mirror of https://github.com/01-edu/public.git
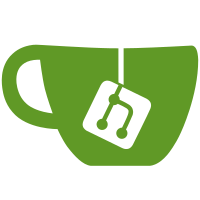
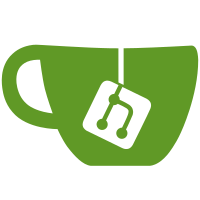
14 changed files with 834 additions and 0 deletions
@ -0,0 +1,54 @@
|
||||
## KeepTheChange |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `KeepTheChange.java`. |
||||
|
||||
Write a function `computeChange` that returns the list of coins that compose the change. |
||||
As parameters, we have : |
||||
* the amount to decompose in different coins |
||||
* the set of existing coins |
||||
|
||||
The awaiting solution is the best solution, so must have the minimum of coins. |
||||
The tests are choosen to have an unique solution. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.List; |
||||
import java.util.Set; |
||||
|
||||
public class KeepTheChange { |
||||
public static List<Integer> computeChange(int amount, Set<Integer> coins) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.Set; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
System.out.println(KeepTheChange.computeChange(18, Set.of(1, 3, 7))); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
[7, 7, 3, 1] |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[List](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
||||
[Set](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Set.html) |
||||
[Change computing algorithm](https://tryalgo.org/fr/2016/12/11/rendudemonnaie) |
@ -0,0 +1,49 @@
|
||||
## ListContains |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `ListContains.java`. |
||||
|
||||
Write a function `containsValue` that returns true if the list as parameter contains the element as parameter, false otherwise. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ListContains { |
||||
public static boolean containsValue(List<Integer> list, Integer value) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
System.out.println(ListContains.containsValue(List.of(9, 13, 8, 23, 1, 0, 89), 8)); |
||||
System.out.println(ListContains.containsValue(List.of(9, 13, 8, 23, 1, 0, 89), 10)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[List](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
@ -0,0 +1,48 @@
|
||||
## ListEqual |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `ListEqual.java`. |
||||
|
||||
Write a function `areListEquals` that returns true if the lists as parameters are equals. Returns false otherwise. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ListEqual { |
||||
public static boolean areListEquals(List<String> list1, List<String> list2) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(ListEqual.areListEquals(List.of("Alice", "Bob", "Charly", "Emily"), List.of("Alice", "Bob", "Charly", "Emily"))); |
||||
System.out.println(ListEqual.areListEquals(List.of("Alice", "Bob", "Charly", "Emily"), List.of("Alice", "Bob", "Emily", "Charly"))); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[List](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
@ -0,0 +1,55 @@
|
||||
## ListSearchIndex |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `ListSearchIndex.java`. |
||||
|
||||
Write a function `findLastIndex` that returns the last index of an element in a list. Returns null otherwise. |
||||
Write a function `findFirstIndex` that returns the first index of an element in a list. Returns null otherwise. |
||||
Write a function `findAllIndexes` that returns a list of all indexes of an element in a list. Returns an empty list otherwise. |
||||
|
||||
### Expected Functions |
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ListSearchIndex { |
||||
public static Integer findLastIndex(List<Integer> list, Integer value) { |
||||
// your code here |
||||
} |
||||
public static Integer findFirstIndex(List<Integer> list, Integer value) { |
||||
// your code here |
||||
} |
||||
public static List<Integer> findAllIndexes(List<Integer> list, Integer value) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(ListSearchIndex.findLastIndex(List.of(9, 13, 89, 8, 23, 1, 0, 89), 89)); |
||||
System.out.println(ListSearchIndex.findFirstIndex(List.of(9, 13, 89, 8, 23, 1, 0, 89), 89)); |
||||
System.out.println(ListSearchIndex.findAllIndexes(List.of(9, 13, 89, 8, 23, 1, 0, 89), 89).toString()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
7 |
||||
