forked from root/public
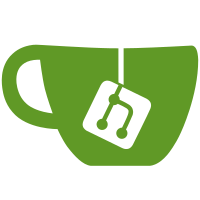
4 changed files with 133 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "simple-hash_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"simple_hash", |
||||
] |
||||
|
||||
[[package]] |
||||
name = "simple_hash" |
||||
version = "0.1.0" |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "simple-hash_test" |
||||
version = "0.1.0" |
||||
authors = ["MSilva95 <miguel-silva98@hotmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
simple_hash = { path = "../../../../rust-piscine-solutions/simple_hash"} |
@ -0,0 +1,56 @@
|
||||
// Create the function `contain` that checks a `HashMap` to see if it contains the given key.
|
||||
// Create the function `remove` that removes a given key from the `HashMap`.
|
||||
|
||||
use std::collections::HashMap; |
||||
|
||||
use simple_hash::*; |
||||
|
||||
fn main() { |
||||
let mut hash: HashMap<&str, i32> = HashMap::new(); |
||||
hash.insert("Daniel", 122); |
||||
hash.insert("Ashley", 333); |
||||
hash.insert("Katie", 334); |
||||
hash.insert("Robert", 14); |
||||
|
||||
println!( |
||||
"Does the HashMap contains the name Roman? => {}", |
||||
contain(hash.clone(), "Roman") |
||||
); |
||||
println!( |
||||
"Does the HashMap contains the name Katie? => {}", |
||||
contain(hash.clone(), "Katie") |
||||
); |
||||
println!("Removing Robert {:?}", remove(hash.clone(), "Robert")); |
||||
println!("Hash {:?}", hash); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_contains() { |
||||
let mut s = HashMap::new(); |
||||
|
||||
s.insert("Pedro", 43); |
||||
s.insert("Ralph", 12); |
||||
s.insert("Johnny", 546); |
||||
s.insert("Albert", 12323214); |
||||
|
||||
assert_eq!(true, contain(s.clone(), "Pedro")); |
||||
assert_eq!(true, contain(s.clone(), "Ralph")); |
||||
assert_eq!(true, contain(s.clone(), "Johnny")); |
||||
assert_eq!(true, contain(s.clone(), "Albert")); |
||||
assert_eq!(false, contain(s.clone(), "Marco")); |
||||
assert_eq!(false, contain(s.clone(), "Joan")); |
||||
assert_eq!(false, contain(s.clone(), "Louise")); |
||||
} |
||||
|
||||
#[test] |
||||
fn test_remove() { |
||||
let mut n = HashMap::new(); |
||||
n.insert("Dani Sordo", 37); |
||||
n.insert("Sébastien Loeb", 46); |
||||
n.insert("Ott Tanak", 32); |
||||
n.insert("Thierry Neuville", 32); |
||||
|
||||
remove(n.clone(), "Dani Sordo"); |
||||
assert_eq!(true, contain(n.clone(), "Ott Tanak")); |
||||
assert_eq!(false, contain(n.clone(), "Dani Ŝordo")) |
||||
} |
@ -0,0 +1,55 @@
|
||||
## simple_hash |
||||
|
||||
### Instructions |
||||
|
||||
- Create the function `contain` that checks a `HashMap` to see if it contains the given key. |
||||
|
||||
- Create the function `remove` that removes a given key from the `HashMap`. |
||||
|
||||
### Expected Functions |
||||
|
||||
```rust |
||||
fn contain(h: HashMap<&str, i32>, s: &str) -> bool { |
||||
} |
||||
|
||||
fn remove(mut h: HashMap<&str, i32>, s: &str) { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
use std::collections::HashMap; |
||||
|
||||
fn main() { |
||||
let mut hash: HashMap<&str, i32> = HashMap::new(); |
||||
hash.insert("Daniel", 122); |
||||
hash.insert("Ashley", 333); |
||||
hash.insert("Katie", 334); |
||||
hash.insert("Robert", 14); |
||||
|
||||
println!( |
||||
"Does the HashMap contains the name Roman? => {}", |
||||
contain(hash.clone(), "Roman") |
||||
); |
||||
println!( |
||||
"Does the HashMap contains the name Katie? => {}", |
||||
contain(hash.clone(), "Katie") |
||||
); |
||||
println!("Removing Robert {:?}", remove(hash.clone(), "Robert")); |
||||
println!("Hash {:?}", hash); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
Does the HashMap contains the name Roman? => false |
||||
Does the HashMap contains the name Katie? => true |
||||
Removing Robert () |
||||
Hash {"Daniel": 122, "Ashley": 333, "Robert": 14, "Katie": 334} |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue