forked from root/public
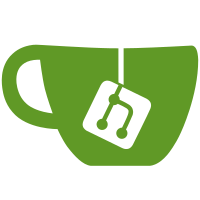
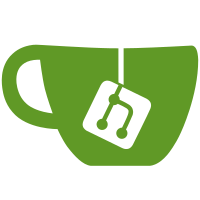
11 changed files with 477 additions and 9 deletions
@ -0,0 +1,92 @@
|
||||
## blood_types_s |
||||
|
||||
### Instructions |
||||
|
||||
Use the following table to define the methods asked: |
||||
|
||||
| Blood Types | Donate Blood to | Receive Blood From | |
||||
|-------------|------------------|--------------------| |
||||
| A+ | A+, AB+ | A+, A-, O+, O- | |
||||
| O+ | O+, A+, B+, AB+ | O+, O- | |
||||
| B+ | O+, O- | B+, B-, O+, O- | |
||||
| AB+ | AB+ | Everyone | |
||||
| A- | A+, A-, AB+, AB- | A-, O- | |
||||
| O- | Everyone | O- | |
||||
| B- | B+, B-, AB+, AB- | B-, O- | |
||||
| AB- | AB+, AB- | AB-, A-, B-, O- | |
||||
|
||||
Write three methods for BloodType: |
||||
|
||||
- `can_receive_from`: which returns true if `self` can receive blood from `other` blood type |
||||
- `donors`: which returns all the blood types that can give blood to `self` |
||||
- `recipients`: which returns all the blood types that can receive blood from `self` |
||||
|
||||
### Expected Functions and Structures |
||||
|
||||
```rust |
||||
#[derive(Debug, PartialEq, Eq, Clone, PartialOrd, Ord)] |
||||
pub enum Antigen { |
||||
A, |
||||
AB, |
||||
B, |
||||
O, |
||||
} |
||||
|
||||
#[derive(Debug, PartialEq, Eq, PartialOrd, Ord, Clone)] |
||||
enum RhFactor { |
||||
Positive, |
||||
Negative, |
||||
} |
||||
|
||||
#[derive(PartialEq, Eq, PartialOrd, Ord, Clone)] |
||||
pub struct BloodType { |
||||
pub antigen: Antigen, |
||||
pub rh_factor: RhFactor, |
||||
} |
||||
|
||||
impl BloodType { |
||||
pub fn can_receive_from(&self, other: &Self) -> bool { |
||||
} |
||||
|
||||
pub fn donors(&self) -> Vec<Self> { |
||||
} |
||||
|
||||
pub fn recipients(&self) -> Vec<Self> { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
use blood_types_s::{Antigen, BloodType, RhFactor}; |
||||
|
||||
fn main() { |
||||
let blood_type = BloodType { |
||||
antigen: Antigen::O, |
||||
rh_factor: RhFactor::Positive, |
||||
}; |
||||
println!("recipients of O+ {:?}", blood_type.recipients()); |
||||
println!("donors of O+ {:?}", blood_type.donors()); |
||||
let another_blood_type = BloodType { |
||||
antigen: Antigen::O, |
||||
rh_factor: RhFactor::Positive, |
||||
}; |
||||
println!( |
||||
"donors of O+ can receive from {:?} {:?}", |
||||
&another_blood_type, |
||||
blood_type.can_receive_from(&another_blood_type) |
||||
); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
recipients of O+ [BloodType { antigen: AB, rh_factor: Positive }, BloodType { antigen: O, rh_factor: Positive }, BloodType { antigen: A, rh_factor: Positive }, BloodType { antigen: B, rh_factor: Positive }] |
||||
donors of O+ [BloodType { antigen: O, rh_factor: Positive }, BloodType { antigen: O, rh_factor: Negative }] |
||||
donors of O+ can receive from BloodType { antigen: O, rh_factor: Positive } true |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -0,0 +1,79 @@
|
||||
## display_table |
||||
|
||||
### Instructions |
||||
|
||||
- Implement the std::fmt::Display trait for the structure table so the table is printed like in the [Usage](#usage) the length of each column must adjust to the longest element of the column and the element must be centered in the "cell" when possible, if the length of the element doesn't allow to center exactly it must alight slightly to the right. |
||||
|
||||
- Note: If the table is empty `println!` must not print anything. |
||||
|
||||
- Define the associated function `new` that create a new empty table. |
||||
|
||||
- Define the method function `add_row` that adds a new row to the table created from a slice of strings. |
||||
|
||||
### Expected function |
||||
|
||||
```rust |
||||
pub struct Table { |
||||
pub headers: Vec<String>, |
||||
pub body: Vec<Vec<String>>, |
||||
} |
||||
|
||||
impl fmt::Display for Table { |
||||
} |
||||
|
||||
impl Table { |
||||
pub fn new() -> Table { |
||||
} |
||||
|
||||
pub fn add_row(&mut self, row: &[String]) { |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible test for your function: |
||||
|
||||
```rust |
||||
fn main() { |
||||
let mut table = Table::new(); |
||||
println!("{}", table); |
||||
table.headers = vec![ |
||||
String::from("Model"), |
||||
String::from("Piece N°"), |
||||
String::from("In Stock"), |
||||
String::from("Description"), |
||||
]; |
||||
table.add_row(&[ |
||||
String::from("model 1"), |
||||
String::from("43-EWQE304"), |
||||
String::from("30"), |
||||
String::from("Piece for x"), |
||||
]); |
||||
table.add_row(&[ |
||||
String::from("model 2"), |
||||
String::from("98-QCVX5433"), |
||||
String::from("100000000"), |
||||
String::from("-"), |
||||
]); |
||||
table.add_row(&[ |
||||
String::from("model y"), |
||||
String::from("78-NMNH"), |
||||
String::from("60"), |
||||
String::from("nothing"), |
||||
]); |
||||
println!("{}", table); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
| Model | Piece N° | In Stock | Description | |
||||
|---------+-------------+-----------+-------------| |
||||
| model 1 | 43-EWQE304 | 30 | Piece for x | |
||||
| model 2 | 98-QCVX5433 | 100000000 | - | |
||||
| model y | 78-NMNH | 60 | nothing | |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -0,0 +1,85 @@
|
||||
## filter_table |
||||
|
||||
### Instructions |
||||
|
||||
- Define the functions: |
||||
|
||||
- new: creates a new empty table. |
||||
|
||||
- add_rows: adds a new row to the table from a slice of strings. |
||||
|
||||
- filter_cols: that receives a closure that receives a `&str` and returns a `bool` value: |
||||
|
||||
- filter_cols returns a table with all the columns that yielded true when applied to the header. |
||||
|
||||
- filter_rows: that receives a closure that receives a `&str` and returns a `bool` value |
||||
|
||||
- filter_rows returns a table with all the columns that yielded true when applied to the elements of the selected column. |
||||
|
||||
### Expected function |
||||
|
||||
```rust |
||||
pub struct Table { |
||||
pub headers: Vec<String>, |
||||
pub body: Vec<Vec<String>>, |
||||
} |
||||
|
||||
impl Table { |
||||
pub fn new() -> Table { |
||||
} |
||||
|
||||
pub fn add_row(&mut self, row: &[String]) { |
||||
} |
||||
|
||||
pub fn filter_col(&self, filter: ) -> Option<Self> { |
||||
|
||||
} |
||||
|
||||
pub fn filter_row(&self, col_name: &str, filter: ) -> Option<Self> { |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible test for your function: |
||||
|
||||
```rust |
||||
fn main() { |
||||
let mut table = Table::new(); |
||||
table.headers = vec![ |
||||
"Name".to_string(), |
||||
"Last Name".to_string(), |
||||
"ID Number".to_string(), |
||||
]; |
||||
table.add_row(&[ |
||||
"Adam".to_string(), |
||||
"Philips".to_string(), |
||||
"123456789".to_string(), |
||||
]); |
||||
table.add_row(&[ |
||||
"Adamaris".to_string(), |
