forked from root/public
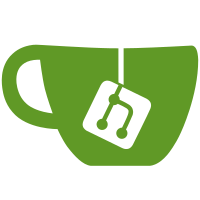
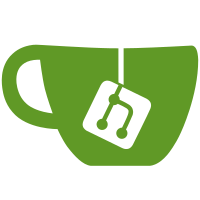
11 changed files with 583 additions and 0 deletions
@ -0,0 +1,143 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"strings" |
||||
"testing" |
||||
|
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func challengeProgram(t *testing.T, stuPath, solPath string, args ...string) { |
||||
exercise := strings.ToLower( |
||||
strings.TrimPrefix(t.Name(), "Test")) |
||||
out, err := z01.MainOut(stuPath+exercise, args...) |
||||
if err != nil { |
||||
t.Error(err) |
||||
} |
||||
correct, err := z01.MainOut(solPath+exercise, args...) |
||||
if err != nil { |
||||
t.Error(err) |
||||
} |
||||
if out != correct { |
||||
t.Errorf("./%s %s prints %q instead of %q\n", |
||||
exercise, strings.Join(args, " "), out, correct) |
||||
} |
||||
} |
||||
|
||||
func TestFlags(t *testing.T) { |
||||
argsAndSolution := map[string]string{ |
||||
"tests/alphacount.go": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import regexp tests/alphacount.go:4:2 |
||||
illegal-access regexp.MustCompile tests/alphacount.go:8:8 |
||||
illegal-call len tests/alphacount.go:10:9 |
||||
illegal-call AlphaCount tests/alphacount.go:7:1 |
||||
`, |
||||
|
||||
"-no-for -no-lit=[a-z] tests/printalphabet/printalphabet.go": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import github.com/01-edu/z01 tests/printalphabet/printalphabet.go:4:2 |
||||
illegal-call append tests/printalphabet/printalphabet.go:9:7 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:15:2 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:7:1 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:17:3 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:19:2 |
||||
illegal-call main tests/printalphabet/printalphabet.go:13:1 |
||||
illegal-literal 'a' tests/printalphabet/printalphabet.go:8:11 |
||||
illegal-literal 'z' tests/printalphabet/printalphabet.go:8:21 |
||||
illegal-literal '\n' tests/printalphabet/printalphabet.go:19:16 |
||||
illegal-literal 'a' tests/printalphabet/printalphabet.go:8:11 |
||||
illegal-literal 'z' tests/printalphabet/printalphabet.go:8:21 |
||||
illegal-literal 'a' tests/printalphabet/printalphabet.go:8:11 |
||||
illegal-literal 'z' tests/printalphabet/printalphabet.go:8:21 |
||||
illegal-loop for tests/printalphabet/printalphabet.go:8:2 |
||||
illegal-loop for tests/printalphabet/printalphabet.go:8:2 |
||||
illegal-loop for tests/printalphabet/printalphabet.go:8:2 |
||||
`, |
||||
"tests/eightqueens.go": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import github.com/01-edu/z01 tests/eightqueens.go:4:2 |
||||
illegal-access z01.PrintRune tests/eightqueens.go:49:5 |
||||
illegal-access z01.PrintRune tests/eightqueens.go:55:2 |
||||
illegal-call rune tests/eightqueens.go:49:19 |
||||
illegal-call printQueens tests/eightqueens.go:70:5 |
||||
illegal-call printQueens tests/eightqueens.go:42:1 |
||||
illegal-call tryX tests/eightqueens.go:73:5 |
||||
illegal-call tryX tests/eightqueens.go:85:2 |
||||
illegal-call tryX tests/eightqueens.go:60:1 |
||||
illegal-call EightQueens tests/eightqueens.go:83:1 |
||||
`, |
||||
"-cast tests/eightqueens.go": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import github.com/01-edu/z01 tests/eightqueens.go:4:2 |
||||
illegal-access z01.PrintRune tests/eightqueens.go:49:5 |
||||
illegal-access z01.PrintRune tests/eightqueens.go:55:2 |
||||
`, |
||||
"-no-arrays tests/printalphabet/printalphabet.go ": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import github.com/01-edu/z01 tests/printalphabet/printalphabet.go:4:2 |
||||
illegal-call append tests/printalphabet/printalphabet.go:9:7 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:15:2 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:7:1 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:17:3 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:19:2 |
||||
illegal-call main tests/printalphabet/printalphabet.go:13:1 |
||||
illegal-array-type rune tests/printalphabet/printalphabet.go:14:8 |
||||
`, |
||||
