
1 |
||||
4 |
||||
5 |
||||
7 |
||||
student@ubuntu:~/piscine/btreeinsertdata$ |
||||
``` |
@ -0,0 +1,47 @@
|
||||
## btreeinsertdata |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that applies a function using a postorder walk to each element in the tree |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeApplyPostorder(root *piscine.TreeNode, f func(...interface{}) (int, error)) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine "." |
||||
) |
||||
|
||||
func main() { |
||||
root := &piscine.TreeNode{Data: "4"} |
||||
piscine.BTreeInsertData(root, "1") |
||||
piscine.BTreeInsertData(root, "7") |
||||
piscine.BTreeInsertData(root, "5") |
||||
BTreeApplyPostorder(root, fmt.Println) |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/btreeinsertdata$ go build |
||||
student@ubuntu:~/piscine/btreeinsertdata$ ./btreeinsertdata |
||||
1 |
||||
5 |
||||
7 |
||||
4 |
||||
student@ubuntu:~/piscine/btreeinsertdata$ |
||||
``` |
@ -0,0 +1,47 @@
|
||||
## btreeinsertdata |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that applies a function using a preorder walk to each element in the tree |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeApplyPreorder(root *piscine.TreeNode, f func(...interface{}) (int, error)) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine "." |
||||
) |
||||
|
||||
func main() { |
||||
root := &piscine.TreeNode{Data: "4"} |
||||
piscine.BTreeInsertData(root, "1") |
||||
piscine.BTreeInsertData(root, "7") |
||||
piscine.BTreeInsertData(root, "5") |
||||
BTreeApplyPreorder(root, fmt.Println) |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/btreeinsertdata$ go build |
||||
student@ubuntu:~/piscine/btreeinsertdata$ ./btreeinsertdata |
||||
4 |
||||
1 |
||||
7 |
||||
5 |
||||
student@ubuntu:~/piscine/btreeinsertdata$ |
||||
``` |
@ -0,0 +1,61 @@
|
||||
## btreedeletenode |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, BTreeDeleteNode, that deletes 'node' from the tree given by root. |
||||
|
||||
The resulting tree should still follow the binary search tree rules. |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeDeleteNode(root, node *TreeNode) *TreeNode { |
||||
|
||||
} |
||||
|
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
node := student.BTreeSearchItem(root, "4") |
||||
fmt.Println("Before delete:") |
||||
student.BTreeApplyInorder(root, fmt.Println) |
||||
root = student.BTreeDeleteNode(root, node) |
||||
fmt.Println("After delete:") |
||||
student.BTreeApplyInorder(root, fmt.Println) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreedeletenode$ go build |
||||
student@ubuntu:~/student/btreedeletenode$ ./btreedeletenode |
||||
Before delete: |
||||
1 |
||||
4 |
||||
5 |
||||
7 |
||||
After delete: |
||||
1 |
||||
5 |
||||
7 |
||||
student@ubuntu:~/student/btreedeletenode$ |
||||
``` |
@ -0,0 +1,52 @@
|
||||
## btreeinsertdata |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that inserts new data in a binary search tree |
||||
following the properties of binary search trees. |
||||
The nodes must be defined as follows: |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
type TreeNode struct { |
||||
Left, Right, Parent *TreeNode |
||||
Data string |
||||
} |
||||
|
||||
func BTreeInsertData(root *TreeNode, data string) *TreeNode { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
root := &TreeNode{data: "4"} |
||||
BTreeInsertData(root, "1") |
||||
BTreeInsertData(root, "7") |
||||
BTreeInsertData(root, "5") |
||||
fmt.Println(root.left.data) |
||||
fmt.Println(root.data) |
||||
fmt.Println(root.right.left.data) |
||||
fmt.Println(root.right.data) |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/btreeinsertdata$ go build |
||||
student@ubuntu:~/piscine/btreeinsertdata$ ./btreeinsertdata |
||||
1 |
||||
4 |
||||
5 |
||||
7 |
||||
student@ubuntu:~/piscine/btreeinsertdata$ |
||||
``` |
@ -0,0 +1,45 @@
|
||||
## btreeisbinary |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, BTreeIsBinary, that returns true only if the tree given by root follows the binary search tree properties. |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeIsBinary(root *TreeNode) bool { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
fmt.Println(student.BTreeIsBinary(root)) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreeisbinary$ go build |
||||
student@ubuntu:~/student/btreeisbinary$ ./btreeisbinary |
||||
true |
||||
student@ubuntu:~/student/btreeisbinary$ |
||||
``` |
@ -0,0 +1,43 @@
|
||||
## btreelevelcount |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, BTreeLevelCount, that return the number of levels of the tree (height of the tree) |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeLevelCount(root *piscine.TreeNode) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
fmt.Println(BTreeLevelCount(root)) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreesearchitem$ go build |
||||
student@ubuntu:~/student/btreesearchitem$ ./btreesearchitem |
||||
3 |
||||
student@ubuntu:~/student/btreesearchitem$ |
||||
``` |
@ -0,0 +1,46 @@
|
||||
## btreemax |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, BTreeMax, that returns the node with the maximum value in the tree given by root |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeMax(root *TreeNode) *TreeNode { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
max := student.BTreeMax(root) |
||||
fmt.Println(max.Data) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreemax$ go build |
||||
student@ubuntu:~/student/btreemax$ ./btreemax |
||||
7 |
||||
student@ubuntu:~/student/btreemax$ |
||||
``` |
@ -0,0 +1,46 @@
|
||||
## btreemin |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, BTreeMin, that returns the node with the minimum value in the tree given by root |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeMin(root *TreeNode) *TreeNode { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
min := student.BTreeMin(root) |
||||
fmt.Println(min.Data) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreemin$ go build |
||||
student@ubuntu:~/student/btreemin$ ./btreemin |
||||
1 |
||||
student@ubuntu:~/student/btreemin$ |
||||
``` |
@ -0,0 +1,48 @@
|
||||
## printroot |
||||
|
||||
### Instructions |
||||
|
||||
Write a function to print the value of the root node of a binary tree. |
||||
You have to create a new number and print the value of data |
||||
The nodes must be defined as follows: |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
type TreeNode struct { |
||||
left, right *TreeNode |
||||
data string |
||||
} |
||||
|
||||
func PrintRoot(root *TreeNode){ |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
//rootNode initialized with the value "who" |
||||
//rootNode1 initialized with the value "are" |
||||
//rootNode2 initialized with the value "you" |
||||
printRoot(rootNode) |
||||
printRoot(rootNode1) |
||||
printRoot(rootNode2) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/printroot$ go build |
||||
student@ubuntu:~/piscine/printroot$ ./printroot |
||||
who |
||||
are |
||||
you |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,74 @@
|
||||