2 |
||||
[2, 7] |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[List](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
||||
[ArrayList](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/ArrayList.html) |
@ -0,0 +1,53 @@
|
||||
## MapEqual |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `MapEqual.java`. |
||||
|
||||
Write a function `areMapEquals` that returns `true` if the maps as parameters are equal. Returns `false` otherwise. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.Map; |
||||
|
||||
public class MapEqual { |
||||
public static boolean areMapEquals(Map<String, Integer> map1, Map<String, Integer> map2) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function: |
||||
|
||||
```java |
||||
import java.util.Map; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
Map<String, Integer> map1 = Map.of("Alice", 1, "Bob", 2, "Charly", 3); |
||||
Map<String, Integer> map2 = Map.of("Alice", 1, "Bob", 2, "Charly", 3); |
||||
System.out.println(MapEqual.areMapEquals(map1, map2)); // Expected Output: true |
||||
|
||||
Map<String, Integer> map3 = Map.of("Alice", 1, "Bob", 2, "Charly", 3); |
||||
Map<String, Integer> map4 = Map.of("Alice", 1, "Bob", 2, "Emily", 3); |
||||
System.out.println(MapEqual.areMapEquals(map3, map4)); // Expected Output: false |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Map](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Map.html) |
@ -0,0 +1,85 @@
|
||||
## MapContains |
||||
|
||||
You have been assigned the task of implementing a product inventory using Java maps. Each product has a unique ID as the key and the corresponding product details as the value. |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `MapInventory.java`. |
||||
|
||||
Write two functions: |
||||
|
||||
Write a function `getProductPrice` that takes a map of product IDs and their corresponding prices, along with a product ID as parameters, and returns the price of the specified product. If the product ID is not present in the map, the function should return `-1`. |
||||
|
||||
Write a function `getProductIdsByPrice` that takes a map of product IDs and their corresponding prices, along with a price as a parameter, and returns a list of product IDs that have the given price. If no products are found with the specified price, the function should return an empty list. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.ArrayList; |
||||
import java.util.List; |
||||
import java.util.Map; |
||||
|
||||
public class MapInventory { |
||||
public static int getProductPrice(Map<String, Integer> inventory, String productId) { |
||||
// your code here |
||||
} |
||||
|
||||
public static List<String> getProductIdsByPrice(Map<String, Integer> inventory, int price) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your functions: |
||||
|
||||
```java |
||||
import java.util.HashMap; |
||||
import java.util.List; |
||||
import java.util.Map; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Map<String, Integer> inventory = new HashMap<>(); |
||||
inventory.put("P001", 100); |
||||
inventory.put("P002", 50); |
||||
inventory.put("P003", 75); |
||||
inventory.put("P004", 50); |
||||
inventory.put("P005", 75); |
||||
|
||||
System.out.println(MapInventory.getProductPrice(inventory, "P002")); // Output: 50 |
||||
System.out.println(MapInventory.getProductPrice(inventory, "P004")); // Output: 50 |
||||
System.out.println(MapInventory.getProductPrice(inventory, "P006")); // Output: -1 |
||||
|
||||
List<String> productsWithPrice50 = MapInventory.getProductIdsByPrice(inventory, 50); |
||||
System.out.println(productsWithPrice50); // Output: [P002, P004] |
||||
|
||||
List<String> productsWithPrice75 = MapInventory.getProductIdsByPrice(inventory, 75); |
||||
System.out.println(productsWithPrice75); // Output: [P003, P005] |
||||
|
||||
List<String> productsWithPrice80 = MapInventory.getProductIdsByPrice(inventory, 80); |
||||
System.out.println(productsWithPrice80); // Output: [] |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
50 |
||||
50 |
||||
-1 |
||||
[P002, P004] |
||||
[P003, P005] |
||||
[] |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Map](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Map.html) |
||||
[List](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