"-no-these-arrays rune tests/printalphabet/printalphabet.go ": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import github.com/01-edu/z01 tests/printalphabet/printalphabet.go:4:2 |
||||
illegal-call append tests/printalphabet/printalphabet.go:9:7 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:15:2 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:7:1 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:17:3 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:19:2 |
||||
illegal-call main tests/printalphabet/printalphabet.go:13:1 |
||||
illegal-array-type rune tests/printalphabet/printalphabet.go:14:8 |
||||
`, |
||||
`-no-these-arrays "int" tests/printalphabet/printalphabet.go`: `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-import github.com/01-edu/z01 tests/printalphabet/printalphabet.go:4:2 |
||||
illegal-call append tests/printalphabet/printalphabet.go:9:7 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:15:2 |
||||
illegal-call fillArray tests/printalphabet/printalphabet.go:7:1 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:17:3 |
||||
illegal-access z01.PrintRune tests/printalphabet/printalphabet.go:19:2 |
||||
illegal-call main tests/printalphabet/printalphabet.go:13:1 |
||||
`, |
||||
} |
||||
|
||||
Compare(t, argsAndSolution) |
||||
} |
||||
|
||||
func Compare(t *testing.T, argsAndSol map[string]string) { |
||||
for args, sol := range argsAndSol { |
||||
out, err := z01.MainOut("../rc", strings.Split(args, " ")...) |
||||
if err.Error() != sol && out != sol { |
||||
fmt.Println("Error:", err) |
||||
fmt.Println("Out:", out) |
||||
t.Errorf("./rc %s prints %q\n instead of %q\n", args, out, sol) |
||||
} |
||||
} |
||||
} |
||||
|
||||
func TestWrapping(t *testing.T) { |
||||
argsAndSolution := map[string]string{ |
||||
"tests/testingWrapping.go ./util": `Parsing |
||||
OK |
||||
Cheating |
||||
illegal-call len tests/util/util.go:6:9 |
||||
illegal-call util.LenWrapper tests/util/util.go:5:1 |
||||
illegal-call Length tests/testingWrapping.go:7:1 |
||||
`, |
||||
} |
||||
Compare(t, argsAndSolution) |
||||
} |
@ -0,0 +1,214 @@
|
||||
// package main
|
||||
|
||||
// import (
|
||||
// "fmt"
|
||||
// "os"
|
||||
// "strconv"
|
||||
// )
|
||||
|
||||
// level 3: doopprog
|
||||
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"os" |
||||
"strconv" |
||||
) |
||||
|
||||
func main() { |
||||
args := os.Args |
||||
|
||||
if len(args) != 4 { |
||||
return |
||||
} |
||||
|
||||
if args[2] != "+" && args[2] != "-" && args[2] != "/" && args[2] != "*" && args[2] != "%" { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
|
||||
// arg1 := getArg(args[1])
|
||||
|
||||
arg1, err := strconv.Atoi(args[1]) |
||||
if err != nil { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
//fmt.Println("Arg1: ", arg1)
|
||||
|
||||
arg2, err := strconv.Atoi(args[3]) |
||||
if err != nil { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
//fmt.Println("Arg2: ", arg2)
|
||||
|
||||
if args[2] == "+" { |
||||
Add(int64(arg1), int64(arg2)) |
||||
} else if args[2] == "-" { |
||||
Subs(int64(arg1), int64(arg2)) |
||||
} else if args[2] == "/" { |
||||
Div(int64(arg1), int64(arg2)) |
||||
} else if args[2] == "*" { |
||||
Multip(int64(arg1), int64(arg2)) |
||||
} else if args[2] == "%" { |
||||
Modulo(int64(arg1), int64(arg2)) |
||||
} |
||||
|
||||
} |
||||
|
||||
var max int64 = 9223372036854775807 |
||||
var min int64 = -9223372036854775808 |
||||
|
||||
func Add(x, y int64) { |
||||
if x >= 0 && y < 0 { |
||||
Subs(x, -y) |
||||
return |
||||
} else if x < 0 && y >= 0 { |
||||
Subs(y, -x) |
||||
return |
||||
} else if x < 0 && y < 0 { |
||||
if x >= min-y { |
||||
fmt.Println(x + y) |
||||
return |
||||
} else { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
} |
||||
|
||||
if x <= max-y { |
||||
fmt.Println(x + y) |
||||
} else { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
} |
||||
|
||||
func Subs(x, y int64) { |
||||
if x >= 0 && y < 0 { |
||||
Add(x, -y) |
||||
return |
||||
} else if x < 0 && y >= 0 { |
||||
Add(-y, x) |
||||
return |
||||
} else if x < 0 && y < 0 { |
||||
Subs(-y, -x) |
||||
return |
