mirror of https://github.com/01-edu/public.git
Browse Source
* docs: adding subjects for quest2 of the java piscine * style: add file reference to the subject of Capitalize exerice * style: fix input file * docs(Cat Exercice): add more information and fix the usage example * docs(CatInFile Exercice): add more information about the input * style(SortArgs Exercice): remove unused import from the usage examplepull/1513/head
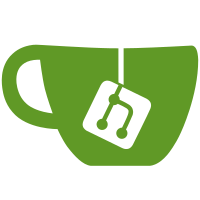
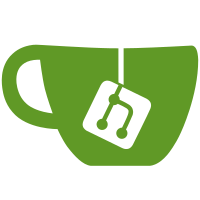
9 changed files with 404 additions and 0 deletions
@ -0,0 +1,55 @@
|
||||
## Capitalize |
||||
|
||||
### Instructions |
||||
|
||||
Create a file named `Capitalize.java`. |
||||
|
||||
Write a function `capitalize` that reads the text from a file given as the first parameter and writes the result to a file given as the second parameter. |
||||
|
||||
### Provided files |
||||
|
||||
You can find the [input](input.txt) and its [result](result.txt) files to use for the test and to understand more what you have to do. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
import java.io.*; |
||||
|
||||
public class Capitalize { |
||||
public static void capitalize(String[] args) throws IOException { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.io.*; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) throws IOException { |
||||
Capitalize.capitalize(new String[]{"input", "output"}); |
||||
String expectedResult = new String(Files.readAllBytes(Paths.get("result"))); |
||||
String userOutput = new String(Files.readAllBytes(Paths.get("output"))); |
||||
System.out.println(expectedResult.equals(userOutput)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Command-Line Arguments](https://docs.oracle.com/javase/tutorial/essential/environment/cmdLineArgs.html) |
||||
[File](https://docs.oracle.com/javase/7/docs/api/java/nio/file/Files.html) |
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
@ -0,0 +1 @@
|
||||
hello world! |
@ -0,0 +1 @@
|
||||
Hello World! |
@ -0,0 +1,56 @@
|
||||
## DoOp |
||||
|
||||
### Instructions |
||||
|
||||
Create a file named `DoOp.java`. |
||||
|
||||
Write a function `operate` that returns the result of the given arithmetic operation specified in the parameters. The arguments should be passed in the following order: |
||||
- The first argument is the right operand |
||||
- The second argument is the operation sign |
||||
- The third argument is the left operand |
||||
|
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class doOp { |
||||
public static int operate(String[] args) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(doOp.operate(new String[]{"1","+","2"})); |
||||
System.out.println(doOp.operate(new String[]{"1","-","1"})); |
||||
System.out.println(doOp.operate(new String[]{"1","%","0"})); |
||||
System.out.println(doOp.operate(args)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
3 |
||||
0 |
||||
Error |
||||
it depend on the input. |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Command-Line Arguments](https://docs.oracle.com/javase/tutorial/essential/environment/cmdLineArgs.html) |
||||
[Operators](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/operators.html) |
||||
[Conditional statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/if.html) |
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
||||
[Array](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/arrays.html) |
@ -0,0 +1,50 @@
|
||||
## FileManager |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `FileManager.java`. |
||||
|
||||
Write a function `createFile` that creates a file with the name and the content as parameter. |
||||
Write a function `getContentFile` that returns the content of the file as parameter. |
||||
Write a function `deleteFile` that deletes the file as parameter. |
||||
|
||||
### Expected Functions |
||||
```java |
||||
public class FileManager { |
||||
public static void createFile(String fileName, String content) throws IOException { |
||||
// your code here |
||||
} |
||||
public static String getContentFile(String fileName) throws IOException { |
||||
// your code here |
||||
} |
||||
public static void deleteFile(String fileName) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
```java |
||||
import java.io.IOException; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) throws IOException { |
||||
FileManager.createFile("file.txt", "Lorem ipsum"); |
||||
System.out.println(FileManager.getContentFile("file.txt")); |
||||
FileManager.deleteFile("file.txt"); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Lorem ipsum |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[File](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/io/File.html) |
@ -0,0 +1,60 @@
|
||||
## FileSearch |
||||
|
||||
### Instructions |
||||
|
||||
Create a file `FileSearch.java`. |
||||
|
||||
Write a function `searchFile` that returns the path of the file in parameter. You need to start searching from a folder named `documents` at the root of the current folder. |
||||
The file to search can contain a subfolder and the file can be in any of them. |
||||
|
||||
### Expected Functions |
||||
```java |
||||
public class FileSearch { |
||||
public static String searchFile(String fileName) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function |
||||
: |
||||
```java |
||||
import java.io.IOException; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) throws IOException { |
||||
System.out.println(FileSearch.searchFile("file.txt")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
Create the following arborescence : |
||||
``` |
||||
\ documents |
||||
| --- file.csv |
||||
| --- \ rep |
||||
| --- example.txt |
||||
| --- file2.csv |
||||
| --- file2.xml |
||||
| --- \ directory33 |
||||
| --- file.txt |
||||
| --- test.png |
||||
| --- \ directory22 |
||||
| --- test.gif |
||||
| --- \ directory435 |
||||
| ---test33.xls |
||||
|
||||
``` |
||||
|
||||
and its output : |
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
document/rep/directory33/file.txt |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
[File](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/io/File.html) |
@ -0,0 +1,54 @@
|
||||
## SortArgs |
||||
|
||||
### Instructions |
||||
|
||||
Create a file named `SortArgs.java`. |
||||
|
||||
Write a function `sort` that sorts the given array specified in the parameters and print the sorted array into the standard ouput. The elements should be separated by spaces with a new line at the end. All the given elements are correct numbers. |
||||
|
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class SortArgs { |
||||
public static void sort(String[] args) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
import java.io.*; |
||||
|
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) throws IOException { |
||||
ByteArrayOutputStream outputStream = new ByteArrayOutputStream(); |
||||
PrintStream printStream = new PrintStream(outputStream); |
||||
System.setOut(printStream); |
||||
SortArgs.sort(new String[]{"4","2","1","3"}); |
||||
String output = outputStream.toString(); |
||||
System.out.println(output.equals("1 2 3 4\n")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
true |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
[Command-Line Arguments](https://docs.oracle.com/javase/tutorial/essential/environment/cmdLineArgs.html) |
||||
[Conditional statement](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/if.html) |
||||
[String](https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/String.html) |
||||
[Array](https://docs.oracle.com/javase/tutorial/java/nutsandbolts/arrays.html) |
||||
[File](https://docs.oracle.com/javase/7/docs/api/java/nio/file/Files.html) |
Loading…
Reference in new issue