mirror of https://github.com/01-edu/public.git
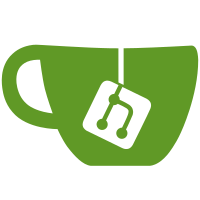
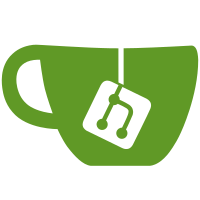
1 changed files with 63 additions and 0 deletions
@ -0,0 +1,63 @@
|
||||
## dress_code |
||||
|
||||
### Instructions |
||||
|
||||
Create a function called `choose_outfit` that receives the following input: |
||||
- a `formality_level` as an `Option<u32>`; |
||||
- an `invitation_message` as a `Result<&str>`. |
||||
|
||||
The function will return a struct `Outfit` which contains: |
||||
- `jacket`, an `enum` `Jacket` that contains `Black`, `White` and `Flowers`; |
||||
- 'hat', an `enum` `Hat` that contains `Snapback`, `Baseball`, `Fedora`. |
||||
|
||||
```rust |
||||
#[derive(Debug, PartialEq, Eq)] |
||||
pub struct Outfit { |
||||
pub jacket: Jacket, |
||||
pub hat: Hat, |
||||
} |
||||
``` |
||||
|
||||
For the `jacket`: |
||||
- the jacket should be `Flowers` when the `formality_level` is unset; |
||||
- the jacket should be `White` when the `formality_level` is more than 0; |
||||
- otherwise it should be `Black`. |
||||
|
||||
For the `hat`: |
||||
- if the `invitation_message` is `Ok()` it should be `Fedora`; |
||||
- otherwise it should be `Snapback`. |
||||
|
||||
In the specific case where `formality_level` is `None` and `invitation_message` |
||||
is not `Ok()` then the `jacket` should be `Flowers` and the `hat` should be `Baseball`. |
||||
|
||||
Remember that all the `enum` and `struct` used must be `pub`. |
||||
|
||||
### Expected functions |
||||
|
||||
```rust |
||||
pub fn choose_outfit(formality_level: Option<u32>, invitation_message: Result<&str>) -> Outfit {} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
use dress_code::*; |
||||
|
||||
fn main() { |
||||
println!("My outfit will be: {:?}", choose_outfit(Some(0), Ok("Dear friend, ..."))); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
```console |
||||
$ cargo run |
||||
My outfit will be: Outfit { jacket: Black, hat: Fedora } |
||||
... |
||||
|
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [patterns](https://doc.rust-lang.org/book/ch18-00-patterns.html) |
Loading…
Reference in new issue