mirror of https://github.com/01-edu/public.git
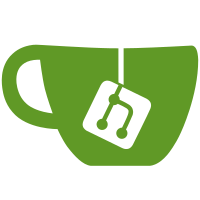
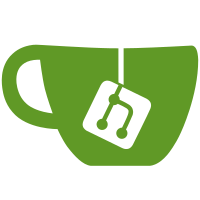
5 changed files with 323 additions and 0 deletions
@ -0,0 +1,73 @@ |
|||||||
|
## DifferenceBetweenDate |
||||||
|
|
||||||
|
### Instructions |
||||||
|
|
||||||
|
Create a file `DifferenceBetweenDate.java`. |
||||||
|
|
||||||
|
Write a function `durationBetweenTime` that returns the duration between the times as parameter. Must always be positive. |
||||||
|
Write a function `periodBetweenDate` that returns the period between the dates as parameter.Must always be positive. |
||||||
|
Write a function `numberOfHoursBetweenDateTime` that returns the number of hours between the date times as parameter. Must always be positive. |
||||||
|
|
||||||
|
### Expected Functions |
||||||
|
|
||||||
|
```java |
||||||
|
import java.time.Duration; |
||||||
|
import java.time.LocalDate; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.LocalTime; |
||||||
|
import java.time.Period; |
||||||
|
|
||||||
|
public class DifferenceBetweenDate { |
||||||
|
|
||||||
|
public static Duration durationBetweenTime(LocalTime localTime1, LocalTime localTime2) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static Period periodBetweenDate(LocalDate date1, LocalDate date2) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static Long numberOfHoursBetweenDateTime(LocalDateTime dateTime1, LocalDateTime dateTime2) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
### Usage |
||||||
|
|
||||||
|
Here is a possible ExerciseRunner.java to test your function : |
||||||
|
|
||||||
|
```java |
||||||
|
import java.time.LocalDate; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.LocalTime; |
||||||
|
|
||||||
|
public class ExerciseRunner { |
||||||
|
|
||||||
|
public static void main(String[] args) { |
||||||
|
Duration duration = DifferenceBetweenDate.durationBetweenTime(LocalTime.of(12, 54, 32), LocalTime.of(21, 23, 53)); |
||||||
|
System.out.println(duration.toHoursPart() + "H" + duration.toMinutesPart() + "M" + duration.toSecondsPart() + "S"); |
||||||
|
Period period = DifferenceBetweenDate.periodBetweenDate(LocalDate.of(2020, 10, 13), LocalDate.of(2022, 5, 8)); |
||||||
|
System.out.println(period.getYears() + "Y" + period.getMonths() + "M" + period.getDays() + "D"); |
||||||
|
System.out.println(DifferenceBetweenDate.numberOfHoursBetweenDateTime(LocalDateTime.of(2022, 4, 12, 16, 18, 56), LocalDateTime.of(2022, 5, 10, 21, 54, 56))); |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
and its output : |
||||||
|
```shell |
||||||
|
$ javac *.java -d build |
||||||
|
$ java -cp build ExerciseRunner |
||||||
|
8H29M21S |
||||||
|
1Y6M25D |
||||||
|
677 |
||||||
|
$ |
||||||
|
``` |
||||||
|
|
||||||
|
### Notions |
||||||
|
[LocalDate](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalDate.html) |
||||||
|
[LocalTime](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalTime.html) |
||||||
|
[LocalDateTime](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalDateTime.html) |
||||||
|
[DateTimeFormatter](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/format/DateTimeFormatter.html) |
||||||
|
[Locale](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Locale.html) |
@ -0,0 +1,69 @@ |
|||||||
|
## FormatDate |
||||||
|
|
||||||
|
### Instructions |
||||||
|
|
||||||
|
Create a file `FormatDate.java`. |
||||||
|
|
||||||
|
Write a function `formatToFullText` that returns a formatted string using the date as parameter. The awaited format is `Le 22 aoû. de l'an 2021 à 13h25m et 46s` |
||||||
|
Write a function `formatSimple` that returns a formatted string using the date as parameter. The awaited format is `febbraio 13 22` |
||||||
|
Write a function `formatIso` that returns a formatted string using the time as parameter. The awaited format is `16:18:56.8495847` |
||||||
|
|
||||||
|
### Expected Functions |
||||||
|
|
||||||
|
```java |
||||||
|
import java.time.LocalDate; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.LocalTime; |
||||||
|
|
||||||
|
public class FormatDate { |
||||||
|
|
||||||
|
public static String formatToFullText(LocalDateTime dateTime) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static String formatSimple(LocalDate date) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static String formatIso(LocalTime time) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
### Usage |
||||||
|
|
||||||
|
Here is a possible ExerciseRunner.java to test your function : |
||||||
|
|
||||||
|
```java |
||||||
|
import java.time.LocalDate; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.LocalTime; |
||||||
|
|
||||||
|
public class ExerciseRunner { |
||||||
|
|
||||||
|
public static void main(String[] args) { |
||||||
|
System.out.println(FormatDate.formatToFullText(LocalDateTime.of(2021, 8, 22, 13, 25, 46))); |
||||||
|
System.out.println(FormatDate.formatSimple(LocalDate.of(2022, 2, 13))); |
||||||
|
System.out.println(FormatDate.formatIso(LocalTime.of(16, 18, 56, 8495847))); |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
and its output : |
||||||
|
```shell |
||||||
|
$ javac *.java -d build |
||||||
|
$ java -cp build ExerciseRunner |
||||||
|
Le 22 août de l'an 2021 à 13h25m et 46s |
||||||
|
febbraio 13 22 |
||||||
|
16:18:56.008495847 |
||||||
|
$ |
||||||
|
``` |
||||||
|
|
||||||
|
### Notions |
||||||
|
[LocalDate](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalDate.html) |
||||||
|
[LocalTime](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalTime.html) |
||||||
|
[LocalDateTime](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalDateTime.html) |
||||||
|
[DateTimeFormatter](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/format/DateTimeFormatter.html) |
||||||
|
[Locale](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Locale.html) |
@ -0,0 +1,63 @@ |
|||||||
|
## ParseDate |
||||||
|
|
||||||
|
### Instructions |
||||||
|
|
||||||
|
Create a file `ParseDate.java`. |
||||||
|
|
||||||
|
Write a function `parseIsoFormat` that returns a date object using the string as parameter. The date as a parameter is in ISO format (`2022-04-25T20:51:28.709039322`) |
||||||
|
Write a function `parseFullTextFormat` that returns a date object using the string as parameter. The date as a parameter use a text format (`lundi 25 avril 2022`) |
||||||
|
Write a function `parseTimeFormat` that returns a time object using the string as parameter. The date as a parameter use a text format (`09 heures du soir, 07 minutes et 23 secondes`) |
||||||
|
|
||||||
|
### Expected Functions |
||||||
|
|
||||||
|
```java |
||||||
|
import java.time.LocalDate; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.LocalTime; |
||||||
|
|
||||||
|
public class ParseDate { |
||||||
|
|
||||||
|
public static LocalDateTime parseIsoFormat(String stringDate) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static LocalDate parseFullTextFormat(String stringDate) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static LocalTime parseTimeFormat(String stringDate) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
### Usage |
||||||
|
|
||||||
|
Here is a possible ExerciseRunner.java to test your function : |
||||||
|
```java |
||||||
|
public class ExerciseRunner { |
||||||
|
public static void main(String[] args) { |
||||||
|
System.out.println(ParseDate.parseIsoFormat("2022-04-25T20:51:28.709039322")); |
||||||
|
System.out.println(ParseDate.parseFullTextFormat("lundi 25 avril 2022")); |
||||||
|
System.out.println(ParseDate.parseTimeFormat("09 heures du soir, 07 minutes et 23 secondes")); |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
and its output : |
||||||
|
```shell |
||||||
|
$ javac *.java -d build |
||||||
|
$ java -cp build ExerciseRunner |
||||||
|
2022-04-25T20:51:28.709039322 |
||||||
|
2022-04-25 |
||||||
|
21:07:23 |
||||||
|
$ |
||||||
|
``` |
||||||
|
|
||||||
|
### Notions |
||||||
|
[LocalDate](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalDate.html) |
||||||
|
[LocalTime](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalTime.html) |
||||||
|
[LocalDateTime](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/LocalDateTime.html) |
||||||
|
[DateTimeFormatter](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/format/DateTimeFormatter.html) |
||||||
|
[Locale](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Locale.html) |
@ -0,0 +1,63 @@ |
|||||||
|
## RegexMatch |
||||||
|
|
||||||
|
### Instructions |
||||||
|
|
||||||
|
Create a file `RegexMatch.java`. |
||||||
|
|
||||||
|
Write a function `containsOnlyAlpha` that returns `true` if the string as parameter contains only alpha characters. |