## btreeinsertdata |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that searches for an item with a data element equal to elem and return that node |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeSearchItem(root *piscine_test.TreeNode, elem string) *piscine_test.TreeNode { |
||||
|
||||
} |
||||
|
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine "." |
||||
) |
||||
|
||||
func main() { |
||||
root := &piscine_test.TreeNode{Data: "4"} |
||||
piscine_test.BTreeInsertData(root, "1") |
||||
piscine_test.BTreeInsertData(root, "7") |
||||
piscine_test.BTreeInsertData(root, "5") |
||||
selected := BTreeSearchItem(root, "7") |
||||
fmt.Print("Item selected -> ") |
||||
if selected != nil { |
||||
fmt.Println(selected.Data) |
||||
} else { |
||||
fmt.Println("nil") |
||||
} |
||||
|
||||
fmt.Print("Parent of selected item -> ") |
||||
if selected.Parent != nil { |
||||
fmt.Println(selected.Parent.Data) |
||||
} else { |
||||
fmt.Println("nil") |
||||
} |
||||
|
||||
fmt.Print("Left child of selected item -> ") |
||||
if selected.Left != nil { |
||||
fmt.Println(selected.Left.Data) |
||||
} else { |
||||
fmt.Println("nil") |
||||
} |
||||
|
||||
fmt.Print("Right child of selected item -> ") |
||||
if selected.Right != nil { |
||||
fmt.Println(selected.Right.Data) |
||||
} else { |
||||
fmt.Println("nil") |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/btreesearchitem$ go build |
||||
student@ubuntu:~/piscine/btreesearchitem$ ./btreesearchitem |
||||
Item selected -> 7 |
||||
Parent of selected item -> 4 |
||||
Left child of selected item -> 5 |
||||
Right child of selected item -> nil |
||||
student@ubuntu:~/piscine/btreesearchitem$ |
||||
``` |
@ -0,0 +1,51 @@
|
||||
## btreetransplant |
||||
|
||||
### Instructions |
||||
|
||||
In order to move subtrees around within the binary search tree, write a function, BTreeTransplant, which replaces the subtree started by node with the node called 'rplc' in the tree given by root. |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func BTreeTransplant(root, node, rplc *TreeNode) *TreeNode { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
root := &student.TreeNode{Data: "4"} |
||||
student.BTreeInsertData(root, "1") |
||||
student.BTreeInsertData(root, "7") |
||||
student.BTreeInsertData(root, "5") |
||||
node := student.BTreeSearchItem(root, "1") |
||||
replacement := &student.TreeNode{Data: "3"} |
||||
root = student.BTreeTransplant(root, node, replacement) |
||||
student.BTreeApplyInorder(root, fmt.Println) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/btreetransplant$ go build |
||||
student@ubuntu:~/student/btreetrandsplant$ ./btreetransplant |
||||
3 |
||||
4 |
||||
5 |
||||
7 |
||||
student@ubuntu:~/student/btreetrandsplant$ |
||||
``` |
@ -0,0 +1,37 @@
|
||||
## Cat |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that does the same thing as the system's `cat` command-line. |
||||
|
||||
- You don't have to handle options. |
||||
|
||||
- But if just call the program with out arguments it should take a input and print it back |
||||
|
||||
- In the program folder create two files named `quest8.txt` and `quest8T.txt`. |
||||
|
||||
- Copy to the `quest8.txt` file this : |
||||
|
||||
- "Programming is a skill best acquired by pratice and example rather than from books" by Alan Turing |
||||
|
||||
- Copy to the `quest8T.txt` file this : |
||||
|
||||
- "Alan Mathison Turing was an English mathematician, computer scientist, logician, cryptanalyst. Turing was highly influential in the development of theoretical computer science, providing a formalisation of the concepts of algorithm and computation with the Turing machine, which can be considered a model of a general-purpose computer. Turing is widely considered to be the father of theoretical computer science and artificial intelligence." |
||||
|
||||
- In case of error it should print the error. |
||||
|
||||
### Output: |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
Hello |
||||
Hello |
||||
student@ubuntu:~/student/test$ ./test quest8.txt |
||||
"Programming is a skill best acquired by pratice and example rather than from books" by Alan Turing |
||||
student@ubuntu:~/student/test$ ./test quest8.txt quest8T.txt |
||||
"Programming is a skill best acquired by pratice and example rather than from books" by Alan Turing |
||||
|
||||
"Alan Mathison Turing was an English mathematician, computer scientist, logician, cryptanalyst. Turing was highly influential in the development of theoretical computer science, providing a formalisation of the concepts of algorithm and computation with the Turing machine, which can be considered a model of a general-purpose computer. Turing is widely considered to be the father of theoretical computer science and artificial intelligence." |
||||
|
||||
``` |
@ -0,0 +1,21 @@
|
||||
## cl-camp1 |
||||
|
||||
### Instructions |
||||
|
||||
A little voice speaks in your head: |
||||
|
||||
"Now that you know who you are. You need to remember what you can do..." |
||||
|
||||
The instincts are coming back... |
||||
|
||||
Put in a file `mastertheLS` the command line that will: |
||||
|
||||
- list the files and folders of the current folder. |
||||
- Ignore the hidden files, the "." and the "..". |
||||
- Separates the resuls with commas. |
||||
- Order them by ascending order of creation date. |
||||
- Have the folders have a `/` in front of them. |
||||
|
||||
### Hint |
||||
|
||||
Read the man... |
@ -0,0 +1,42 @@
|
||||
## collatzcountdown |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, CollatzCountdown, that returns the number of steps to reach 1 using the collatz countdown. |
||||
|
||||
The function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func CollatzCountdown(start int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
steps := student.CollatzCountdown(12) |
||||
fmt.Println(steps) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/collatzcountdown$ go build |
||||
student@ubuntu:~/student/collatzcountdown$ ./collatzcountdown |
||||
10 |
||||
student@ubuntu:~/student/collatzcountdown$ |
||||
``` |
@ -0,0 +1,47 @@
|
||||
## ROT 14 |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `rot14` that returns the string within the parameter but transformed into a rot14 string. |
||||
|
||||
- If you not certain what we are talking about, there is a rot13 already. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func rot14(str string) string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
result := rot14("Hello How are You") |
||||
arrayRune := []rune(result) |
||||
|
||||
for _, s := range arrayRune { |
||||
z01.PrintRune(s) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Vszzc Vck ofs Mci |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,114 @@
|
||||
--- |
||||
tier: 0 |
||||
team: 1 |
||||
duration: 1 hour |
||||
objectives: reading the rules |
||||
skills: git, github, reading |
||||
--- |
||||
|
||||
## commandments |
||||
|
||||
A few basic principles to follow |
||||
|
||||
<p align="center"> |
||||
<img width="476" height="600" src="https://upload.wikimedia.org/wikipedia/commons/thumb/e/e5/MCC-31231_Mozes_toont_de_wetstafelen_%281%29.tif/lossy-page1-476px-MCC-31231_Mozes_toont_de_wetstafelen_%281%29.tif.jpg"> |
||||
</p> |
||||
