forked from root/public
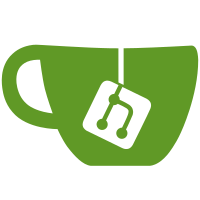
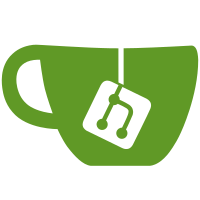
18 changed files with 489 additions and 160 deletions
@ -1,38 +1,58 @@
|
||||
## atoibaseprog |
||||
## atoibase |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that takes a `string` number and its `string` base as arguments and prints its conversion as an `int`. |
||||
Write a function that takes a `string` number and its `string` base in parameters and returns its conversion as an `int`. |
||||
|
||||
- If the base or the `string` number is not valid it returns `0`. |
||||
|
||||
- If the number of arguments is bigger or lower that two it should print a newline ("`\n`"). |
||||
If the base or the `string` number is not valid it returns `0`: |
||||
|
||||
Validity rules for a base : |
||||
|
||||
- A base must contain at least 2 characters. |
||||
- Each character of a base must be unique. |
||||
- A base should not contain `+` or `-` characters. |
||||
- A base must contain at least 2 characters. |
||||
- Each character of a base must be unique. |
||||
- A base should not contain `+` or `-` characters. |
||||
|
||||
Only valid `string` numbers will be tested. |
||||
|
||||
The program **does not have** to manage negative numbers. |
||||
The function **does not have** to manage negative numbers. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func AtoiBase(s string, base string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
### Expected output : |
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(AtoiBase("125", "0123456789")) |
||||
fmt.Println(AtoiBase("1111101", "01")) |
||||
fmt.Println(AtoiBase("7D", "0123456789ABCDEF")) |
||||
fmt.Println(AtoiBase("uoi", "choumi")) |
||||
fmt.Println(AtoiBase("bbbbbab", "-ab")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/atoibaseprog$ go build |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 125 0123456789 |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
125 |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 1111101 01 |
||||
125 |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 7D 0123456789ABCDEF |
||||
125 |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog uoi choumi | cat -e |
||||
125$ |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog bbbbbab -ab | cat -e |
||||
0$ |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 1111101 |
||||
|
||||
student@ubuntu:~/atoibaseprog$ |
||||
125 |
||||
0 |
||||
student@ubuntu:~/test$ |
||||
``` |
||||
|
@ -1,38 +1,58 @@
|
||||
## atoibaseprog |
||||
## atoibase |
||||
|
||||
### Instructions |
||||
|
||||
Écrire un programme qui prend un nombre `string` et sa base `string` en paramètres et retourne sa conversion en `int`. |
||||
Écrire une fonction qui prend un nombre `string` et sa base `string` en paramètres et retourne sa conversion en `int`. |
||||
|
||||
- Si la base ou le nombre `string` n'est pas valide le programme retourne `0`: |
||||
|
||||
- Si le nombre d'argument est différent de deux alors le programme affiche un newline ("`\n`"). |
||||
Si la base n'est pas valide elle retourne `0`: |
||||
|
||||
Règles de validité d'une base : |
||||
|
||||
- Une base doit contenir au moins 2 caractères. |
||||
- Chaque caractère d'une base doit être unique. |
||||
- Une base ne doit pas contenir les caractères `+` ou `-`. |
||||
- Une base doit contenir au moins 2 caractères. |
||||
- Chaque caractère d'une base doit être unique. |
||||
- Une base ne doit pas contenir les caractères `+` ou `-`. |
||||
|
||||
Seuls des nombres en `string` valides seront testés. |
||||
|
||||
La fonction **ne doit pas** gérer les nombres négatifs. |
||||
|
||||
### Expected output : |
||||
### Fonction attendue |
||||
|
||||
```go |
||||
func AtoiBase(s string, base string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Utilisation |
||||
|
||||
Voici un éventuel [programme](TODO-LINK) pour tester votre fonction : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(AtoiBase("125", "0123456789")) |
||||
fmt.Println(AtoiBase("1111101", "01")) |
||||
fmt.Println(AtoiBase("7D", "0123456789ABCDEF")) |
||||
fmt.Println(AtoiBase("uoi", "choumi")) |
||||
fmt.Println(AtoiBase("bbbbbab", "-ab")) |
||||
} |
||||
``` |
||||
|
||||
Et son résultat : |
||||
|
||||
```console |
||||
student@ubuntu:~/atoibaseprog$ go build |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 125 0123456789 |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
125 |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 1111101 01 |
||||
125 |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 7D 0123456789ABCDEF |
||||
