forked from root/public
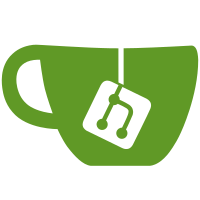
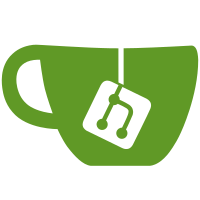
22 changed files with 0 additions and 630 deletions
@ -1,56 +0,0 @@
|
||||
## atoibaseprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that takes a `string` number and its `string` base in parameters and returns its conversion as an `int`. |
||||
|
||||
If the base or the `string` number is not valid it returns `0` |
||||
|
||||
Validity rules for a base : |
||||
|
||||
- A base must contain at least 2 characters. |
||||
- Each character of a base must be unique. |
||||
- A base should not contain `+` or `-` characters. |
||||
|
||||
Only valid `string` numbers will be tested. |
||||
|
||||
The function **does not have** to manage negative numbers. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func AtoiBase(s string, base string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(AtoiBase("125", "0123456789")) |
||||
fmt.Println(AtoiBase("1111101", "01")) |
||||
fmt.Println(AtoiBase("7D", "0123456789ABCDEF")) |
||||
fmt.Println(AtoiBase("uoi", "choumi")) |
||||
fmt.Println(AtoiBase("bbbbbab", "-ab")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
125 |
||||
125 |
||||
125 |
||||
125 |
||||
0 |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -1,74 +0,0 @@
|
||||
## atoiprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that simulates the behaviour of the `Atoi` function in Go. `Atoi` transforms a number represented as a `string` in a number represented as an `int`. |
||||
|
||||
- `Atoi` returns `0` if the `string` is not considered as a valid number. For this exercise **non-valid `string` chains will be tested**. Some will contain non-digits characters. |
||||
|
||||
- For this exercise the handling of the signs + or - **does have** to be taken into account. |
||||
|
||||
- This function will **only** have to return the `int`. For this exercise the `error` result of atoi is not required. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Atoi(s string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
s := "12345" |
||||
s2 := "0000000012345" |
||||
s3 := "012 345" |
||||
s4 := "Hello World!" |
||||
s5 := "+1234" |
||||
s6 := "-1234" |
||||
s7 := "++1234" |
||||
s8 := "--1234" |
||||
|
||||
n := Atoi(s) |
||||
n2 := Atoi(s2) |
||||
n3 := Atoi(s3) |
||||
n4 := Atoi(s4) |
||||
n5 := Atoi(s5) |
||||
n6 := Atoi(s6) |
||||
n7 := Atoi(s7) |
||||
n8 := Atoi(s8) |
||||
|
||||
fmt.Println(n) |
||||
fmt.Println(n2) |
||||
fmt.Println(n3) |
||||
fmt.Println(n4) |
||||
fmt.Println(n5) |
||||
fmt.Println(n6) |
||||
fmt.Println(n7) |
||||
fmt.Println(n8) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ go build |
||||
student@ubuntu:~/[[ROOT]]/test$ ./test |
||||
12345 |
||||
12345 |
||||
0 |
||||
0 |
||||
1234 |
||||
-1234 |
||||
0 |
||||
0 |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -1,23 +0,0 @@
|
||||
## capitalizeprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that capitalizes the first letter of each word **and** lowercases the rest of each word of a `string`. |
||||
|
||||
- A word is a sequence of **alphanumerical** characters. |
||||
|
||||
- If there is more than one argument the program should print `Too many arguments`. |
||||
|
||||
- If there is no arguments given the program should print nothing. |
||||
|
||||
### Usage : |
||||
|
||||
```console |
||||
student@ubuntu:~/capitalizeprog$ go build |
||||
student@ubuntu:~/capitalizeprog$ ./capitalizeprog "Hello! How are you? How+are+things+4you?" | cat -e |
||||
Hello! How Are You? How+Are+Things+4you?$ |
||||
student@ubuntu:~/capitalizeprog$ ./capitalizeprog Hello! How are you? | cat -e |
||||
Too many arguments$ |
||||
student@ubuntu:~/capitalizeprog$ ./capitalizeprog |
||||
student@ubuntu:~/capitalizeprog$ |
||||
``` |
@ -1,24 +0,0 @@
|
||||
## compareprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that behaves like the `Compare` function from the `Go` package `strings`. |
||||
|
||||
This program prints a number after comparing two `string` lexicographically. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/compareprog$ go build |
||||
student@ubuntu:~/compareprog$ ./compareprog a b | cat -e |
||||
-1$ |
||||
student@ubuntu:~/compareprog$ ./compareprog a a | cat -e |
||||
0$ |
||||
student@ubuntu:~/compareprog$ ./compareprog b a | cat -e |
||||
1$ |
||||
student@ubuntu:~/compareprog$ ./compareprog b a d | cat -e |
||||
$ |
||||
student@ubuntu:~/compareprog$ ./compareprog | cat -e |
||||
$ |
||||
student@ubuntu:~/compareprog$ |
||||
``` |
@ -1,13 +0,0 @@
|
||||
## firstruneprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the first `rune` of a `string`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func FirstRune(s string) rune { |
||||