|
||||
### The Commandements _(read them)_ |
||||
|
||||
- Le numérique est ta passion. |
||||
|
||||
- Ton objectif à 42 : développer ton talent et tes compétences pour le marché du numérique. |
||||
|
||||
- L’objectif de 42 pour toi : te faire accéder au marché de l’emploi, à une longue et pérenne carrière dans le numérique, et te faire progresser socialement. |
||||
|
||||
- L’ambition de 42 pour toi : être un pionnier du numérique de demain. |
||||
|
||||
- Il est de ta responsabilité de gérer ta progression : 42 te propose un parcours individualisé adapté à ton rythme. |
||||
|
||||
- Des challenges jalonnent ton parcours. 42 ne fournira aucun élément de solution. C’est ton rôle de chercher et trouver par toi-même ces solutions pour atteindre l’objectif. |
||||
|
||||
- Sois actif. N’attend pas que les choses se fassent pour toi. 42 est un parcours 100% pratique. |
||||
|
||||
- Sois autonome dans ton cursus. N’attend pas que l’on te dise quoi faire. |
||||
|
||||
- Sois collaboratif, autant sur les projets solos que les projets de groupe. Face aux challenges à resoudre, l’échange et le debat sont tes meilleures armes. |
||||
|
||||
- Ne "crois" pas. Sois sûr. Techniquement, relationnelement, organisationellement, administrativement. |
||||
|
||||
- Pour être sûr, teste, contrôle. |
||||
|
||||
- N’ai pas peur de te tromper, d’échouer. L’échec est une étape normale vers le succès. |
||||
|
||||
- Teste Ă nouveau. Collabore davantage pour tester davantage. |
||||
|
||||
- Ose. Le risque est de se tromper. Voir le commandement 12. |
||||
|
||||
- Sois rigoureux dans ton travail. |
||||
|
||||
- Sois investi dans ton cursus : 50 heures par semaine est un minimum. Ta capacité de travail est une valeur. L’école est ouverte 24h/24 et 7j/7. |
||||
|
||||
- Sois régulier dans ton travail. Un jour oui, un jour non, un coup nocturne, un coup diurne... le chaos t’empêche d’avancer. |
||||
|
||||
- Prévois un groupe de travail large et hétérogène pour y trouver facilement des idées neuves et des groupes de projet. |
||||
|
||||
- Pour tes collaborations et ton travail en groupe, privilégie des étudiants qui n’ont pas déjà la solution au problème. |
||||
|
||||
- Sois investi dans ton groupe de projet, et ne le laisse pas faire ton travail Ă ta place. |
||||
|
||||
- Ton groupe de projet est solidaire, son succès comme son échec est de la responsabilité de tous, et les conflits se règlent en interne. |
||||
|
||||
- Travaille à l’école. Faire du peer-learning, collaborer, cela demande d’être physiquement avec les autres. A distance cela ne fonctionne pas. |
||||
|
||||
- Implique toi dans les évaluations de tes projets. Elles te permettent de prendre du recul. |
||||
|
||||
- Implique toi dans tes évaluations des projets des autres. La qualité de la communauté en dépend. |
||||
|
||||
- Sois juste et teste rigoureusement tes projets comme ceux des autres en évaluation avec tes propres jeux de tests. |
||||
|
||||
- Joue pleinement le jeu de ta scolarité dans l’état d’esprit demandé, fait tous les exercices et projets demandés. |
||||
|
||||
- Ne cherche pas des biais et des failles dans le système. Tu vas fausser ta propre formation et ta valeur sur le marché. |
||||
|
||||
- Ne triche pas intentionellement. C’est amoral, cela contredit le commandement 12, et c’est du temps inutilement passé à ne pas développer tes compétences pour faire croire aux autres que tu sais coder alors que ce n’est pas le cas. |
||||
|
||||
- Ne rends pas un projet que tu ne serais pas capable de reproduire seul à huis clos. Même si c’est parfois involontaire, c’est aussi de la triche. |
||||
|
||||
- C’est pas pour tes parents que tu travailles, ni pour le staff. C’est pour toi. |
||||
|
||||
- Participe à la vie de la communauté, à son épanouissement, et sa qualité en sortie de formation. |
||||
|
||||
- Aide au respect de ces commandements par la communauté. |
||||
|
||||
- Sois bienveillant et empathique vis à vis de tes camarades comme des personnes avec qui tu interagis, échanges, débats. |
||||
|
||||
- N’ai pas peur du monde professionnel. |
||||
|
||||
- Respecte le matériel. Des consignes spécifiques sont données en ce sens. |
||||
|
||||
- Respecte les locaux. Des consignes spécifiques sont données en ce sens. |
||||
|
||||
- Respecte les gens, étudiants, staffs, partenaires, visiteurs. |
||||
|
||||
- Respecte la loi en vigueur dans le pays. |
||||
|
||||
- Respecte les lois et consignes en vigueur liées à la consommation d’alcool. |
||||
|
||||
- Respecte les lois et consignes en vigueur liées à la consommation de tabac, stupéfiants, ou produits assimilés. |
||||
|
||||
- N’hésite pas à interagir avec le staff, pour parler de tes problèmes, pour remonter des problèmes dans le cursus, pour contribuer au cursus. |
||||
|
||||
- Si tu t’interroges ou ne comprends pas nos choix pédagogiques, demande nous. On ne fait généralement rien au hasard. |
||||
|
||||
### Required |
||||
|
||||
You [clone](http://lmgtfy.com/?q=git+clone) your [fork](http://lmgtfy.com/?q=github+fork) of this [repository](http://lmgtfy.com/?q=git+repository) |
||||
and in it, you must create a file named `turn_in` () in which you write EXACTLY the following sentence ending by a line break. |
||||
|
||||
<p align="center"> |
||||
<img width="600" height="300" src="https://i.imgur.com/2PPQ2iZ.png"> |
||||
</p> |
||||
|
||||
### Submiting your solution |
||||
|
||||
Your work should be commited and pushed in the master branch of your own fork of this repository. |
@ -0,0 +1,41 @@
|
||||
## Compact |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that will take a pointer to a array as parameter and overwrites any element that points to `nil`. |
||||
|
||||
- If you not sure what the function does. It exists in Ruby. |
||||
|
||||
### Expected functions |
||||
|
||||
```go |
||||
func Compact(ptr *[]string, length int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import fmt |
||||
|
||||
func main() { |
||||
array := []string{"hello", " ", "there", " ", "bye"} |
||||
|
||||
ptr := &array |
||||
fmt.Println(Compact(ptr, len(array))) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
3 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,45 @@
|
||||
## createelem |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `CreateElem` that creates a new element of type`Node`. |
||||
|
||||
### Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
} |
||||
|
||||
func CreateElem(n *Node, value int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
n := &node{} |
||||
n.CreateElem(1234) |
||||
fmt.Println(n) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
&{1234} |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,17 @@
|
||||
## dispfirstpar |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes strings as arguments, and displays its first argument. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test hello there |
||||
hello |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" how are you |
||||
hello there |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,19 @@
|
||||
## displastpar |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes strings as arguments, and displays its last argument. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test hello there |
||||
there |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" how are you |
||||
you |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" |
||||
hello there |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,25 @@
|
||||
## Display File |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that displays, on the standard output, only the content of the file given as argument. |
||||
|
||||
- Create a file `quest8.txt` and write into the file `Almost there!!` |
||||
|
||||
- The argument of the program should be the name of the file, in this case, `quest8.txt`. |
||||
|
||||
- In case of error it should print: |
||||
- `File name missing`. |
||||
- `Too many arguments`. |
||||
|
||||
### Output: |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
File name missing |
||||
student@ubuntu:~/student/test$ ./test quest8.txt main.go |
||||
Too many arguments |
||||
student@ubuntu:~/student/test$ ./test quest8.txt |
||||
Almost there!! |
||||
``` |
@ -0,0 +1,86 @@
|
||||
## enigma |
||||
|
||||
### Instructions |
||||
|
||||
Write a function called `Enigma` that receives poiters to functions and move its values around to hide them |
||||
|
||||
This function will put a into c; c into d; d into b and b into a |
||||
|
||||
This function must have the following signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Enigma(a ***int, b *int, c *******int, d ****int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
x := 5 |
||||
y := &x |
||||
z := &y |
||||
a := &z |
||||
|
||||
w := 2 |
||||
b := &w |
||||
|
||||
u := 7 |
||||
e := &u |
||||
f := &e |
||||
g := &f |
||||
h := &g |
||||
i := &h |
||||
j := &i |
||||
c := &j |
||||
|
||||
k := 6 |
||||
l := &k |
||||
m := &l |
||||
n := &m |
||||
d := &n |
||||
|
||||
fmt.Println(***a) |
||||
fmt.Println(*b) |
||||
fmt.Println(*******c) |
||||
fmt.Println(****d) |
||||
|
||||
student.Enigma(a, b, c, d) |
||||
|
||||
fmt.Println("After using Enigma") |
||||
fmt.Println(***a) |
||||
fmt.Println(*b) |
||||
fmt.Println(*******c) |
||||
fmt.Println(****d) |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/enigma$ go build |
||||
student@ubuntu:~/student/enigma$ ./enigma |
||||
5 |
||||
2 |
||||
7 |
||||
6 |
||||
After using Enigma |
||||
2 |
||||
6 |
||||
5 |
||||
7 |
||||
student@ubuntu:~/student/enigma$ |
||||
``` |
@ -0,0 +1,28 @@
|
||||
## expandstr |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string and displays it with exactly three spaces |
||||
between each word, with no spaces or tabs at either the beginning nor the end. |
||||
|
||||
The string will be followed by a newline. |
||||
|
||||
A word is a sequence of alphanumerical characters. |
||||
|
||||
If the number of parameters is not 1, or if there are no words, the program displays |
||||
a newline. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/expandstr$ go build |
||||
student@ubuntu:~/student/expandstr$ ./expandstr "you see it's easy to display the same thing" | cat -e |
||||
you see it's easy to display the same thing$ |
||||
student@ubuntu:~/student/expandstr$ ./expandstr " only it's harder " | cat -e |
||||
only it's harder$ |
||||
student@ubuntu:~/student/expandstr$ ./expandstr " how funny it is" "did you hear, Mathilde ?" | cat -e |
||||
$ |
||||
student@ubuntu:~/student/expandstr$ ./expandstr | cat -e |
||||
$ |
||||
student@ubuntu:~/student/expandstr$ |
||||
``` |
@ -0,0 +1 @@
|
||||
## firebase-demo |
@ -0,0 +1,26 @@
|
||||
## firstword |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string and displays its first word, followed by a newline. |
||||
|
||||
- A word is a section of string delimited by spaces or by the start/end of the string. |
||||
|
||||
- The output will be followed by a newline. |
||||
|
||||
- If the number of parameters is not 1, or if there are no words, simply display a newline. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" |
||||
hello |
||||
student@ubuntu:~/piscine/test$ ./test "hello ......... bye" |
||||
hello |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test "hello" "there" |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,66 @@
|
||||
## Fix the Main |
||||
|
||||
### Instructions |
||||
|
||||
Write and fix the folloing functions. |
||||
|
||||
### Expected functions |
||||
|
||||
```go |
||||
func PutStr(str string) { |
||||
arrayRune := []rune(str) |
||||
for _, s := range arrayRune { |
||||
z01.PrintRune(s) |
||||
} |
||||
} |
||||
|
||||
func CloseDoor(ptrDoor *Door) bool { |
||||
PutStr("Door closing...") |
||||
state = CLOSE |
||||
return true |
||||
} |
||||
|
||||
func IsDoorOpen(Door Door) { |
||||
PutStr("Door is open ?") |
||||
return Door.state = OPEN |
||||
} |
||||
|
||||
func IsDoorClose(ptrDoor *Door) bool { |
||||
PutStr("Door is close ?") |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
door := &Door{} |
||||
|
||||
OpenDoor(door) |
||||
if IsDoorClose(door) { |
||||
OpenDoor(door) |
||||
} |
||||
if IsDoorOpen(door) { |
||||
CloseDoor(door) |
||||
} |
||||
if door.state == OPEN { |
||||
CloseDoor(door) |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Door Opening... |
||||
Door is close ? |
||||
Door is open ? |
||||
Door closing... |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,30 @@
|
||||
## fprime |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a positive `int` and displays its prime factors on the standard output, followed by a newline. |
||||
|
||||
- Factors must be displayed in ascending order and separated by `*`, so that the expression in the output gives the right result. |
||||
|
||||
- If the number of parameters is not 1, the program displays a newline. |
||||
|
||||
- The input, when there is one, will always be valid. |
||||
|
||||
Example of output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test 225225 |
||||
3*3*5*5*7*11*13 |
||||
student@ubuntu:~/piscine/test$ ./test 8333325 |
||||
3*3*5*5*7*11*13*37 |
||||
student@ubuntu:~/piscine/test$ ./test 9539 |
||||
9539 |
||||
student@ubuntu:~/piscine/test$ ./test 804577 |
||||
804577 |
||||
student@ubuntu:~/piscine/test$ ./test 42 |
||||
2*3*7 |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,3 @@
|
||||
## functions |
||||
|
||||
This repository aggregate every functions of the Zone 01 organization |
@ -0,0 +1,32 @@
|
||||
## gcd |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes two strings representing two strictly positive |
||||
integers that fit in an int. |
||||
|
||||
Display their greatest common divisor followed by a newline (It is always a |
||||
strictly positive integer). |
||||
|
||||
If the number of parameters is not 2, display a newline. |
||||
|
||||
All arguments tested will be valid positive `int` values. |
||||
|
||||
Example of output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/gcd$ go build |
||||
student@ubuntu:~/student/gcd$ ./gcd 42 10 | cat -e |
||||
2$ |
||||
student@ubuntu:~/student/gcd$ ./gcd 42 12 | cat -e |
||||
6$ |
||||
student@ubuntu:~/student/gcd$ ./gcd 14 77 | cat -e |
||||
7$ |
||||
student@ubuntu:~/student/gcd$ ./gcd 17 3 | cat -e |
||||
1$ |
||||
student@ubuntu:~/student/gcd$ ./gcd | cat -e |
||||
$ |
||||
student@ubuntu:~/student/gcd$ ./gcd 50 12 4 | cat -e |
||||
$ |
||||
student@ubuntu:~/student/gcd$ |
||||
``` |
@ -0,0 +1,28 @@
|
||||
## hiddenp |
||||
|
||||
### Instructions |
||||
|
||||
Write a program named hiddenp that takes two strings and that, if the first string is hidden in the second one, displays 1 |
||||
followed by a newline, otherwise it displays 0 followed by a newline. |
||||
|
||||
Let s1 and s2 be strings. It is considered that s1 is hidden in s2 if it is possible to |
||||
find each character from s1 in s2, **in the same order as they appear in s1.** |
||||
|
||||
If s1 is an empty string it is considered hidden in any string. |
||||
|
||||
If the number of parameters is not 2, the program displays a newline. |
||||
|
||||
Example of output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/hiddenp$ go build |
||||
student@ubuntu:~/student/hiddenp$ ./hiddenp "fgex.;" "tyf34gdgf;'ektufjhgdgex.;.;rtjynur6" | cat -e |
||||
1$ |
||||
student@ubuntu:~/student/hiddenp$ ./hiddenp "abc" "2altrb53c.sse" | cat -e |
||||
1$ |
||||
student@ubuntu:~/student/hiddenp$ ./hiddenp "abc" "btarc" | cat -e |
||||
0$ |
||||
student@ubuntu:~/student/hiddenp$ ./hiddenp | cat -e |
||||
$ |
||||
student@ubuntu:~/student/hiddenp$ |
||||
``` |
@ -0,0 +1,20 @@
|
||||
## switchcase |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes two strings and displays, without doubles, the characters that appear in both strings, in the order they appear in the first one. |
||||
|
||||
- The display will be followed by a `\n`. |
||||
|
||||
- If the number of arguments is not 2, the program displays `\n`. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "padinton" "paqefwtdjetyiytjneytjoeyjnejeyj" |
||||
padinto |
||||
student@ubuntu:~/piscine/test$ ./test ddf6vewg64f twthgdwthdwfteewhrtag6h4ffdhsd |
||||
df6ewg4 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,15 @@
|
||||
## ispowerof2 |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that determines if a given number is a power of 2. |
||||
|
||||
This function returns true if the given number is a power of 2, otherwise it returns false. |
||||
|
||||
## Expected function |
||||
|
||||
```go |
||||
func IsPowerOf2(n uint) bool { |
||||
|
||||
} |
||||
``` |
@ -0,0 +1,15 @@
|
||||
## itoa |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that simulates the behaviour of the `Itoa` function in Go. `Itoa` transforms a number represented as an`int` in a number represented as a `string`. |
||||
|
||||
- For this exercise the handling of the signs + or - **does have** to be taken into account. |
||||
|
||||
## Expected function |
||||
|
||||
```go |
||||
func Itoa(n int) string { |
||||
|
||||
} |
||||
``` |
@ -0,0 +1,24 @@
|
||||
## itoabase |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that: |
||||
|
||||
- converts an integer value to a string using the specified base in the argument |
||||
- and then returns this string |
||||
|
||||
The base is expressed as an integer, from 2 to 16. The characters comprising |
||||
the base are the digits from 0 to 9, followed by uppercase letters from A to F. |
||||
|
||||
For example, the base `4` would be the equivalent of "0123" and the base `16` would be the equivalent of "0123456789ABCDEF". |
||||
|
||||
If the value is negative, the resulting string has to be preceded with a |
||||
minus sign `-`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ItoaBase(value, base int) string { |
||||
|
||||
} |
||||
``` |
@ -0,0 +1,29 @@
|
||||
## lastword |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string and displays its last word, followed by a |
||||
newline. |
||||
|
||||
A word is a section of string delimited by spaces/tabs or by the start/end of |
||||
the string. |
||||
|
||||
If the number of parameters is not 1, or if there are no words, simply display |
||||
a newline. |
||||
|
||||
Example : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/lastword$ go build |
||||
student@ubuntu:~/student/lastword$ ./lastword "FOR PONY" | cat -e |
||||
PONY$ |
||||
student@ubuntu:~/student/lastword$ ./lastword "this ... is sparta, then again, maybe not" | cat -e |
||||
not$ |
||||
student@ubuntu:~/student/lastword$ ./lastword " " | cat -e |
||||
$ |
||||
student@ubuntu:~/student/lastword$ ./lastword "a" "b" | cat -e |
||||
$ |
||||
student@ubuntu:~/student/lastword$ ./lastword " lorem,ipsum " | cat -e |
||||
lorem,ipsum$ |
||||
student@ubuntu:~/student/lastword$ |
||||
``` |
@ -0,0 +1,20 @@
|
||||
## listpushpara |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that creates a new linked list and includes each command-line argument in to the list. |
||||
|
||||
- The first argument should be at the end of the list |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./listpushparams choumi is the best cat |
||||
cat |
||||
best |
||||
the |
||||
is |
||||
choumi |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,43 @@
|
||||
## max |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, Max, that returns the maximum value in a slice of integers |
||||
|
||||
The function must have the next signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Max(arr []int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
arrInt := []int{23, 123, 1, 11, 55, 93} |
||||
max := student.Max(arrInt) |
||||
fmt.Println(max |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/max$ go build |
||||
student@ubuntu:~/student/max$ ./max |
||||
123 |
||||
student@ubuntu:~/student/max$ |
||||
``` |
@ -0,0 +1,34 @@
|
||||
# options |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes an undefined number of arguments which could be considered as options and writes on the standard output a representation of those options as groups of bytes followed by a newline. |
||||
|
||||
- An option is an argument that begins by a `-` and have multiple characters which could be : |
||||
- abcdefghijklmnopqrstuvwxyz |
||||
|
||||
- All options are stocked in a single int and each options represents a bit of that int, and should be stocked like this : |
||||
|
||||
- 00000000 00000000 00000000 00000000 |
||||
- ******zy xwvutsrq ponmlkji hgfedcba |
||||
|
||||
- Launching the program without arguments or with the `-h` flag activated must print all the valid options on the standard output, as shown on one of the following examples. |
||||
|
||||
- A wrong option must print "Invalid Option" followed by a newline. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test | cat -e |
||||
options: abcdefghijklmnopqrstuvwxyz$ |
||||
student@ubuntu:~/piscine/test$ ./test -abc -ijk | cat -e |
||||
00000000 00000000 00000111 00000111$ |
||||
student@ubuntu:~/piscine/test$ ./test -z | cat -e |
||||
00000010 00000000 00000000 00000000$ |
||||
student@ubuntu:~/piscine/test$ ./test -abc -hijk | cat -e |
||||
options: abcdefghijklmnopqrstuvwxyz$ |
||||
student@ubuntu:~/piscine/test$ ./test -% | cat -e |
||||
Invalid Option$ |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,21 @@
|
||||
## paramcount |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that displays the number of arguments passed to it. This number will be followed by |
||||
a newline. |
||||
|
||||