@ -0,0 +1,72 @@
|
||||
## SetEqual |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `SetEqual.java`. |
||||
|
||||
Write a function `areSetEquals` that returns `true` if the sets as parameters are equal. Returns `false` otherwise. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.Set; |
||||
|
||||
public class SetEqual { |
||||
public static boolean areSetEquals(Set<String> set1, Set<String> set2) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function: |
||||
|
||||
```java |
||||
import java.util.HashSet; |
||||
import java.util.Set; |
||||
import java.util.TreeSet; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Set<String> set1 = new TreeSet<>(); |
||||
set1.add("Alice"); |
||||
set1.add("Bob"); |
||||
set1.add("Charly"); |
||||
|
||||
Set<String> set2 = new TreeSet<>(); |
||||
set2.add("Bob"); |
||||
set2.add("Charly"); |
||||
set2.add("Alice"); |
||||
|
||||
System.out.println(SetEqual.areSetEquals(set1, set2)); // Expected Output: true |
||||
|
||||
Set<String> set3 = new HashSet<>(); |
||||
set3.add("Alice"); |
||||
set3.add("Bob"); |
||||
set3.add("Charly"); |
||||
|
||||
Set<String> set4 = new HashSet<>(); |
||||
set4.add("Alice"); |
||||
set4.add("Bob"); |
||||
set4.add("Emily"); |
||||
|
||||
System.out.println(SetEqual.areSetEquals(set3, set4)); // Expected Output: false |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
false |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Set](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
@ -0,0 +1,73 @@
|
||||
## SetContains |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `SetOperations.java`. |
||||
|
||||
Write two functions: |
||||
|
||||
Write a function `union` that takes two sets of integers as parameters and returns a new set that contains all the distinct elements from both sets. |
||||
|
||||
Write a function `intersection` that Takes two sets of integers as parameters and returns a new set that contains the common elements present in both sets. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.HashSet; |
||||
import java.util.Set; |
||||
|
||||
public class SetOperations { |
||||
public static Set<Integer> union(Set<Integer> set1, Set<Integer> set2) { |
||||
// your code here |
||||
} |
||||
|
||||
public static Set<Integer> intersection(Set<Integer> set1, Set<Integer> set2) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your functions: |
||||
|
||||
```java |
||||
import java.util.HashSet; |
||||
import java.util.Set; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
Set<Integer> set1 = new HashSet<>(); |
||||
set1.add(1); |
||||
set1.add(2); |
||||
set1.add(3); |
||||
|
||||
Set<Integer> set2 = new HashSet<>(); |
||||
set2.add(2); |
||||
set2.add(3); |
||||
set2.add(4); |
||||
|
||||
Set<Integer> unionSet = SetOperations.union(set1, set2); |
||||
System.out.println(unionSet); // Expected Output: [1, 2, 3, 4] |
||||
|
||||
Set<Integer> intersectionSet = SetOperations.intersection(set1, set2); |
||||
System.out.println(intersectionSet); // Expected Output: [2, 3] |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Le 22 août de l'an 2021 à 13h25m et 46s |
||||
[1, 2, 3, 4] |
||||
[2, 3] |
||||
$ |
||||
``` |
||||
|
||||
### Notion |
||||
|
||||
[Set](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
@ -0,0 +1,53 @@
|
||||
## SortList |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `Sort.java`. |
||||
|
||||
Write a function `sort` that returns the ascending sorted list as parameter. |
||||
Write a function `sortReverse` that returns the descending sorted list as parameter. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class Sort { |
||||
|
||||
public static List<Integer> sort(List<Integer> list) { |
||||
// your code here |
||||
} |
||||
|
||||
public static List<Integer> sortReverse(List<Integer> list) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.List; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
System.out.println(Sort.sort(List.of(15, 1, 14, 18, 14, 98, 54, -1, 12)).toString()); |
||||
System.out.println(Sort.sortReverse(List.of(15, 1, 14, 18, 14, 98, 54, -1, 12)).toString()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
[-1, 1, 12, 14, 14, 15, 18, 54, 98] |
||||