||||
"Shelby".to_string(), |
||||
"1111123456789".to_string(), |
||||
]); |
||||
table.add_row(&[ |
||||
"Ackerley".to_string(), |
||||
"Philips".to_string(), |
||||
"123456789".to_string(), |
||||
]); |
||||
let filter_names = |col: &str| col == "Name"; |
||||
println!("{:?}", table.filter_col(filter_names)); |
||||
|
||||
let filter_philips = |lastname: &str| lastname == "Philips"; |
||||
println!("{:?}", table.filter_row("Last Name", filter_philips)); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
Some(Table { headers: ["Name"], body: [["Adam"], ["Adamaris"], ["Ackerley"]] }) |
||||
Some(Table { headers: ["Name", "Last Name", "ID Number"], body: [["Adam", "Philips", "123456789"], ["Ackerley", "Philips", "123456789"]] }) |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -0,0 +1,43 @@
|
||||
## flat_rust |
||||
|
||||
### Instructions |
||||
|
||||
- Define the functions `flatten_tree` that receives a std::collections::BTreeSet and returns a new `Vec` with the elements in the binary tree in order. |
||||
|
||||
### Expected function |
||||
|
||||
```rust |
||||
pub fn flatten_tree<T: ToOwned<Owned = T>>(tree: &BTreeSet<T>) -> Vec<T> { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible test for your function: |
||||
|
||||
```rust |
||||
fn main() { |
||||
let mut tree = BTreeSet::new(); |
||||
tree.insert(34); |
||||
tree.insert(0); |
||||
tree.insert(9); |
||||
tree.insert(30); |
||||
println!("{:?}", flatten_tree(&tree)); |
||||
|
||||
let mut tree = BTreeSet::new(); |
||||
tree.insert("Slow"); |
||||
tree.insert("kill"); |
||||
tree.insert("will"); |
||||
tree.insert("Horses"); |
||||
println!("{:?}", flatten_tree(&tree)); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
[0, 9, 30, 34] |
||||
["Horses", "Slow", "kill", "will"] |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
After Width: | Height: | Size: 66 KiB |
@ -0,0 +1,56 @@
|
||||
## insertion_sort |
||||
|
||||
### Instructions |
||||
|
||||
The insertion sort algorithm: |
||||
|
||||
- To sort an array of size n in ascending order: |
||||
|
||||
1. Iterate from slice[1] to slice[n] over the slice. |
||||
|
||||
2. Compare the current element (key) to its predecessor. |
||||
|
||||
3. If the key element is smaller than its predecessor, compare it to the elements before. Move the greater elements one position up to make space for the swapped element. |
||||
|
||||
Here is a visual example of sorting a slice step by step using the insertion sort algorithm. |
||||
|
||||
 |
||||
**Figure 1** - Step by step execution of the algorithm insertion sort |
||||
|
||||
- Implement the algorithm insertion sort by creating a function `insertion_sort(slice, steps)` that executes the iterations of the algorithm the number of steps indicated by the parameter `steps`. See the [Usage](#usage) for more information. |
||||
|
||||
### Expected Function |
||||
|
||||
```rust |
||||
pub fn insertion_sort(slice: &mut [i32], steps: usize) { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function |
||||
|
||||
```rust |
||||
fn main() { |
||||
let mut target = [5, 3, 7, 2, 1, 6, 8, 4]; |
||||
// executes the first iteration of the algorithm |
||||
insertion_sort(&mut target, 1); |
||||
println!("{:?}", target); |
||||
|
||||
let mut target = [5, 3, 7, 2, 1, 6, 8, 4]; |
||||
let len = target.len(); |
||||
// executes len - 1 iterations of the algorithm |
||||
// i.e. sorts the slice |
||||
