forked from root/public
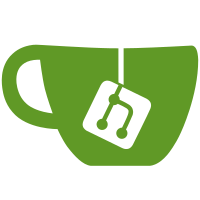
1 changed files with 137 additions and 29 deletions
@ -1,49 +1,157 @@
|
||||
# Student |
||||
## Student |
||||
|
||||
### Instructions |
||||
|
||||
Create a class named `Student` that extends class named `Person` that you have previously created. |
||||
Create a `class` named `Student`, that extends the `Person` `class` that you created earlier. |
||||
|
||||
Its attributes: |
||||
Attributes: |
||||
|
||||
- batch - `public integer` |
||||
- level - `public integer` |
||||
- secretKey - `private string`, by default equal to "01". |
||||
- Constructor: |
||||
- name - `required string` |
||||
- cityOfOrigin - `required string` |
||||
- age - `required int` |
||||
- height - `required int` |
||||
- batch - `required int` |
||||
- level - `required int` |
||||
- `batch`: `int` |
||||
- `level`: `int` |
||||
- `secretKey`: private `string`. Defaults to "01". |
||||
|
||||
Constructor: |
||||
- `name`: `string` |
||||
- `cityOfOrigin`: `string` |
||||
- `age`: `int` |
||||
- `height`: `int` |
||||
- `batch`: `int` |
||||
- `level`: `int` |
||||
|
||||
### Inheritance |
||||
|
||||
Classes can inherit other classes methods and fields, by doing so, every public field and method will be visible to the child class. It can be useful when some class is completely in the set of other class and you don't want to copy all the code you wrote for another class. If necessary, behavior of certain methods can be changed in the inferior class by "@override" command. |
||||
A class can inherit the fields and methods from another class. By doing so, every public field and method will be visible to the child class. It can be useful in cases where one class is a superset of the other, and you feel that it is not useful to copy all of the code from one class to another. If necessary, the behavior of certain methods can be changed in the child class by using the `@override` keyword. |
||||
|
||||
Let's say we have a class `TV` with a lot of code. Then the class `SmartTV` can have all of the features of `TV` plus its own smart features. |
||||
|
||||
```dart |
||||
class TV { |
||||
void turnOn() { |
||||
_illuminateDisplay(); |
||||
_activateIrSensor(); |
||||
bool _hasPower = false; |
||||
|
||||
int _channel = 1; |
||||
int _minChannel = 1; |
||||
int _maxChannel = 99; |
||||
|
||||
bool _isMute = false; |
||||
int _volume = 50; |
||||
int _minVolume = 0; |
||||
int _maxVolume = 100; |
||||
|
||||
void connectPower() { |
||||
this._hasPower = true; |
||||
} |
||||
|
||||
void disconnectPower() { |
||||
this._hasPower = false; |
||||
this._channel = 1; |
||||
this._volume = 50; |
||||
this._isMute = false; |
||||
} |
||||
|
||||
void toggleMute() { |
||||
this._isMute = !this._isMute; |
||||
} |
||||
|
||||
bool get mute => this._isMute; |
||||
|
||||
int get volume => (this._isMute) ? 0 : this._volume; |
||||
|
||||
int get channel => this._channel; |
||||
|
||||
bool get hasPower => this._hasPower; |
||||
|
||||
set channel(int channelNum) { |
||||
this._channel = |
||||
(channelNum >= this._maxChannel || channelNum <= this._minChannel) |
||||
? this._channel |
||||
: channelNum; |
||||
} |
||||
|
||||
void increaseVolume() { |
||||
this._isMute = false; |
||||
if (this._volume <= this._maxVolume) { |
||||
this._volume++; |
||||
} |
||||
} |
||||
|
||||
void decrementVolume() { |
||||
this._isMute = false; |
||||
|
||||
this._volume = |
||||
(this._volume <= this._minVolume) ? this._minVolume : this._volume - 1; |
||||
} |
||||
|
||||
void incrementVolume() { |
||||
this._isMute = false; |
||||
|
||||
this._volume = |
||||
(this._volume >= this._maxVolume) ? this._maxVolume : this._volume + 1; |
||||
} |
||||
|
||||
void decrementChannel() { |
||||
this._isMute = false; |
||||
|
||||
this._channel = (this._channel <= this._minChannel) |
||||
? this._minChannel |
||||
: this._channel - 1; |
||||
} |
||||
|
||||
void incrementChannel() { |
||||
this._isMute = false; |
||||
|
||||
this._channel = (this._channel >= this._maxChannel) |
||||
? this._maxChannel |
||||
: this._channel + 1; |
||||
} |
||||
|
||||
TV({required bool hasPower}) { |
||||
this._hasPower = hasPower; |
||||
} |
||||
// ··· |
||||
} |
||||
``` |
||||
|
||||
`SmartTV` can be created with **much** less code, and still have all of the base functionality of `TV`. |
||||
|
||||
Notice that `@override` changes the behavior of the `disconnectPower` method. |
||||
|
||||
> If you override a method, you can still use the method from the parent class by writing `super.` before invoking the method: `super.disconnectPower()`. |
||||
|
||||
```dart |
||||
class SmartTV extends TV { |
||||
@override |
||||
void turnOn() { |
||||
super.turnOn(); |
||||
_bootNetworkInterface(); |
||||
_initializeMemory(); |
||||
_upgradeApps(); |
||||
} |
||||
// ··· |
||||
String? _app; |
||||
|
||||
void startApp(String app) => this._app = app; |
||||
void quitApp() => this._app = null; |
||||
|
||||
bool get tvMode => this._app == null; |
||||
String? get app => this._app; |
||||
|
||||
@override |
||||
void disconnectPower() { |
||||
super.disconnectPower(); |
||||
this._app = null; |
||||
} |
||||
|
||||
SmartTV({required bool hasPower}) : super(hasPower: hasPower); |
||||
} |
||||
``` |
||||
|
||||
We know that every SmartTV is a TV, therefore we can extend all the methods and fields of the TV class to the SmartTV class. |
||||
```dart |
||||
void main() { |
||||
var tv = new SmartTV(hasPower: true); |
||||
|
||||
print(tv.volume); // 50 |
||||
tv.toggleMute(); |
||||
print(tv.volume); // 0 |
||||
tv.increaseVolume(); |
||||
print(tv.volume); // 51 |
||||
|
||||
By writing `@override`, we are overriding the behavior of the parent class, so that it meets our needs, and if we want to call the parent classes method, we simply put `super` before the name of the method. |
||||
tv.startApp('Chrome'); |
||||
print(tv.tvMode); // true |
||||
tv.quitApp(); |
||||
print(tv.tvMode); // false |
||||
} |
||||
|
||||
``` |
||||
|
||||
- Note: Use the constructor of parent class. |
||||
> Don't forget to use the constructor of the parent class. |
||||
|
Loading…
Reference in new issue