[98, 54, 18, 15, 14, 14, 12, 1, -1] |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[List](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
@ -0,0 +1,50 @@
|
||||
## Wedding |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `Wedding.java`. |
||||
|
||||
Write a function `createCouple` that returns a map of name which associates randomly a name from the first list to a name of the second list. |
||||
If the lists have different size, some names from the bigger list will not be ignored. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.Set; |
||||
import java.util.Map; |
||||
|
||||
public class Wedding { |
||||
public static Map<String, String> createCouple(Set<String> first, Set<String> second) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.Set; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
System.out.println(Wedding.createCouple(Set.of("Pikachu", "Dracaufeu", "Tortank"), Set.of("Legolas", "Aragorn", "Gimli"))); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
{Pikachu=Legolas, Tortank=Gimli, Dracaufeu=Aragorn} |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Set](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/List.html) |
||||
[Map](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Map.html) |
@ -0,0 +1,72 @@
|
||||
## WeddingComplex |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `WeddingComplex.java`. |
||||
|
||||
Write a function `createBestCouple` that returns a map of name from the first map associated with a name from the second list. |
||||
Each argument will be composed as follow : |
||||
* The name of the member to marry |
||||
* The ordered list of preference of member of the second list. |
||||
|
||||
To objective of this function is to determine the best couple possible. |
||||
The best solution is when for a given member (will be called Alice) : If there is a preferred partner (will be called Bob) than the chosen partner (will be called Charly), Bob is happier with its chosen partner (called Daphnee) than with Alice. |
||||
|
||||
> With an example : |
||||
> * First list : |
||||
> * Naruto orders his preferences : Sakura > Hinata |
||||
> * Sasuke orders his preferences : Sakura > Hinata |
||||
> * Second list : |
||||
> * Sakura orders her preferences : Sasuke > Naruto |
||||
> * Hinata orders her preferences : Naruto > Sasuke |
||||
> |
||||
> A correct solution for this example is : |
||||
> * Sasuke <-> Sakura (they are both with their first choice) |
||||
> * Naruto <-> Hinata (Naruto would prefere Sakura, but Sakura is happier with Sasuke, Hinata has her first choice) |
||||
|
||||
All the test will be chosen to guarantee such a solution exists. More than one solution could exist. |
||||
|
||||
In order to simplify, the list will always have the same size. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.util.List; |
||||
import java.util.Map; |
||||
|
||||
public class WeddingComplex { |
||||
public static Map<String, String> createBestCouple(Map<String, List<String>> first, Map<String, List<String>> second) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.List; |
||||
import java.util.Map; |
||||
|
||||
public class ExerciseRunner { |
||||
|
||||
public static void main(String[] args) { |
||||
System.out.println(WeddingComplex.createBestCouple( |
||||
Map.of("Naruto", List.of("Sakura", "Hinata"), "Sasuke", List.of("Sakura", "Hinata")), |
||||
Map.of("Sakura", List.of("Sasuke", "Naruto"), "Hinata", List.of("Naruto", "Sasuke")))); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
{Sasuke=Sakura, Naruto=Hinata} |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[Wedding Algorithm](https://fr.wikipedia.org/wiki/Algorithme_de_Gale_et_Shapley#Pseudo-code) |
||||
[Map](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/Map.html) |
@ -0,0 +1,58 @@
|
||||
## StreamCollect |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StreamCollect.java`. |
||||
|
||||
Create a function `mapByFirstLetter` which maps the first letter (to upper case) to the String. |
||||
Create a function `getMaxByModulo4` which return a map associating the modulo 4 to the max of the integer mathching the modulo 4. |
||||
##Create a function `orderAndConcatWithSharp` which joins the alphabetically sorted Strings with a ' # ' between each. The result String starts with a '{' and ends with a '}'. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class StreamCollect { |