If there are no arguments, just display a 0 followed by a newline. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/paramcount$ go build |
||||
student@ubuntu:~/student/paramcount$ ./paramcount 1 2 3 5 7 24 |
||||
6 |
||||
student@ubuntu:~/student/paramcount$ ./paramcount 6 12 24 | cat -e |
||||
3$ |
||||
student@ubuntu:~/student/paramcount$ ./paramcount | cat -e |
||||
0$ |
||||
student@ubuntu:~/student/paramcount$ |
||||
``` |
@ -0,0 +1,26 @@
|
||||
## pilot |
||||
|
||||
### Instructions |
||||
|
||||
Write a go file so that the following program compile |
||||
|
||||
### Usage |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
var donnie student.Pilot |
||||
donnie.Name = "Donnie" |
||||
donnie.Life = 100.0 |
||||
donnie.Age = 24 |
||||
donnie.Aircraft = student.AIRCRAFT1 |
||||
|
||||
fmt.Println(donnie) |
||||
} |
||||
``` |
@ -0,0 +1,32 @@
|
||||
## Point |
||||
|
||||
### Instructions |
||||
|
||||
Create a `.go` file and copy the code below into our file |
||||
|
||||
- The main task is to return a working program. |
||||
|
||||
```go |
||||
func setPoint(ptr *point) { |
||||
ptr.x = 42 |
||||
ptr.y = 21 |
||||
} |
||||
|
||||
func main() { |
||||
points := &point{} |
||||
|
||||
setPoint(points) |
||||
|
||||
fmt.Printf("x = %d, y = %d",points.x, points.y) |
||||
fmt.Println() |
||||
} |
||||
``` |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
x = 42, y = 21 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,19 @@
|
||||
## printbits |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that takes a byte, and prints it in binary value **without a newline at the end**. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func PrintBits(octe byte) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Example of output: |
||||
|
||||
If 2 is passed to the function PrintBits, it will print "00000010". |
@ -0,0 +1,22 @@
|
||||
## printhex |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a positive (or zero) number expressed in base 10, and displays it in base 16 ( with lowercase letters) followed by a newline. |
||||
|
||||
- If the number of parameters is not 1, the program displays a newline. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "10" |
||||
a |
||||
student@ubuntu:~/piscine/test$ ./test "255" |
||||
ff |
||||
student@ubuntu:~/piscine/test$ ./test "5156454" |
||||
4eae66 |
||||
student@ubuntu:~/piscine/test$ |
||||
|
||||
student@ubuntu:~/piscine/ |
||||
``` |
@ -0,0 +1,36 @@
|
||||
## printmemory |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that takes `(arr [10]int)`, and displays the memory as in the example. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func PrintMemory(arr [10]int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
func main() { |
||||
arr := [10]int{104, 101, 108, 108, 111, 16, 21, 42} |
||||
PrintMemory(arr) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test | cat -e |
||||
6800 0000 6500 0000 6c00 0000 6c00 0000 $ |
||||
6f00 0000 1000 0000 1500 0000 2a00 0000 $ |
||||
0000 0000 0000 0000 $ |
||||
hello..*..$ |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1 @@
|
||||
## public |
@ -0,0 +1,45 @@
|
||||
## raid3 |
||||
|
||||
### Instructions |
||||
|
||||
Creare a program that takes a string as argument and displays the name of the raid in question, as well as its dimensions. |
||||
|
||||
- Executable name : `raid3`. |
||||
|
||||
- If the argument isn't a raid should print `Not a Raid function`. |
||||
|
||||
Example of this output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ echo HELLO | ./raid3 |
||||
Not a Raid function |
||||
student@ubuntu:~/student/test$ |
||||
``` |
||||
|
||||
- Whatever the answer, your line must be ended by a `\n`. |
||||
|
||||
- If there is more than one raid1, you must display them all alphabetically. |
||||
|
||||
Examples of output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ ./raid1a 4 4 |
||||
o--o |
||||
| | |
||||
| | |
||||
o--o |
||||
student@ubuntu:~/student/test$ ./raid1a 4 4 | ./raid3 |
||||
[raid1a] [4] [4] |
||||
student@ubuntu:~/student/test$ ./raid1a 3 4 | ./raid3 |
||||
[raid1a] [3] [4] |
||||
student@ubuntu:~/student/test$ ./raid1c 1 1 |
||||
A |
||||
student@ubuntu:~/student/test$ ./raid1d 1 1 |
||||
A |
||||
student@ubuntu:~/student/test$ ./raid1e 1 1 |
||||
A |
||||
student@ubuntu:~/student/test$ ./raid1c 1 1 | ./raid3 |
||||
[raid1c] [1] [1] || [raid1d] [1] [1] || [raid1e] [1] [1] |
||||
student@ubuntu:~/student/test$ |
||||
``` |
@ -0,0 +1,24 @@
|
||||
## range |
||||
|
||||
### Instructions |
||||
|
||||
Write the function Range which must: |
||||
|
||||
- allocate (with make()) an array of integers. |
||||
- fill it with consecutive values that begin at `start` and end at `end` (Including `start` and `end` !) |
||||
- finally return that array. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Range(start, end int) []int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
Examples of outputs : |
||||
|
||||
- With (1, 3) you will return an array containing 1, 2 and 3. |
||||
- With (-1, 2) you will return an array containing -1, 0, 1 and 2. |
||||
- With (0, 0) you will return an array containing 0. |
||||
- With (0, -3) you will return an array containing 0, -1, -2 and -3. |
@ -0,0 +1,55 @@
|
||||
## Rectangle |
||||
|
||||
### Instructions |
||||
|
||||
Consider that a point is defined by its coordinates and that a rectangle |
||||
is defined by the points of the upper left and lower right corners. |
||||
|
||||
- Define two structures named, `point` and `rectangle`. |
||||
|
||||
- The struct `point` has to have two variables, `x` and `y`, type `int`. |
||||
|
||||
- The struct `rectangle` has to have two variables, `upLeft` and `downRight` type `point`. |
||||
|
||||
- Our main task is to make a program that: |
||||
|
||||
- Given a slice of points of size `n` returns the smallest rectangle that contains all the points in the vector of points. The name of that function is `defineRectangle` |
||||
|
||||
- And calculates and prints the area of that rectangle you define. |
||||
|
||||
### Expected main and function for the program |
||||
|
||||
```go |
||||
func defineRectangle(ptr *point, n int) *rectangle { |
||||
|
||||
} |
||||
|
||||
func calArea(ptr *rectangle) int { |
||||
|
||||
} |
||||
|
||||
func main() { |
||||
vPoint := []point{} |
||||
rectangle := &rectangle{} |
||||
n := 7 |
||||
|
||||
for i := 0; i < n; i++ { |
||||
val := point{ |
||||
x: i%2 + 1, |
||||
y: i + 2, |
||||
} |
||||
vPoint = append(vPoint, val) |
||||
} |
||||
rectangle = defineRectangle(vPoint, n) |
||||
fmt.Println("area of the rectangle:", calArea(rectangle)) |
||||
} |
||||
``` |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
area of the rectangle: 6 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,30 @@
|
||||
## repeatalpha |
||||
|
||||
### Instructions |
||||
|
||||
Write a program called repeat_alpha that takes a string and display it |
||||
repeating each alphabetical character as many times as its alphabetical index, |
||||
followed by a newline. |
||||
|
||||
`'a'` becomes `'a'`, `'b'` becomes `'bb'`, `'e'` becomes `'eeeee'`, etc... |
||||
|
||||
Case remains unchanged. |
||||
|
||||
