mirror of https://github.com/01-edu/public.git
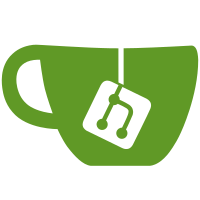
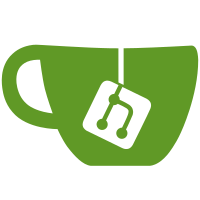
2 changed files with 35 additions and 43 deletions
@ -1,43 +0,0 @@
|
||||
# count-character |
||||
|
||||
### Instructions |
||||
write a function that takes a string and a character as arguments and returns the number of times the character appears in the string. |
||||
- if the character is not in the string return 0 |
||||
- if the string is empty return 0 |
||||
|
||||
### Expected Function |
||||
|
||||
```go |
||||
func CountChar(str string, c rune) int { |
||||
... |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.CountChar("Hello World", 'l')) |
||||
fmt.Println(piscine.CountChar("5 balloons",5)) |
||||
fmt.Println(piscine.CountChar(" ", ' ')) |
||||
fmt.Println(piscine.CountChar("The 7 deadly sins", '7')) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
3 |
||||
0 |
||||
1 |
||||
1 |
||||
``` |
||||
|
@ -0,0 +1,35 @@
|
||||
# getascii |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a character as an argument, and displays the correspondent ASCII code followed by a newline. |
||||
|
||||
- If the input is more than one character, return a newline `\n`. |
||||
- If the number of arguments is less than 1, return a newline `\n`. |
||||
- If the number of arguments is more than 1, return a newline `\n`. |
||||
- If the the input is a non ascii character, return a newline `\n`. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
console |
||||
$ go run . "W" | cat -e |
||||
87$ |
||||
$ |
||||
$ go run . "W" "Q" "T" | cat -e |
||||
$ |
||||
$ |
||||
$ go run . "^" | cat -e |
||||
94$ |
||||
$ |
||||
$ go run . 7 | cat -e |
||||
55$ |
||||
$ |
||||
$ go run . a | cat -e |
||||
97$ |
||||
$ |
||||
$ go run . "Stay Home" | cat -e |
||||
$ |
||||
$ go run . |
||||
$ |
||||
``` |
Loading…
Reference in new issue