125 |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog uoi choumi | cat -e |
||||
125$ |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog bbbbbab -ab | cat -e |
||||
0$ |
||||
student@ubuntu:~/atoibaseprog$ ./atoibaseprog 1111101 |
||||
|
||||
student@ubuntu:~/atoibaseprog$ |
||||
125 |
||||
0 |
||||
student@ubuntu:~/test$ |
||||
``` |
||||
|
@ -0,0 +1,76 @@
|
||||
## atoiprog |
||||
|
||||
### Instructions |
||||
|
||||
- Write a [function](TODO-LINK) that simulates the behaviour of the `Atoi` function in Go. `Atoi` transforms a number represented as a `string` in a number represented as an `int`. |
||||
|
||||
- `Atoi` returns `0` if the `string` is not considered as a valid number. For this exercise **non-valid `string` chains will be tested**. Some will contain non-digits characters. |
||||
|
||||
- For this exercise the handling of the signs + or - **does have** to be taken into account. |
||||
|
||||
- This function will **only** have to return the `int`. For this exercise the `error` result of atoi is not required. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Atoi(s string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
s := "12345" |
||||
s2 := "0000000012345" |
||||
s3 := "012 345" |
||||
s4 := "Hello World!" |
||||
s5 := "+1234" |
||||
s6 := "-1234" |
||||
s7 := "++1234" |
||||
s8 := "--1234" |
||||
|
||||
n := Atoi(s) |
||||
n2 := Atoi(s2) |
||||
n3 := Atoi(s3) |
||||
n4 := Atoi(s4) |
||||
n5 := Atoi(s5) |
||||
n6 := Atoi(s6) |
||||
n7 := Atoi(s7) |
||||
n8 := Atoi(s8) |
||||
|
||||
fmt.Println(n) |
||||
fmt.Println(n2) |
||||
fmt.Println(n3) |
||||
fmt.Println(n4) |
||||
fmt.Println(n5) |
||||
fmt.Println(n6) |
||||
fmt.Println(n7) |
||||
fmt.Println(n8) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/test$ go build |
||||
student@ubuntu:~/piscine-go/test$ ./test |
||||
12345 |
||||
12345 |
||||
0 |
||||
0 |
||||
1234 |
||||
-1234 |
||||
0 |
||||
0 |
||||
student@ubuntu:~/piscine-go/test$ |
||||
``` |
@ -1,19 +1,17 @@
|
||||
## firstruneprog |
||||
## firstrune |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that receives a `string` and returns the first `rune` of that `string`. |
||||
Write a function that returns the first `rune` of a `string`. |
||||
|
||||
### Expected output : |
||||
### Expected function |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/firstruneprog$ go build |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog "this is not happening" |
||||
t |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog "hello" | cat-e |
||||
h$ |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog "this" "is" "not" "happening" |
||||
student@ubuntu:~/piscine-go/firstruneprog$ |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog |
||||
student@ubuntu:~/piscine-go/firstruneprog$ |
||||
```go |
||||
func FirstRune(s string) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Note |
||||
|
||||
Remember to submit a main function testing the function |
@ -0,0 +1,51 @@
|
||||
<!DOCTYPE html> |
||||
<html> |
||||
<head> |
||||
<!--- This is the css used by for displaying gfm. ---> |
||||
<!--- Source ---> |
||||
<!--- https://github.com/sindresorhus/github-markdown-css ---> |
||||
<link rel="stylesheet" href="https://cdn.rawgit.com/mola-T/flymd/master/cdn/github-markdown_1_0_0.css"> |
||||
|
||||
<!--- My css file to format the page ---> |
||||
<link rel="stylesheet" href="https://cdn.rawgit.com/mola-T/flymd/master/cdn/flymd_1_1_0.css"> |
||||
|
||||
<!--- jQuery ---> |
||||
<script src="https://code.jquery.com/jquery-2.2.3.min.js" |
||||
integrity="sha256-a23g1Nt4dtEYOj7bR+vTu7+T8VP13humZFBJNIYoEJo=" |
||||
crossorigin="anonymous"></script> |
||||
|
||||
<!--- This is the script for converting md to html ---> |
||||
<!--- Source ---> |
||||
<!--- https://github.com/showdownjs/showdown ---> |
||||
<script type="text/javascript" |
||||
src="https://cdn.rawgit.com/showdownjs/showdown/1.3.0/dist/showdown.min.js"></script> |
||||
|
||||
<!--- My js file. ---> |
||||
<script type="text/javascript" |
||||
src="https://cdn.rawgit.com/mola-T/flymd/master/cdn/flymd_1_3_0.js"></script> |
||||
</head> |
||||
|
||||
<body> |
||||
<div class="flymd-static"> |
||||
<ul> |
||||
<li><input type="button" id="GFMize" value="GFMize"/></li> |
||||
<li><input type="button" id="MathJaxize" value="MathJaxize"/></li> |
||||
<li><input type="button" id="GFMmode" value="GFM Mode"/></li> |
||||
<li><input type="button" id="AutoRefresh" value="Auto Refresh"/></li> |
||||
<li><input type="button" id="AutoScroll" value="Auto Scroll"/></li> |
||||
</ul> |
||||
</div> |
||||
|
||||
<article class="markdown-body"> |
||||
<span id="replacer"> |
||||