||||
public static Map<Object, List<String>> mapByFirstLetter(Stream<String> s) { |
||||
// your code here |
||||
} |
||||
|
||||
public static Map<Integer, Optional<Integer>> getMaxByModulo4(Stream<Integer> s) { |
||||
// your code here |
||||
} |
||||
|
||||
public static String orderAndConcatWithSharp(Stream<String> s) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.util.stream.Stream; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(StreamCollect.mapByFirstLetter(Stream.of("Bonjour", "le", "monde !", "bonsoir"))); |
||||
System.out.println(StreamCollect.getMaxByModulo4(Stream.of(5, 12, 32, 4, 9, 17, 98, 424, 97, 5843, 48354))); |
||||
System.out.println(StreamCollect.orderAndConcatWithSharp(Stream.of("Hello", "how are you ?", "where do you live ?", "Bordeaux"))); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
{B=[Bonjour, bonsoir], L=[le], M=[monde !]} |
||||
{0=Optional[424], 1=Optional[97], 2=Optional[48354], 3=Optional[5843]} |
||||
##{Bordeaux # Hello # how are you ? # where do you live ?} |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Stream](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/stream/Stream.html) |
@ -0,0 +1,57 @@
|
||||
## StreamMap |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StreamMap.java`. |
||||
|
||||
Create a function `sumOfStringLength` which returns the sum of the length of the strings in the stream. |
||||
Create e function `upperCaseAllString` which returns the list of all the strings in upper case as stream. |
||||
Create e function `uniqIntValuesGreaterThan42` which keep only double greater than 42, transforms the double in integer and return them as a set. |
||||
|
||||
|
||||
### Expected Functions |
||||
```java |
||||
public class StreamMap { |
||||
public static Integer sumOfStringLength(Stream<String> s) { |
||||
// your code here |
||||
} |
||||
|
||||
public static List<String> upperCaseAllString(Stream<String> s) { |
||||
// your code here |
||||
} |
||||
|
||||
public static Set<Integer> uniqIntValuesGreaterThan42(Stream<Double> s) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.io.IOException; |
||||
import java.util.stream.Stream; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) throws IOException { |
||||
System.out.println(StreamMap.sumOfStringLength(Stream.of("Bonjour", "le", "monde !"))); |
||||
System.out.println(StreamMap.upperCaseAllString(Stream.of("IL", "Fait", "beaU !!"))); |
||||
System.out.println(StreamMap.uniqIntValuesGreaterThan42(Stream.of(23.439, 42.34, 39194.4))); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
16 |
||||
[IL, FAIT, BEAU !!] |
||||
[42, 39194] |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[Stream](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/stream/Stream.html) |
@ -0,0 +1,55 @@
|
||||
## StreamReduce |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `StreamReduce.java`. |
||||
|
||||
Create a function `sumAll` which returns sum of integers in the stream. |
||||
Create e function `divideAndAddElements` which sum the result of the division between all the integers in the stream and the divider. |
||||
|
||||
|
||||
### Expected Functions |
||||
```java |
||||
public class StreamReduce { |
||||
public static Integer sumAll(Stream<Integer> s) { |
||||
// your code here |
||||
} |
||||
|
||||
public static Integer divideAndAddElements(Stream<Integer> s, int divider) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.io.IOException; |
||||
import java.util.stream.Stream; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) throws IOException { |
||||
System.out.println(StreamReduce.sumAll(Stream.of(3, 5, 7, 10))); |
||||
System.out.println(StreamReduce.sumAll(Stream.of())); |
||||
System.out.println(StreamReduce.divideAndAddElements(Stream.of(3, 5, 7, 10), 2)); |
||||
System.out.println(StreamReduce.divideAndAddElements(Stream.of(), 2)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
25 |
||||
0 |
||||
11 |
||||
0 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[Stream](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/util/stream/Stream.html) |
||||
[Reduce](https://www.baeldung.com/java-stream-reduce) |
Loading…
Reference in new issue