mirror of https://github.com/01-edu/public.git
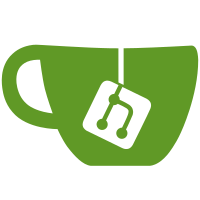
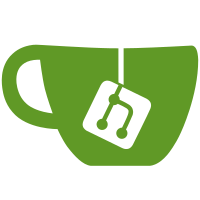
10 changed files with 19 additions and 320 deletions
@ -1,37 +0,0 @@
|
||||
## capitalize |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that capitalizes the first letter of each word **and** lowercases the rest. |
||||
|
||||
A word is a sequence of **alphanumeric** characters. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Capitalize(s string) string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(Capitalize("Hello! How are you? How+are+things+4you?")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
Hello! How Are You? How+Are+Things+4you? |
||||
$ |
||||
``` |
@ -1,7 +0,0 @@
|
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(Capitalize("Hello! How are you? How+are+things+4you?")) |
||||
} |
@ -1,48 +0,0 @@
|
||||
## foreach |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ForEach` that, for an `int` slice, applies a function on each element of that slice. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ForEach(f func(int), a []int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func PrintNbr(n int) { |
||||
fmt.Print(n) |
||||
} |
||||
|
||||
func main() { |
||||
|
||||
a := []int{1, 2, 3, 4, 5, 6} |
||||
ForEach(PrintNbr, a) |
||||
fmt.Println() |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
123456 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [Function literals](https://golang.org/ref/spec#Function_literals) |
||||
- [Function declaration](https://golang.org/ref/spec#Function_declarations) |
||||
- [Function types](https://golang.org/ref/spec#Function_types) |
@ -1,62 +0,0 @@
|
||||
## listsize |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ListSize` that returns the number of elements in a linked list `l`. |
||||
|
||||
### Expected function and structure |
||||
|
||||
```go |
||||
type NodeL struct { |
||||
Data interface{} |
||||
Next *NodeL |
||||
} |
||||
|
||||
type List struct { |
||||
Head *NodeL |
||||
Tail *NodeL |
||||
} |
||||
|
||||
func ListSize(l *List) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func ListPushFront(l *List, data interface{}) { |
||||
n := &NodeL{Data: data} |
||||
if l.Head == nil { |
||||
l.Head = n |
||||
l.Tail = n |
||||
} else { |
||||
l.Head, n.Next = n, l.Head |
||||
} |
||||
} |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
|
||||
ListPushFront(link, "Hello") |
||||
ListPushFront(link, "2") |
||||
ListPushFront(link, "you") |
||||
ListPushFront(link, "man") |
||||
|
||||
fmt.Println(ListSize(link)) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
4 |
||||
$ |
||||
``` |
@ -0,0 +1,17 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
link := &piscine.List{} |
||||
|
||||
piscine.ListPushFront(link, "Hello") |
||||
piscine.ListPushFront(link, "2") |
||||
piscine.ListPushFront(link, "you") |
||||
piscine.ListPushFront(link, "man") |
||||
|
||||
fmt.Println(piscine.ListSize(link)) |
||||
} |
@ -1,46 +0,0 @@
|
||||
## nrune |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the nth `rune` of a `string`. If not possible, it returns `0`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func NRune(s string, n int) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
z01.PrintRune(NRune("Hello!", 3)) |
||||
z01.PrintRune(NRune("Salut!", 2)) |
||||
z01.PrintRune(NRune("Bye!", -1)) |
||||
z01.PrintRune(NRune("Bye!", 5)) |
||||
z01.PrintRune(NRune("Ola!", 4)) |
||||
z01.PrintRune('\n') |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
la! |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [rune-literals](https://golang.org/ref/spec#Rune_literals) |
@ -1,36 +0,0 @@
|
||||
## printstr |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that prints one by one the characters of a `string` on the screen. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func PrintStr(s string) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Hints |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
PrintStr("Hello World!") |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
go run . | cat -e |
||||
Hello World! |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [01-edu/z01](https://github.com/01-edu/z01) |
@ -1,39 +0,0 @@
|
||||
## strrev |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that reverses a `string`. |
||||
|
||||
- This function will **return** the reversed `string`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func StrRev(s string) string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
s := "Hello World!" |
||||
s = StrRev(s) |
||||
fmt.Println(s) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
!dlroW olleH |
||||
$ |
||||
``` |
@ -1,44 +0,0 @@
|
||||
## swap |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that takes two **pointers to an `int`** (`*int`) and swaps their contents. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Swap(a *int, b *int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
a := 0 |
||||
b := 1 |
||||
Swap(&a, &b) |
||||
fmt.Println(a) |
||||
fmt.Println(b) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
1 |
||||
0 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [Pointers](https://golang.org/ref/spec#Pointer_types) |
Loading…
Reference in new issue