forked from root/public
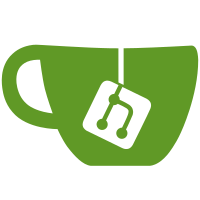
9 changed files with 178 additions and 1 deletions
@ -1 +1,2 @@
|
||||
node_modules |
||||
node_modules |
||||
**/target/** |
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "fibonacci" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "fibonacci2_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"fibonacci", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "fibonacci2_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
fibonacci = {path = "../../../../Documents/rust/rust-piscine-solutions/fibonacci"} |
@ -0,0 +1,9 @@
|
||||
use fibonacci::*; |
||||
|
||||
#[test] |
||||
fn it_works() { |
||||
assert_eq!(fibonacci(0), 0); |
||||
assert_eq!(fibonacci(1), 1); |
||||
assert_eq!(fibonacci(22), 17711); |
||||
assert_eq!(fibonacci(20), 6765); |
||||
} |
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "scalar" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "scalar_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"scalar", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "scalar_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
scalar = {path = "../../../../Documents/rust/rust-piscine-solutions/scalar"} |
@ -0,0 +1,28 @@
|
||||
use scalar::*; |
||||
|
||||
#[test] |
||||
#[should_panic] |
||||
fn test_panic_sum() { |
||||
sum(25, 255); |
||||
} |
||||
|
||||
#[test] |
||||
#[should_panic] |
||||
fn test_panic_diff() { |
||||
diff(-32768, 32766); |
||||
} |
||||
|
||||
#[test] |
||||
#[should_panic] |
||||
fn test_panic_pro() { |
||||
pro(-128, 2); |
||||
} |
||||
|
||||
#[test] |
||||
fn pass() { |
||||
assert_eq!(sum(1, 2), 3); |
||||
assert_eq!(diff(1, 2), -1); |
||||
assert_eq!(pro(1, 2), 2); |
||||
assert_eq!(quo(1.0, 2.0), 0.5); |
||||
assert_eq!(rem(1.0, 2.0), 1.0); |
||||
} |
@ -0,0 +1,37 @@
|
||||
## fibonacci2 |
||||
|
||||
### Instructions |
||||
|
||||
Complete the body of the function `fibonacci`. |
||||
|
||||
This functions receives a number n and returns the nth number in the fibonacci series. |
||||
|
||||
The Fibonacci Series starts like this: 0, 1, 1, 2, 3, 5, 8, 13... so fibonacci(2) = 1 and fibonnacci(4) = 3 |
||||
|
||||
|
||||
### Expected function |
||||
|
||||
```rust |
||||
fn fibonacci(n: u32) -> u32 { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible test for your function: |
||||
|
||||
``` |
||||
#[cfg(test)] |
||||
mod tests { |
||||
use super::*; |
||||
|
||||
#[test] |
||||
fn it_works() { |
||||
assert_eq!(fibonacci(2), 1); |
||||
assert_eq!(fibonacci(4), 1); |
||||
assert_eq!(fibonacci(22), 17711); |
||||
assert_eq!(fibonacci(20), 6765); |
||||
} |
||||
} |
||||
``` |
@ -0,0 +1,58 @@
|
||||
## Scaler |
||||
|
||||
### Instructions |
||||
Create the following functions, that receives two parameters: |
||||
- sum, that returns the sum between two values from 0 to 255 |
||||
- diff, that returns the difference between two values from -32768 to 32767 |
||||
- pro, that returns the product of the multiplication between two values from -128 to 127 |
||||
- quo, that returns the quotient of the division between two values |
||||
- rem, that returns the remainder of the division between two values |
||||
### Notions |
||||
- https://doc.rust-lang.org/book/ch03-02-data-types.html |
||||
|
||||
|
||||
### Expected functions |
||||
|
||||
```rust |
||||
pub fn sum(a:, b: ) -> { |
||||
|
||||
} |
||||
|
||||
pub fn diff(a: , b: ) -> { |
||||
|
||||
} |
||||
|
||||
pub fn pro(a: , b: ) -> { |
||||
|
||||
} |
||||
|
||||
pub fn quo(a: , b: ) -> { |
||||
|
||||
} |
||||
|
||||
pub fn rem(a: , b: ) -> { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage: |
||||
|
||||
```rust |
||||
fn main() { |
||||
// sum |
||||
println!("sum : {}", sum(234, 2)); |
||||
println!("sum : {}", sum(1, 255)); // 'ERROR: attempt to add with overflow' |
||||
// diff |
||||
println!("diff : {}", diff(234, 2)); |
||||
println!("diff : {}", diff(-32768, 32766)); // 'ERROR: attempt to subtract with overflow' |
||||
// product |
||||
println!("pro : {}", pro(23, 2)); |
||||
println!("pro : {}", pro(-128, 2)); // 'ERROR: attempt to multiply with overflow' |
||||
// quotient |
||||
println!("quo : {}", quo(22.0, 2.0)); |
||||
println!("quo : {}", quo(-128.23, 2.0)); |
||||
// remainder |
||||
println!("rem : {}", rem(22.0, 2.0)); |
||||
println!("rem : {}", rem(-128.23, 2.0)); |
||||
} |
||||
``` |
Loading…
Reference in new issue