||||
} |
||||
|
||||
if x >= min+y { |
||||
fmt.Println(x - y) |
||||
return |
||||
} else { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
} |
||||
|
||||
func Multip(x, y int64) { |
||||
if x >= 0 && y >= 0 { |
||||
if x <= max/y { |
||||
fmt.Println(x * y) |
||||
return |
||||
} else { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
} else if x < 0 && y < 0 { |
||||
if x > max/y { |
||||
fmt.Println(x * y) |
||||
return |
||||
} else { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
} else { |
||||
if x >= min/y { |
||||
fmt.Println(x * y) |
||||
return |
||||
} else { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
} |
||||
} |
||||
|
||||
func Div(x, y int64) { |
||||
if y == 0 { |
||||
fmt.Println("No division by 0") |
||||
return |
||||
} |
||||
if x == min && y == -1 { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
fmt.Println(x / y) |
||||
return |
||||
} |
||||
|
||||
func Modulo(x, y int64) { |
||||
if y == 0 { |
||||
fmt.Println("No modulo by 0") |
||||
return |
||||
} |
||||
if y == min && x == -1 { |
||||
fmt.Println(0) |
||||
return |
||||
} |
||||
fmt.Println(x % y) |
||||
return |
||||
} |
||||
|
||||
//9223372036854775807
|
||||
// func main() {
|
||||
// if len(os.Args) == 4 {
|
||||
// var result int
|
||||
// firstArg, err := strconv.Atoi(os.Args[1])
|
||||
|
||||
// if err != nil {
|
||||
// fmt.Println(0)
|
||||
// return
|
||||
// }
|
||||
|
||||
// operator := os.Args[2]
|
||||
// secondArg, err1 := strconv.Atoi(os.Args[3])
|
||||
|
||||
// if err1 != nil {
|
||||
// fmt.Println(0)
|
||||
// return
|
||||
// }
|
||||
|
||||
// if secondArg == 0 && operator == "/" {
|
||||
// fmt.Println("No division by 0")
|
||||
// return
|
||||
// } else if secondArg == 0 && operator == "%" {
|
||||
// fmt.Println("No modulo by 0")
|
||||
// return
|
||||
// } else if operator == "+" {
|
||||
// result = firstArg + secondArg
|
||||
// if !((result > firstArg) == (secondArg > 0)) {
|
||||
// fmt.Println(0)
|
||||
// return
|
||||
// }
|
||||
// } else if operator == "-" {
|
||||
// result = firstArg - secondArg
|
||||
// if !((result < firstArg) == (secondArg > 0)) {
|
||||
// fmt.Println(0)
|
||||
// return
|
||||
// }
|
||||
// } else if operator == "/" {
|
||||
// result = firstArg / secondArg
|
||||
// } else if operator == "*" {
|
||||
// result = firstArg * secondArg
|
||||
// if firstArg != 0 && (result/firstArg != secondArg) {
|
||||
// fmt.Println(0)
|
||||
// return
|
||||
// }
|
||||
// } else if operator == "%" {
|
||||
// result = firstArg % secondArg
|
||||
// }
|
||||
// fmt.Println(result)
|
||||
// }
|
||||
// }
|
@ -0,0 +1,86 @@
|
||||
package solutions |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
const size = 8 |
||||
|
||||
// board is a chessboard composed of boolean squares, a true square means a queen is on it
|
||||
// a false square means it is a free square
|
||||
var board [size][size]bool |
||||
|
||||
// goodDirection check that there is no queen on the segment that starts at (x, y)
|
||||
// coordinates, points into the direction vector (vx, vy) and ends at the edge of the board
|
||||
func goodDirection(x, y, vx, vy int) bool { |
||||
// x and y are still on board
|
||||
for 0 <= x && x < size && |
||||
0 <= y && y < size { |
||||
if board[x][y] { |
||||
// Not a good line : the square is already occupied
|
||||
return false |
||||
} |
||||
x = x + vx // Move x in the right direction
|
||||
y = y + vy // Move y in the right direction
|
||||
} |
||||
// All clear
|
||||
return true |
||||
} |
||||
|
||||
// goodSquare makes all the necessary line checks for the queens movements
|
||||
func goodSquare(x, y int) bool { |
||||
return goodDirection(x, y, +0, -1) && |
||||
goodDirection(x, y, +1, -1) && |
||||
goodDirection(x, y, +1, +0) && |
||||
goodDirection(x, y, +1, +1) && |
||||
goodDirection(x, y, +0, +1) && |
||||
goodDirection(x, y, -1, +1) && |
||||
goodDirection(x, y, -1, +0) && |
||||
goodDirection(x, y, -1, -1) |
||||
} |
||||
|
||||
func printQueens() { |
||||
x := 0 |
||||
for x < size { |
||||
y := 0 |
||||
for y < size { |
||||