<h1>If you see this page, you are so lucky.......</h1> |
||||
<p>Hello, I am Mola-T.</p> |
||||
<p>You can find me at <a href="mailto:mola@molamola.xyz">mola@molamola.xyz</a>.</p> |
||||
<p>It is the github page of this project <a href="https://github.com/mola-T/flymd">https://github.com/mola-T/flymd</a>.</p> |
||||
<p>If you see this page, it is most possibly due to browser compatibility problem.</p> |
||||
<p>You can check for the solution here:</p> |
||||
<h3><a href="https://github.com/mola-T/flymd/blob/master/browser.md">https://github.com/mola-T/flymd/blob/master/browser.md</a></h3> |
||||
</span> |
||||
</article> |
||||
</body> |
||||
</html> |
@ -0,0 +1,40 @@
|
||||
## maxfLyMd-mAkEr |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, `Max`, that returns the maximum value in a slice of integers. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Max(arr []int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
arrInt := []int{23, 123, 1, 11, 55, 93} |
||||
max := Max(arrInt) |
||||
fmt.Println(max |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
123 |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -0,0 +1,39 @@
|
||||
## foreach |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ForEach` that, for an `int` array, applies a function on each elements of that array. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func ForEach(f func(int), arr []int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
arr := []int{1, 2, 3, 4, 5, 6} |
||||
ForEach(PrintNbr, arr) |
||||
} |
||||
|
||||
func PrintNbr(int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
123456 |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -1,39 +0,0 @@
|
||||
## itoabaseprog |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that: |
||||
|
||||
- converts a number in base 10 into the number in the specified base |
||||
- receives two parameters: |
||||
- the first is the value |
||||
- the second is the base |
||||
|
||||
The base is expressed as an `int`, from 2 to 16. The characters comprising |
||||
the base are the digits from 0 to 9, followed by uppercase letters from A to F. |
||||
|
||||
For example, the base `4` would be the equivalent of "0123" and the base `16` would be the equivalent of "0123456789ABCDEF". |
||||
|
||||
If the value is negative, the resulting `string` has to be preceded with a |
||||
minus sign `-`. |
||||
|
||||
### Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ go build |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 15 16 |
||||
F |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 255 2 |
||||
11111111 |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog -4 2 | cat -e |
||||
-100$ |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog -df sdf |
||||
The value "-df" can not be converted to an int |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 23 ew | cat -e |
||||
The value "ew" can not be converted to an int$ |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 4 23 |
||||
|
||||
student@ubuntu:~/piscine-go/itoabaseprog$ 323 12 |
||||
22B |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ |
||||
``` |
@ -1,37 +0,0 @@
|
||||
## itoabaseprog |
||||
|
||||
### Instructions |
||||
|
||||
Écrire un programme qui: |
||||
|
||||
- convertit un nombre en base 10 en nombre dans la base spécifiée |
||||
- reçoit deux paramètres: |
||||
- le premier est la valeur |
||||
- le deuxième est la base |
||||
|
||||
Cette base est exprimée comme un `int`, de 2 à 16. Les caractères compris dans la base sont les chiffres de 0 à 9, suivis des lettres majuscules de A à F. |
||||
|
||||
Par exemple, la base `4` sera équivalente à "0123" et la base `16` sera équivalente à "0123456789ABCDEF". |
||||
|
||||
Si la valeur est négative, la `string` résultante doit être précédée d'un signe moins `-`. |
||||
|
||||
### Utilisation |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ go build |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 15 16 |
||||
F |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 255 2 |
||||
11111111 |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog -4 2 | cat -e |
||||
-100$ |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog -df sdf |
||||
The value "-df" can not be converted to an int |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 23 ew | cat -e |
||||
The value "ew" can not be converted to an int$ |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ ./itoabaseprog 4 23 |
||||
|
||||
student@ubuntu:~/piscine-go/itoabaseprog$ 323 12 |
||||
22B |
||||
student@ubuntu:~/piscine-go/itoabaseprog$ |
||||
``` |
@ -0,0 +1,15 @@
|
||||