|
||||
} |
||||
``` |
@ -1,39 +0,0 @@
|
||||
## foreachprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ForEach` that, for an `int` slice, applies a function on each elements of that slice. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ForEach(f func(int), a []int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
a := []int{1, 2, 3, 4, 5, 6} |
||||
ForEach(PrintNbr, a) |
||||
} |
||||
|
||||
func PrintNbr(int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
123456 |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -1,26 +0,0 @@
|
||||
## itoabase |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that: |
||||
|
||||
- converts an `int` value to a `string` using the specified base in the argument |
||||
- and that returns this `string` |
||||
|
||||
The base is expressed as an `int`, from 2 to 16. The characters comprising |
||||
the base are the digits from 0 to 9, followed by uppercase letters from A to F. |
||||
|
||||
For example, the base `4` would be the equivalent of "0123" and the base `16` would be the equivalent of "0123456789ABCDEF". |
||||
|
||||
If the value is negative, the resulting `string` has to be preceded with a |
||||
minus sign `-`. |
||||
|
||||
Only valid inputs will be tested. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ItoaBase(value, base int) string { |
||||
|
||||
} |
||||
``` |
@ -1,15 +0,0 @@
|
||||
## itoaprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that simulates the behaviour of the `Itoa` function in Go. `Itoa` transforms a number represented as an `int` in a number represented as a `string`. |
||||
|
||||
- For this exercise the handling of the signs + or - **does have** to be taken into account. |
||||
|
||||
## Expected function |
||||
|
||||
```go |
||||
func Itoa(n int) string { |
||||
|
||||
} |
||||
``` |
@ -1,13 +0,0 @@
|
||||
## lastruneprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the last `rune` of a `string`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func LastRune(s string) rune { |
||||
|
||||
} |
||||
``` |
@ -1,23 +0,0 @@
|
||||
## listremoveifprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ListRemoveIf` that removes all elements that have a `Data` field equal to the `data_ref` in the argument of the function. |
||||
|
||||
### Expected function and structure |
||||
|
||||
```go |
||||
type NodeL struct { |
||||
Data interface{} |
||||
Next *NodeL |
||||
} |
||||
|
||||
type List struct { |
||||
Head *NodeL |
||||
Tail *NodeL |
||||
} |
||||
|
||||
func ListRemoveIf(l *List, data_ref interface{}) { |
||||
|
||||
} |
||||
``` |
@ -1,23 +0,0 @@
|
||||
## listsizeprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ListSize` that returns the number of elements in a linked list `l`. |
||||
|
||||
### Expected function and structure |
||||
|
||||
```go |
||||
type NodeL struct { |
||||
Data interface{} |
||||
Next *NodeL |
||||
} |
||||
|
||||
type List struct { |
||||
Head *NodeL |
||||
Tail *NodeL |
||||
} |
||||
|
||||
func ListSize(l *List) int { |
||||
|
||||
} |
||||
``` |
@ -1,38 +0,0 @@
|
||||
## maxprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, `Max`, that returns the maximum value in a slice of integers. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Max(a []int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
arrInt := []int{23, 123, 1, 11, 55, 93} |
||||
max := Max(arrInt) |
||||
fmt.Println(max |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
123 |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -1,46 +0,0 @@
|
||||
## nruneprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the nth `rune` of a `string`. |
||||
|
||||
- In case of impossibilities, the function returns `0`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func NRune(s string, n int) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
z01.PrintRune(piscine.NRune("Hello!", 3)) |
||||
z01.PrintRune(piscine.NRune("Salut!", 2)) |
||||
z01.PrintRune(piscine.NRune("Bye!", -1)) |
||||
z01.PrintRune(piscine.NRune("Bye!", 5)) |
||||
z01.PrintRune(piscine.NRune("Ola!", 4)) |
||||
z01.PrintRune('\n') |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ go build |
||||
student@ubuntu:~/[[ROOT]]/test$ ./test |
||||
la! |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -1,22 +0,0 @@
|
||||
## printcombprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that prints in ascending order on a single line all unique combinations of three different digits so that the first digit is lower than the second and the second is lower than the third. |
||||
|
||||
These combinations are separated by a comma and a space. |
||||
|
||||
### Usage |
||||
|
||||