If the number of arguments is not 1, just display a newline. |
||||
|
||||
Examples: |
||||
|
||||
```console |
||||
student@ubuntu:~/student/repeatalpha$ go build |
||||
student@ubuntu:~/student/repeatalpha$ ./repeatalpha "abc" | cat -e |
||||
abbccc |
||||
student@ubuntu:~/student/repeatalpha$ ./repeatalpha "Alex." | cat -e |
||||
Alllllllllllleeeeexxxxxxxxxxxxxxxxxxxxxxxx.$ |
||||
student@ubuntu:~/student/repeatalpha$ ./repeatalpha "abacadaba 42!" | cat -e |
||||
abbacccaddddabba 42!$ |
||||
student@ubuntu:~/student/repeatalpha$ ./repeatalpha | cat -e |
||||
$ |
||||
student@ubuntu:~/student/repeatalpha$ ./repeatalpha "" | cat -e |
||||
$ |
||||
student@ubuntu:~/student/repeatalpha$ |
||||
``` |
@ -0,0 +1,27 @@
|
||||
## reversebits |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that takes a byte, reverses it, bit by bit (like the |
||||
example) and returns the result. |
||||
|
||||
Your function must be declared as follows: |
||||
|
||||
```go |
||||
func ReverseBits(octet byte) byte { |
||||
|
||||
} |
||||
``` |
||||
|
||||
Example: |
||||
|
||||
1 byte |
||||
|
||||
--- |
||||
|
||||
``` |
||||
00100110 |
||||
|| |
||||
\/ |
||||
01100100 |
||||
``` |
@ -0,0 +1,24 @@
|
||||
## reverserange |
||||
|
||||
### Instructions |
||||
|
||||
Write the function ReverseRange which must: |
||||
|
||||
- allocate (with make()) an array of integers. |
||||
- fill it with consecutive values that begin at `end` and end at `start` (Including `start` and `end` !) |
||||
- finally return that array. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ReverseRange(start, end int) []int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
Examples of output : |
||||
|
||||
- With (1, 3) the function will return an array containing 3, 2 and 1. |
||||
- With (-1, 2) the function will return an array containing 2, 1, 0 and -1. |
||||
- With (0, 0) the function will return an array containing 0. |
||||
- With (0, -3) the function will return an array containing -3, -2, -1 and 0. |
@ -0,0 +1,27 @@
|
||||
## reversestrcap |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes one or more strings and that, **for each argument**: |
||||
-puts the last character of each word (if it is a letter) in uppercase and the rest |
||||
in lowercase |
||||
-then it displays the result followed by a `\n`. |
||||
|
||||
A word is a sequence of alphanumerical characters. |
||||
|
||||
If there are no parameters, the program displays a `\n`. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/reversestrcap$ go build |
||||
student@ubuntu:~/student/reversestrcap$ ./reversestrcap "First SMALL TesT" | cat -e |
||||
firsT smalL tesT$ |
||||
student@ubuntu:~/student/reversestrcap$ ./reversestrcap go run reversestrcap.go "SEconD Test IS a LItTLE EasIEr" "bEwaRe IT'S NoT HARd WhEN " " Go a dernier 0123456789 for the road e" | cat -e |
||||
seconD tesT iS A littlE easieR$ |
||||
bewarE it'S noT harD wheN $ |
||||
gO A dernieR 0123456789 foR thE roaD E$ |
||||
student@ubuntu:~/student/reversestrcap$ ./reversestrcap | cat -e |
||||
$ |
||||
student@ubuntu:~/student/reversestrcap$ |
||||
``` |
@ -0,0 +1,26 @@
|
||||
## revWstr |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string as a parameter, and prints its words in reverse. |
||||
|
||||
- A word is a sequence of **alphanumerical** characters. |
||||
|
||||
- If the number of parameters is different from 1, the program will display `\n`. |
||||
|
||||
- In the parameters that are going to be tested, there will not be any additional spaces. (meaning that there will not be additionnal spaces at the beginning or at the end of the string, and words will always be separated by exactly one space). |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "the time of contempt precedes that of indifference" |
||||
indifference of that precedes contempt of time the |
||||
student@ubuntu:~/piscine/test$ ./test "abcdefghijklm" |
||||
abcdefghijklm |
||||
student@ubuntu:~/piscine/test$ ./test "he stared at the mountain" |
||||
mountain the at stared he |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,29 @@
|
||||
## rostring |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string and displays this string after rotating it |
||||
one word to the left. |
||||
|
||||
Thus, the first word becomes the last, and others stay in the same order. |
||||
|
||||
A word is a sequence of **alphanumerical** characters. |
||||
|
||||
Words will be separated by only one space in the output. |
||||
|
||||
If the number of arguments is not one, the program displays a newline. |
||||
|
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/rostring$ go build |
||||
student@ubuntu:~/piscine/rostring$ ./rostring "abc " | cat -e |
||||
abc$ |
||||
student@ubuntu:~/piscine/rostring$ ./rostring "Let there be light" |
||||
there be light There |
||||
student@ubuntu:~/piscine/rostring$ ./rostring " AkjhZ zLKIJz , 23y" |
||||
zLKIJz , 23y AkjhZ |
||||
student@ubuntu:~/piscine/rostring$ ./rostring | cat -e |
||||
$ |
||||
student@ubuntu:~/piscine/rostring$ |
||||
``` |
@ -0,0 +1,25 @@
|
||||
## rot13 |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string and displays it, replacing each of its |
||||
letters by the letter 13 spaces ahead in alphabetical order. |
||||
|
||||
- 'z' becomes 'm' and 'Z' becomes 'M'. Case remains unaffected. |
||||
|
||||
- The output will be followed by a newline. |
||||
|
||||
- If the number of arguments is not 1, the program displays a newline. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "abc" |
||||
nop |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" |
||||
uryyb gurer |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,46 @@
|
||||
## ROT 14 |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `rot14` that returns the string within the parameter but transformed into a rot14 string. |
||||
|
||||
- If you not certain what we are talking about, there is a rot13 already. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func rot14(str string) string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
result := rot14("Hello How are You") |
||||
arrayRune := []rune(result) |
||||
|
||||
for _, s := range arrayRune { |
||||
z01.PrintRune(s) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Vszzc Vck ofs Mci |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,60 @@
|
||||
## rpncalc |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string which contains an equation written in |
||||
Reverse Polish notation (RPN) as its first argument, evaluates the equation, and |
||||
prints the result on the standard output followed by a newline. |
||||
|
||||
Reverse Polish Notation is a mathematical notation in which every operator |
||||
follows all of its operands. In RPN, every operator encountered evaluates the |
||||
previous 2 operands, and the result of this operation then becomes the first of |
||||
the two operands for the subsequent operator. Operands and operators must be |
||||
spaced by at least one space. |
||||
|
||||
The following operators must be implemented : `+`, `-`, `*`, `/`, and `%`. |
||||
|
||||
If the string is not valid or if there is not exactly one argument, `Error` must be printed |