## itoa |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that simulates the behaviour of the `Itoa` function in Go. `Itoa` transforms a number represented as an`int` in a number represented as a `string`. |
||||
|
||||
- For this exercise the handling of the signs + or - **does have** to be taken into account. |
||||
|
||||
## Expected function |
||||
|
||||
```go |
||||
func Itoa(n int) string { |
||||
|
||||
} |
||||
``` |
@ -1,19 +1,17 @@
|
||||
## lastruneprog |
||||
## lastrune |
||||
|
||||
### Instructions |
||||
|
||||
Write a program that receives a `string` and returns the last `rune` of a `string`. |
||||
Write a function that returns the last `rune` of a `string`. |
||||
|
||||
### Expected output : |
||||
### Expected function |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/firstruneprog$ go build |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog "this is not happening" |
||||
g |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog "hello" | cat -e |
||||
o$ |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog "this" "is" "not" "happening" |
||||
student@ubuntu:~/piscine-go/firstruneprog$ |
||||
student@ubuntu:~/piscine-go/firstruneprog$ ./firstruneprog |
||||
student@ubuntu:~/piscine-go/firstruneprog$ |
||||
```go |
||||
func LastRune(s string) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Note |
||||
|
||||
Remember to submit a main function testing the function |
@ -0,0 +1,40 @@
|
||||
## max |
||||
|
||||
### Instructions |
||||
|
||||
Write a function, `Max`, that returns the maximum value in a slice of integers. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Max(arr []int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
arrInt := []int{23, 123, 1, 11, 55, 93} |
||||
max := Max(arrInt) |
||||
fmt.Println(max |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
123 |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -1,22 +1,17 @@
|
||||
## nruneprog |
||||
## nrune |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the nth `rune` of a `string`. |
||||
|
||||
### Expected output : |
||||
### Expected function |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine-go/nruneprog$ ./nruneprog "hello" 2 |
||||
e |
||||
student@ubuntu:~/piscine-go/nruneprog$ ./nruneprog "hello" 4 | cat -e |
||||
l$ |
||||
student@ubuntu:~/piscine-go/nruneprog$ ./nruneprog "hello" 5 |
||||
o |
||||
student@ubuntu:~/piscine-go/nruneprog$ ./nruneprog "hello" |
||||
student@ubuntu:~/piscine-go/nruneprog$ ./nruneprog "hello" f | cat -e |
||||
"f" is not an integer value$ |
||||
student@ubuntu:~/piscine-go/nruneprog$ ./nruneprog "hello" 9 |
||||
Invalid position: "9" in "hello" |
||||
student@ubuntu:~/piscine-go/nruneprog$ |
||||
```go |
||||
func NRune(s string, n int) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Note |
||||
|
||||
Remember to submit a main function testing the function |
@ -0,0 +1,42 @@
|
||||
## sortwordarr |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `SortWordArr` that sorts by `ascii` (in ascending order) a `string` array. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func SortWordArr(array []string) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
|
||||
result := []string{"a", "A", "1", "b", "B", "2", "c", "C", "3"} |
||||
SortWordArr(result) |
||||
|
||||
fmt.Println(result) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
[1 2 3 A B C a b c] |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -0,0 +1,42 @@
|
||||
## sortwordarr |
||||
|
||||
### Instructions |
||||
|
||||
Écrire une fonction `SortWordArr` qui trie par ordre `ascii` (dans l'ordre croissant) un tableau de `string`. |
||||
|
||||
### Fonction attendue |
||||
|
||||
```go |
||||
func SortWordArr(array []string) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Utilisation |
||||
|
||||
Voici un éventuel [programme](TODO-LINK) pour tester votre fonction : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
|
||||
result := []string{"a", "A", "1", "b", "B", "2", "c", "C", "3"} |
||||
SortWordArr(result) |
||||
|
||||
fmt.Println(result) |
||||
} |
||||
``` |
||||
|
||||
Et son résultat : |
||||
|
||||
```console |
||||
student@ubuntu:~/test$ go build |
||||
student@ubuntu:~/test$ ./test |
||||
[1 2 3 A B C a b c] |
||||
student@ubuntu:~/test$ |
||||
``` |
@ -0,0 +1,17 @@
|
||||
## swap |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that swaps the contents of two **pointers to an int** (`*int`). |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Swap(a *int, b *int) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Note |
||||
|
||||
Remember to submit a main function testing the function |
Loading…
Reference in new issue