Here is an **incomplete** output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/printcombprog$ go build |
||||
student@ubuntu:~/[[ROOT]]/printcombprog$ ./printcombprog | cat -e |
||||
012, 013, 014, 015, 016, 017, 018, 019, 023, ..., 689, 789$ |
||||
student@ubuntu:~/[[ROOT]]/printcombprog$ |
||||
``` |
||||
|
||||
`000` or `999` are not valid combinations because the digits are not different. |
||||
|
||||
`987` should not be shown because the first digit is not less than the second. |
@ -1,16 +0,0 @@
|
||||
## printdigitsprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that prints the decimal digits in ascending order (from `0` to `9`) on a single line. |
||||
|
||||
A line is a sequence of characters preceding the [end of line](https://en.wikipedia.org/wiki/Newline) character (`'\n'`). |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/printdigitsprog$ go build |
||||
student@ubuntu:~/printdigitsprog$ ./main |
||||
0123456789 |
||||
student@ubuntu:~/printdigitsprog$ |
||||
``` |
@ -1,17 +0,0 @@
|
||||
## printstrprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a program that prints one by one the characters of a `string` passed as an argument of the program. |
||||
|
||||
### Expected output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/printstrprog$ go build |
||||
student@ubuntu:~/[[ROOT]]/printstrprog$ ./printstrprog "Hello World!" | cat -e |
||||
Hello World!$ |
||||
student@ubuntu:~/[[ROOT]]/printstrprog$ ./printstrprog |
||||
student@ubuntu:~/[[ROOT]]/printstrprog$ |
||||
student@ubuntu:~/[[ROOT]]/printstrprog$ ./printstrprog "Hello" "World" |
||||
student@ubuntu:~/[[ROOT]]/printstrprog$ |
||||
``` |
@ -1,16 +0,0 @@
|
||||
## rot14 |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that prints the `string` passed as argument, transformed into a `rot14 string`. |
||||
|
||||
### Usage |
||||
|
||||
```console |
||||
student@ubuntu:~/rot14prog$ go build |
||||
student@ubuntu:~/rot14prog$ ./rot14prog "Hello How are You" | cat -e |
||||
Vszzc Vck ofs Mci$ |
||||
student@ubuntu:~/rot14prog$ ./rot14prog Hello How are You |
||||
student@ubuntu:~/rot14prog$ ./rot14prog |
||||
student@ubuntu:~/rot14prog$ |
||||
``` |
@ -1,38 +0,0 @@
|
||||
## sortwordarrprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `SortWordArr` that sorts by ASCII (in ascending order) a `string` slice. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func SortWordArr(a []string) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
result := []string{"a", "A", "1", "b", "B", "2", "c", "C", "3"} |
||||
SortWordArr(result) |
||||
fmt.Println(result) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
[1 2 3 A B C a b c] |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -1,42 +0,0 @@
|
||||
## splitprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that separates the words of a `string` and puts them in a `string` slice. |
||||
|
||||
The separators are the characters of the separator string given in parameter. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Split(s, sep string) []string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
s := "HelloHAhowHAareHAyou?" |
||||
fmt.Printf("%#v\n", piscine.Split(s, "HA")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ go build |
||||
student@ubuntu:~/[[ROOT]]/test$ ./test |
||||
[]string{"Hello", "how", "are", "you?"} |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -1,38 +0,0 @@
|
||||
## strlenprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that counts the `runes` of a `string` and that returns that count. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func StrLen(s string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
s := "Hello World!" |
||||
nb := StrLen(s) |
||||
fmt.Println(nb) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ go build |
||||
student@ubuntu:~/[[ROOT]]/test$ ./test |
||||
12 |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
@ -1,15 +0,0 @@
|
||||
## strrevprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a program that reverses a `string` and prints it in the standard output. |
||||
|
||||
### Expected output : |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/strrevprog$ go build |
||||
student@ubuntu:~/[[ROOT]]/strrevprog$ ./strrevprog "Hello World!" | cat -e |
||||
!dlroW olleH$ |
||||
student@ubuntu:~/[[ROOT]]/strrevprog$ ./strrevprog |
||||
student@ubuntu:~/[[ROOT]]/strrevprog$ |
||||
``` |
@ -1,13 +0,0 @@
|
||||
## swapprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that swaps the contents of two **pointers to an int** (`*int`). |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Swap(a *int, b *int) { |
||||
|
||||
} |
||||
``` |
Loading…
Reference in new issue