if board[x][y] { |
||||
// We have found a queen, let's print her y
|
||||
z01.PrintRune(rune(y) + '1') |
||||
} |
||||
y++ |
||||
} |
||||
x++ |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
// tryX tries, for a given x (column) to find a y (row) so that the queen on (x, y) is a part
|
||||
// of the solution to the problem
|
||||
func tryX(x int) { |
||||
y := 0 |
||||
for y < size { |
||||
if goodSquare(x, y) { |
||||
// Since the square is good for the queen, let's put one on it:
|
||||
board[x][y] = true |
||||
|
||||
if x == size-1 { |
||||
// x is the biggest possible x, it means that we just placed the last
|
||||
// queen on the board, so the solution is complete and we can print it
|
||||
printQueens() |
||||
} else { |
||||
// let's try to put another queen on the next empty x (column)
|
||||
tryX(x + 1) |
||||
} |
||||
|
||||
// remove the queen of the board, to try other y values
|
||||
board[x][y] = false |
||||
} |
||||
y++ |
||||
} |
||||
} |
||||
|
||||
func EightQueens() { |
||||
// try the first column
|
||||
tryX(0) |
||||
} |
@ -0,0 +1,25 @@
|
||||
package solutions |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func thisIsAFunc() { |
||||
fmt.Println("This is a function") |
||||
} |
||||
|
||||
var ThisToo = func(s string) { |
||||
fmt.Printf("ThisToo prints %s\n", s) |
||||
} |
||||
|
||||
func aux(s string) int { |
||||
return 1 |
||||
} |
||||
|
||||
func youCanAlso(f func(string), s string) { |
||||
aux := func(s string) int { |
||||
return len(s) |
||||
} |
||||
aux(s) |
||||
f(s) |
||||
} |
@ -0,0 +1,16 @@
|
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func myFunction(s string) bool { |
||||
return s > "m" |
||||
} |
||||
|
||||
func main() { |
||||
// myFunction := func(s string) bool {
|
||||
// return s < "m"
|
||||
// }
|
||||
|
||||
fmt.Printf("Does %s comes before \"m\" %v\n", "name", myFunction("name")) |
||||
fmt.Printf("Does %s comes before \"m\" %v\n", "change", myFunction("change")) |
||||
} |
@ -0,0 +1,29 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
|
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func fillArray(a []rune) { |
||||
for i := 'a'; i <= 'z'; i++ { |
||||
a = append(a, i) |
||||
} |
||||
} |
||||
|
||||
func main() { |
||||
a := []rune{'a', 'b', 'c', 'd', 'e', 'f'} |
||||
b := int('a') |
||||
for _, v := range a { |
||||
z01.PrintRune(v) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
func testingScope() { |
||||
defFun := func(s string) { |
||||
fmt.Println(s) |
||||
} |
||||
defFun("Hello") |
||||
} |
@ -0,0 +1,19 @@
|
||||
package main |
||||
|
||||
import "github.com/01-edu/z01" |
||||
|
||||
func main() { |
||||
z01.PrintRune('a') |
||||
z01.PrintRune('b') |
||||
z01.PrintRune('c') |
||||
z01.PrintRune('d') |
||||
z01.PrintRune('e') |
||||
z01.PrintRune('f') |
||||
z01.PrintRune('g') |
||||
z01.PrintRune('h') |
||||
z01.PrintRune('i') |
||||
z01.PrintRune('j') |
||||
z01.PrintRune('k') |
||||
z01.PrintRune('l') |
||||
z01.PrintRune('m') |
||||
} |
@ -0,0 +1,11 @@
|
||||
package solutions |
||||
|
||||
import ( |
||||
"regexp" |
||||
) |
||||
|
||||
func SimpleFunc(str string) int { |
||||
re := regexp.MustCompile(`[a-zA-Z]`) |
||||
found := re.FindAll([]byte(str), -1) |
||||
return len(found) |
||||
} |
@ -0,0 +1,12 @@
|
||||
package solutions |
||||
|
||||
import ( |
||||
util "./util" |
||||
) |
||||
|
||||
func Length(ss []string) int { |
||||
return util.LenWrapperU(ss) |
||||
} |
||||
|
||||
// func NotUsed() {
|
||||
// }
|
@ -0,0 +1,23 @@
|
||||
package util |
||||
|
||||
import ( |
||||
"fmt" |
||||
|
||||
util2 "../utilDepth2" |
||||
) |
||||
|
||||
func LenWrapperU(ss []string) int { |
||||
return util2.LenWrapper(ss) |
||||
} |
||||
|
||||
func NotUsed() { |
||||
b := []string{"just", "something"} |
||||
a := len(b) |
||||
for i, v := range b { |
||||
if i == a-1 { |
||||
fmt.Println("Last element", v) |
||||
continue |
||||
} |
||||
fmt.Println("Element", v) |
||||
} |
||||
} |
@ -0,0 +1,5 @@
|
||||
package util |
||||
|
||||
func LenWrapper(ss []string) int { |
||||
return len(ss) |
||||
} |
Loading…
Reference in new issue