insertion_sort(&mut target, len - 1); |
||||
println!("{:?}", target); |
||||
} |
||||
``` |
||||
|
||||
And it's output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
[3, 5, 7, 2, 1, 6, 8, 4] |
||||
[1, 2, 3, 4, 5, 6, 7, 8] |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -0,0 +1,44 @@
|
||||
## matrix_display |
||||
|
||||
### Instructions |
||||
|
||||
Use the Matrix struct given in the [expected struct](#expected-functions-and-struct) and implement the `std::fmt::Display` trait so it prints the matrix like in the [usage](#usage). |
||||
|
||||
You will also have to implement the associated function `new` that creates a matrix from a slice of slices. |
||||
|
||||
### Expected Functions and Struct |
||||
|
||||
```rust |
||||
pub struct Matrix(pub Vec<Vec<i32>>); |
||||
|
||||
pub fn new(slice: &[&[i32]]) -> Self { |
||||
} |
||||
|
||||
use std::fmt; |
||||
|
||||
impl fmt::Display for Matrix { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function |
||||
|
||||
```rust |
||||
use matrix_display::*; |
||||
|
||||
fn main() { |
||||
let matrix = Matrix::new(&[&[1, 2, 3], &[4, 5, 6], &[7, 8, 9]]); |
||||
println!("{}", matrix); |
||||
} |
||||
``` |
||||
|
||||
And it's output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
(1 2 3) |
||||
(4 5 6) |
||||
(7 8 9) |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -0,0 +1,69 @@
|
||||
## matrix_transposition_4by3 |
||||
|
||||
### Instructions |
||||
|
||||
- Define the structure matrix as a tuple of tuples of `i32`'s |
||||
|
||||
- Define a function that calculate the transpose matrix of a 4x3 matrix (4 rows by 3 columns) which is a 3x4 matrix (3 rows by 4 columns). |
||||
|
||||
- Note: |
||||
|
||||
- The transpose of a matrix `A` is the matrix `A'` where `A'`'s columns are `A`'s row and the rows are the columns: |
||||
|
||||
Example: |
||||
|
||||
``` |
||||
( a b c ) __ transposition __> ( a d g j ) |
||||
( d e f ) ( b e h k ) |
||||
( g h i ) ( c f i l ) |
||||
( j k l ) |
||||
``` |
||||
|
||||
- Matrix must implement Debug, PartialEq and Eq. You can use derive |
||||
|
||||
- Remember that you're defining a library so you have to make public the elements that are going to be called from an external crate. |
||||
|
||||
### Notions |
||||
|
||||
[Chapter 7]( https://doc.rust-lang.org/stable/book/ch07-03-paths-for-referring-to-an-item-in-the-module-tree.html ) |
||||
|
||||
### Expected Function and Structs |
||||
|
||||
```rust |
||||
pub struct Matrix4by3( |
||||
pub (i32, i32, i32), |
||||
pub (i32, i32, i32), |
||||
pub (i32, i32, i32), |
||||
pub (i32, i32, i32), |
||||
); |
||||
|
||||
pub struct Matrix3by4( |
||||
pub (i32, i32, i32, i32), |
||||
pub (i32, i32, i32, i32), |
||||
pub (i32, i32, i32, i32), |
||||
); |
||||
|
||||
pub fn transpose(m: Matrix4by3) -> Matrix3by4 { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a posible program to test your function |
||||
|
||||
```rust |
||||
fn main() { |
||||
let matrix = Matrix4by3((1, 2, 3), (4, 5, 6), (7, 8, 9), (10, 11, 12)); |
||||
println!("Original matrix {:?}", matrix); |
||||
println!("Transpose matrix {:?}", transpose(matrix)); |
||||
} |
||||
``` |
||||
|
||||
And it's output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
Original matrix Matrix4by3((1, 2, 3), (4, 5, 6), (7, 8, 9), (10, 11, 12)) |
||||
Transpose matrix Matrix3by4((1, 4, 7, 10), (2, 5, 8, 11), (3, 6, 9, 12)) |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue