forked from root/public
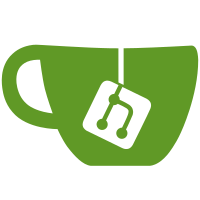
4 changed files with 96 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "find_factorial" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "find_factorial_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"find_factorial", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "find_factorial_test" |
||||
version = "0.1.0" |
||||
authors = ["MSilva95 <miguel-silva98@hotmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
find_factorial = { path = "../../../../rust-piscine-solutions/find_factorial"} |
@ -0,0 +1,34 @@
|
||||
// Complete this function to return the factorial of a given number
|
||||
|
||||
pub fn factorial(num: u64) -> u64 { |
||||
match num { |
||||
0 | 1 => 1, |
||||
_ => factorial(num - 1) * num, |
||||
} |
||||
} |
||||
|
||||
fn main() { |
||||
println!("The factorial of 0 = {}", factorial(0));
|
||||
println!("The factorial of 1 = {}", factorial(1));
|
||||
println!("The factorial of 5 = {}", factorial(5));
|
||||
println!("The factorial of 10 = {}", factorial(10));
|
||||
println!("The factorial of 19 = {}", factorial(19));
|
||||
} |
||||
|
||||
#[cfg(test)] |
||||
mod tests { |
||||
use super::*; |
||||
|
||||
#[test] |
||||
fn factorial_of_1() { |
||||
assert_eq!(1, factorial(0)); |
||||
assert_eq!(1, factorial(1)); |
||||
assert_eq!(120, factorial(5)); |
||||
assert_eq!(40320, factorial(8)); |
||||
assert_eq!(3628800, factorial(10)); |
||||
assert_eq!(87178291200, factorial(14)); |
||||
assert_eq!(6402373705728000, factorial(18)); |
||||
assert_eq!(121645100408832000, factorial(19)); |
||||
assert_eq!(2432902008176640000, factorial(20)); |
||||
} |
||||
} |
@ -0,0 +1,40 @@
|
||||
## find_factorial |
||||
|
||||
### Instruccions |
||||
|
||||
Complete the function `factorial` to return the factorial of a given number |
||||
|
||||
### Expected Function |
||||
|
||||
```rust |
||||
pub fn factorial(num: u64) -> u64 { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```rust |
||||
use find_factorial::factorial; |
||||
|
||||
fn main() { |
||||
println!("The factorial of 0 = {}", factorial(0)); |
||||
println!("The factorial of 1 = {}", factorial(1)); |
||||
println!("The factorial of 5 = {}", factorial(5)); |
||||
println!("The factorial of 10 = {}", factorial(10)); |
||||
println!("The factorial of 19 = {}", factorial(19)); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
The factorial of 0 = 1 |
||||
The factorial of 1 = 1 |
||||
The factorial of 5 = 120 |
||||
The factorial of 10 = 3628800 |
||||
The factorial of 19 = 121645100408832000 |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue