mirror of https://github.com/01-edu/public.git
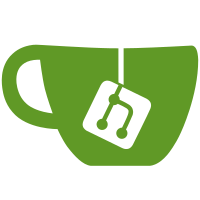
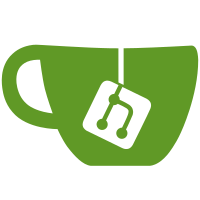
3 changed files with 150 additions and 108 deletions
@ -1,51 +1,76 @@
|
||||
test_empty_input() { |
||||
output=$(echo -n "" | ./solutions/joker-num.sh) |
||||
expected_output="Error: Input is empty, please try again." |
||||
diff <(echo "$output") <(echo "$expected_output") |
||||
} |
||||
#!/usr/bin/env bash |
||||
|
||||
test_not_a_number() { |
||||
output=$(echo "a" | ./solutions/joker-num.sh) |
||||
expected_output="Error: Input is not a number, please try again." |
||||
diff <(echo "$output") <(echo "$expected_output") |
||||
} |
||||
IFS=' |
||||
' |
||||
script_dirS=$(cd -P "$(dirname "$BASH_SOURCE")" &>/dev/null && pwd) |
||||
|
||||
test_number_out_of_range() { |
||||
output=$(echo "100001" | ./solutions/joker-num.sh) |
||||
expected_output="Error: Number out of range, please try again." |
||||
diff <(echo "$output") <(echo "$expected_output") |
||||
} |
||||
challenge() { |
||||
args=${@:1:$#-1} |
||||
input="${@: -1}" |
||||
|
||||
test_correct_guess() { |
||||
output=$(echo "50000" | ./solutions/joker-num.sh; echo "50000" | ./solutions/joker-num.sh) |
||||
expected_output="Congratulations! You guessed the number." |
||||
diff <(echo "$output") <(echo "$expected_output") |
||||
submitted=$( |
||||
./student/joker-num.sh $args <<EOF |
||||
$input |
||||
EOF |
||||
) |
||||
expected=$( |
||||
./solutions/joker-num.sh $args <<EOF |
||||
$input |
||||
EOF |
||||
) |
||||
diff <(echo "$submitted") <(echo "$expected") |
||||
} |
||||
|
||||
test_guess_too_low() { |
||||
output=$(echo "50000" | ./solutions/joker-num.sh; echo "49999" | ./solutions/joker-num.sh) |
||||
expected_output="Go up." |
||||
diff <(echo "$output") <(echo "$expected_output") |
||||
} |
||||
# Good input, win |
||||
input="1 |
||||
100 |
||||
49 |
||||
51 |
||||
50 |
||||
" |
||||
|
||||
test_guess_too_high() { |
||||
output=$(echo "50000" | ./solutions/joker-num.sh; echo "50001" | ./solutions/joker-num.sh) |
||||
expected_output="Go down." |
||||
diff <(echo "$output") <(echo "$expected_output") |
||||
} |
||||
test_player_one() { |
||||
test_empty_input |
||||
test_not_a_number |
||||
test_number_out_of_range |
||||
} |
||||
test_player_2() { |
||||
test_empty_input |
||||
test_not_a_number |
||||
test_number_out_of_range |
||||
test_correct_guess |
||||
test_guess_too_low |
||||
test_guess_too_high |
||||
} |
||||
# Good input, win |
||||
challenge 50 "1 |
||||
100 |
||||
49 |
||||
51 |
||||
50 |
||||
" |
||||
|
||||
# Good input, lose |
||||
challenge 50 "10 |
||||
20 |
||||
30 |
||||
40 |
||||
41 |
||||
42 |
||||
" |
||||
|
||||
# Bad arguments |
||||
challenge "10" |
||||
|
||||
# Bad arguments |
||||
challenge 0 "10" |
||||
|
||||
# Bad arguments |
||||
challenge 101 "10" |
||||
|
||||
# Bad arguments |
||||
challenge -20 "10" |
||||
|
||||
# Bad arguments |
||||
challenge aa "10" |
||||
|
||||
# Handle bad input |
||||
challenge 78 "10 |
||||
aa |
||||
|
||||
|
||||
test_player_1 |
||||
test_player_2 |
||||
3000 |
||||
-10 |
||||
0 |
||||
0 |
||||
40 |
||||
80 |
||||
79 |
||||
78" |
||||
|
@ -1,63 +1,49 @@
|
||||
#!/bin/bash |
||||
|
||||
# Loop for player one |
||||
for (( ; ; )) |
||||
do |
||||
echo "Player one, please enter a number between 1 and 100000 (inclusive) and press enter:" |
||||
read number |
||||
# timeout 1s read -s number |
||||
# read -p "Player one, please enter a number between 1 and 100000 (inclusive) and press enter:" -s number |
||||
# sleep 5s |
||||
# Check if input is empty |
||||
if [[ -z "$number" ]] |
||||
then |
||||
echo "Error: Input is empty, please try again." |
||||
if [[ $# != 1 |
||||
|| -z "$1" |
||||
|| ! "$1" =~ ^[0-9]+$ |
||||
|| "$1" -lt 1 |
||||
|| "$1" -gt 100 ]] |
||||
then |
||||
echo "Error: wrong argument" |
||||
exit 1 |
||||
fi |
||||
|
||||
# Check if input is a number |
||||
elif ! [[ "$number" =~ ^[0-9]+$ ]] |
||||
then |
||||
echo "Error: Input is not a number, please try again." |
||||
|
||||
# Check if input is between 1 and 100000 (inclusive) |
||||
elif [[ "$number" -lt 1 || "$number" -gt 100000 ]] |
||||
then |
||||
echo "Error: Number out of range, please try again." |
||||
|
||||
else |
||||
break |
||||
fi |
||||
done |
||||
number=$1 |
||||
|
||||
# Start the for loop for player two |
||||
for (( ; ; )) |
||||
for (( tries_left=5 ; tries_left > 0; tries_left-- )) |
||||
do |
||||
echo "Player two, please enter your guess:" |
||||
echo "Enter your guess ($tries_left tries left):" |
||||
|
||||
read guess |
||||
if [[ -z "$guess" ]] |
||||
if [[ $? < 0 ]] |
||||
then |
||||
echo "Error: Input is empty" |
||||
continue |
||||
exit 1 |
||||
fi |
||||
|
||||
# Check if input is a number |
||||
if ! [[ "$guess" =~ ^[0-9]+$ ]] |
||||
if [[ -z "$guess" |
||||
|| ! "$guess" =~ ^[0-9]+$ |
||||
|| "$guess" -lt 1 |
||||
|| "$guess" -gt 100 ]] |
||||
then |
||||
echo "Error: Input is not a number" |
||||
tries_left=$tries_left+1 |
||||
continue |
||||
fi |
||||
|
||||
# Check if guess is correct |
||||
if [[ "$guess" -eq "$number" ]] |
||||
then |
||||
echo "Congratulations! You guessed the number." |
||||
break |
||||
echo "Congratulations, you found the number in $((5-$tries_left+1)) moves!" |
||||
exit |
||||
fi |
||||
|
||||
# Check if guess is too low or too high |
||||
if [[ "$guess" -lt "$number" ]] |
||||
then |
||||
echo "Go up." |
||||
echo "Go up" |
||||
else |
||||
echo "Go down." |
||||
echo "Go down" |
||||
fi |
||||
done |
||||
|
||||
echo "You lost, the number was $number" |
||||
|
Loading…
Reference in new issue