forked from root/public
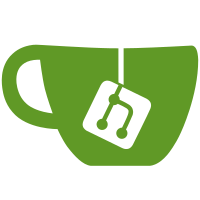
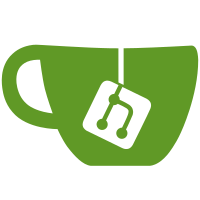
31 changed files with 120 additions and 50 deletions
@ -0,0 +1,35 @@
|
||||
## free-project |
||||
|
||||
### Instructions |
||||
|
||||
The free project is the opportunity to show what you have learned by making your |
||||
own webpage. |
||||
|
||||
You need to submit at least one `index.html` page, have some styling in a |
||||
`style.css` file and add a a `script.js` file, all of them must be properly |
||||
linked and used. |
||||
|
||||
Focus on building something that interest you, anything you want as long as you |
||||
use the given files for it: |
||||
|
||||
- Interactive trivia pages of something you love |
||||
- Business oriented ? Build your own branding with your online CV |
||||
- Artistic soul ? Make your own generative art |
||||
- You have a story to tell ? Make a point and click adventure |
||||
- Looking for a fun but ambitious idea ? Try a basic game (lots of JS) |
||||
|
||||
**Don't hesitate to use the code from the previous raid to get a head start.** |
||||
|
||||
> Not inspired ? Just focus on improving your previous raid: |
||||
> |
||||
> - Customize the colors |
||||
> - Change the subject |
||||
> - Add a new section, pictures |
||||
> - Do all the bonuses |
||||
> - Make sections collapsable |
||||
|
||||
### Extra |
||||
|
||||
If you want to go the extra mile here is to way to gain extra credits: |
||||
- Responsive (Make your web page work on mobile and desktop) |
||||
- Publish It ! (Use a service, like [github pages](https://pages.github.com/) or [cloudflare pages](https://pages.cloudflare.com/) to host your page, it's free) |
@ -0,0 +1,13 @@
|
||||
#### Required |
||||
|
||||
###### Is the `index.html` webpage displayed properly ? |
||||
|
||||
###### Is css applied from the `style.css` file ? |
||||
|
||||
###### Are scripts loaded from `script.js` file and no errors shown in the console ? |
||||
|
||||
#### Optional |
||||
|
||||
###### +Did you use a service to publish it ? (github pages or equivalent) |
||||
|
||||
###### +Does the webpage work on multiple window size ? (phone, tablets, computers...) |
Loading…
Reference in new issue