||||||
|
Write a function `startWithLetterAndEndWithNumber` that returns `true` if the string as parameter starts with one letter and ends with one number. |
||||||
|
Write a function `containsAtLeast3SuccessiveA` that returns `true` if the string as parameter contains at least 3 successive A. |
||||||
|
|
||||||
|
### Expected Functions |
||||||
|
```java |
||||||
|
public class RegexMatch { |
||||||
|
public static boolean containsOnlyAlpha(String s) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static boolean startWithLetterAndEndWithNumber(String s) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static boolean containsAtLeast3SuccessiveA(String s) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
### Usage |
||||||
|
|
||||||
|
Here is a possible ExerciseRunner.java to test your function |
||||||
|
: |
||||||
|
|
||||||
|
```java |
||||||
|
import java.io.IOException; |
||||||
|
|
||||||
|
public class ExerciseRunner { |
||||||
|
|
||||||
|
public static void main(String[] args) throws IOException { |
||||||
|
System.out.println(RegexReplace.containsOnlyAlpha("azejkdfhjsdf")); |
||||||
|
System.out.println(RegexReplace.containsOnlyAlpha("azejkd fhjsdf")); |
||||||
|
System.out.println(RegexReplace.startWithLetterAndEndWithNumber("asjd jd34jds jkfd6f5")); |
||||||
|
System.out.println(RegexReplace.startWithLetterAndEndWithNumber("asjd jd34jds jkfd6.")); |
||||||
|
System.out.println(RegexReplace.containsAtLeast3SuccessiveA("sdjkAAAAAsdjksj")); |
||||||
|
System.out.println(RegexReplace.containsAtLeast3SuccessiveA("sdjkAAsdaaasdjksj")); |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
and its output : |
||||||
|
```shell |
||||||
|
$ javac *.java -d build |
||||||
|
$ java -cp build ExerciseRunner |
||||||
|
true |
||||||
|
false |
||||||
|
true |
||||||
|
false |
||||||
|
true |
||||||
|
false |
||||||
|
$ |
||||||
|
``` |
||||||
|
|
||||||
|
### Notions |
||||||
|
[Pattern](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/regex/Pattern.html) |
@ -0,0 +1,55 @@ |
|||||||
|
## RegexReplace |
||||||
|
|
||||||
|
### Instructions |
||||||
|
|
||||||
|
Create a file `RegexReplace.java`. |
||||||
|
|
||||||
|
Write a function `removeUnits` that returns the string where the units `cm` and `€` is removed if it followed directly a number and followed by a space. |
||||||
|
Write a function `removeFeminineAndPlural` that returns the string where the mark of feminine and plural is removed from word : |
||||||
|
- if a word ends a mark of plural (with s or x), remove it. |
||||||
|
- if it ends with an e or if the e is followed by the mark of plural, remove the it |
||||||
|
- if it ends with `le` following `el` (or if `le` is followed by plural), remove it. |
||||||
|
|
||||||
|
### Expected Functions |
||||||
|
```java |
||||||
|
public class RegexReplace { |
||||||
|
public static String removeUnits(String s) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
|
||||||
|
public static String removeFeminineAndPlural(String s) { |
||||||
|
// your code here |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
### Usage |
||||||
|
|
||||||
|
Here is a possible ExerciseRunner.java to test your function |
||||||
|
: |
||||||
|
```java |
||||||
|
import java.io.IOException; |
||||||
|
|
||||||
|
public class ExerciseRunner { |
||||||
|
public static void main(String[] args) throws IOException { |
||||||
|
System.out.println(RegexReplace.removeUnits("32cm et 50€")); |
||||||
|
System.out.println(RegexReplace.removeUnits("32 cm et 50 €")); |
||||||
|
System.out.println(RegexReplace.removeUnits("32cms et 50€!")); |
||||||
|
System.out.println(RegexReplace.removeFeminineAndPlural("le lapin joue à la belle balle avec des animaux rigolos pour gagner les billes bleues")); |
||||||
|
} |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
and its output : |
||||||
|
```shell |
||||||
|
$ javac *.java -d build |
||||||
|
$ java -cp build ExerciseRunner |
||||||
|
32 et 50 |
||||||
|
32 cm et 50 € |
||||||
|
32cms et 50€! |
||||||
|
l lapin jou à la bel ball avec d animau rigolo pour gagner l bill bleu |
||||||
|
$ |
||||||
|
``` |
||||||
|
|
||||||
|
### Notions |
||||||
|
[Pattern](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/regex/Pattern.html) |
Loading…
Reference in new issue