||||
on the standard output followed by a newline. |
||||
If the string has extra spaces it is still valid. |
||||
|
||||
All the given operands must fit in a `int`. |
||||
|
||||
Examples of formulas converted in RPN: |
||||
|
||||
3 + 4 >> 3 4 + |
||||
((1 * 2) * 3) - 4 >> 1 2 * 3 * 4 - ou 3 1 2 * * 4 - |
||||
50 * (5 - (10 / 9)) >> 5 10 9 / - 50 * |
||||
|
||||
Here is how to evaluate a formula in RPN: |
||||
|
||||
``` |
||||
1 2 * 3 * 4 - |
||||
2 3 * 4 - |
||||
6 4 - |
||||
2 |
||||
``` |
||||
Or: |
||||
|
||||
``` |
||||
3 1 2 * * 4 - |
||||
3 2 * 4 - |
||||
6 4 - |
||||
2 |
||||
``` |
||||
Examples of outputs : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/rpncalc$ go build |
||||
student@ubuntu:~/student/rpncalc$ ./rpncalc "1 2 * 3 * 4 +" | cat -e |
||||
10$ |
||||
student@ubuntu:~/student/rpncalc$ ./rpncalc 1 2 3 4 +" | cat -e |
||||
Error$ |
||||
student@ubuntu:~/student/rpncalc$ ./rpncalc | cat -e |
||||
Error$ |
||||
student@ubuntu:~/student/rpncalc$ ./rpncalc " 1 3 * 2 -" | cat -e |
||||
1 |
||||
student@ubuntu:~/student/rpncalc$ ./rpncalc " 1 3 * ksd 2 -" | cat -e |
||||
Error$ |
||||
student@ubuntu:~/student/rpncalc$ |
||||
``` |
@ -0,0 +1,22 @@
|
||||
## searchreplace |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes 3 arguments, the first arguments is a string in which to replace a letter (2nd argument) by another one (3rd argument). |
||||
|
||||
- If the number of arguments is not 3, just display a newline. |
||||
|
||||
- If the second argument is not contained in the first one (the string) then the program simply rewrites the string followed by a newline. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "hella there" "a" "o" |
||||
hello there |
||||
student@ubuntu:~/piscine/test$ ./test "abcd" "z" "l" |
||||
abcd |
||||
student@ubuntu:~/piscine/test$ ./test "something" "a" "o" "b" "c" |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,42 @@
|
||||
## sortList |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that must: |
||||
|
||||
- Sort the list given as a parameter, using the function cmp to select the order to apply, |
||||
|
||||
- Return a pointer to the first element of the sorted list. |
||||
|
||||
Duplications must remain. |
||||
|
||||
Inputs will always be consistent. |
||||
|
||||
The `type NodeList` must be used. |
||||
|
||||
Functions passed as `cmp` will always return `true` if `a` and `b` are in the right order, otherwise it will return `false`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
type Nodelist struct { |
||||
Data int |
||||
Next *Nodelist |
||||
} |
||||
|
||||
func SortList (l *NodeList, cmp func(a,b int) bool) *NodeList{ |
||||
|
||||
} |
||||
``` |
||||
|
||||
- For example, the following function used as `cmp` will sort the list in ascending order : |
||||
|
||||
```go |
||||
func ascending(a, b int) bool{ |
||||
if a <= b { |
||||
return true |
||||
} else { |
||||
return false |
||||
} |
||||
} |
||||
``` |
@ -0,0 +1,27 @@
|
||||
## swapbits |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that takes a byte, swaps its halves (like the example) and |
||||
returns the result. |
||||
|
||||
Your function must be declared as follows: |
||||
|
||||
```go |
||||
func SwapBits(octet byte) byte { |
||||
|
||||
} |
||||
``` |
||||
|
||||
Example: |
||||
|
||||
1 byte |
||||
|
||||
--- |
||||
|
||||
``` |
||||
0100 | 0001 |
||||
\ / |
||||
/ \ |
||||
0001 | 0100 |
||||
``` |
@ -0,0 +1,22 @@
|
||||
## switchcase |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a string and reverses the case of all its letters. |
||||
|
||||
- Other characters remain unchanged. |
||||
|
||||
- You must display the result followed by a '\n'. |
||||
|
||||
- If the number of arguments is not 1, the program displays '\n'. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "SometHingS iS WronG" |
||||
sOMEThINGs Is wRONg |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,28 @@
|
||||
## union |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes two strings and displays, without doubles, the |
||||
characters that appear in either one of the strings. |
||||
|
||||
The display will be in the order characters appear in the command line, and |
||||
will be followed by a \n. |
||||
|
||||
If the number of arguments is not 2, the program displays \n. |
||||
|
||||
Example : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/union$ go build |
||||
student@ubuntu:~/student/union$ ./union zpadinton "paqefwtdjetyiytjneytjoeyjnejeyj" | cat -e |
||||
zpadintoqefwjy$ |
||||
student@ubuntu:~/student/union$ ./union ddf6vewg64f gtwthgdwthdwfteewhrtag6h4ffdhsd | cat -e |
||||
df6vewg4thras$ |
||||
student@ubuntu:~/student/union$ ./union "rien" "cette phrase ne cache rien" | cat -e |
||||
rienct phas$ |
||||
student@ubuntu:~/student/union$ ./union | cat -e |
||||
$ |
||||
student@ubuntu:~/student/union$ ./union "rien" | cat -e |
||||
$ |
||||
student@ubuntu:~/student/union$ |
||||
``` |
@ -0,0 +1,43 @@
|
||||
## join |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, Unmatch, that returns the element of the slice (arr) that does not have a correspondent pair. |
||||
|
||||
The function must have the next signature. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Unmatch(arr []int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func main() { |
||||
arr := []int{1, 2, 3, 1, 2, 3, 4} |
||||
unmatch := student.Unmatch(arr) |
||||
fmt.Println(unmatch) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/unmatch$ go build |
||||
student@ubuntu:~/student/unmatch$ ./unmatch |
||||
4 |
||||
student@ubuntu:~/student/unmatch$ |
||||
``` |
@ -0,0 +1,27 @@
|
||||
# wdmatch |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes two strings and checks whether it is possible to write the first string with characters from the second string, while respecting the order in which these characters appear in the second string. |
||||
|
||||
- If it is possible, the program displays the string followed by a `\n`, otherwise it simply displays a `\n`. |
||||
|
||||
- If the number of arguments is not 2, the program displays `\n`. |
||||
|
||||
Example of output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "faya" "fgvvfdxcacpolhyghbreda" |
||||
faya |
||||
student@ubuntu:~/piscine/test$ ./test "faya" "fgvvfdxcacpolhyghbred" |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test "error" rrerrrfiiljdfxjyuifrrvcoojh |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test "quarante deux" "qfqfsudf arzgsayns tsregfdgs sjytdekuoixq " |
||||
quarante deux |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,13 @@
|
||||
## ztail |
||||
|
||||
### Instructions |
||||
|
||||
Write a program called ztail that does the same thing as the system command tail, but witch takes at least one file as argument. |
||||
|
||||
The only option you have to handle is -c. This option will be used in all tests. |
||||
|
||||
For this program you can use the "os" package. |
||||
|
||||
For the program to pass the test you should follow the convention for the return code of program in Unix sistems (see os.Exit) |
||||
|
||||
For more information consult the man page for tail. |
Loading…
Reference in new issue