forked from root/public
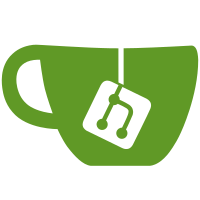
4 changed files with 142 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "borrow" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "borrow_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"borrow", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "borrow_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
borrow = { path = "../../../../rust-piscine-solutions/borrow"} |
@ -0,0 +1,85 @@
|
||||
/* |
||||
## borrow |
||||
|
||||
### Instructions |
||||
|
||||
Complete the signature and the body of the `str_len` function so it |
||||
receives a string or a string literal and returns its length (of type usize) |
||||
without taking ownership of the value (i.e, borrowing the value) |
||||
|
||||
### Example |
||||
|
||||
```rust |
||||
fn main() { |
||||
let s = "hello"; |
||||
let s1 = "camelCase".to_string(); |
||||
|
||||
println!("\tstr_len(\"{}\") = {}", s, str_len(s)); |
||||
println!("\tstr_len(\"{}\") = {}", s1, str_len(&s1)); |
||||
} |
||||
``` |
||||
*/ |
||||
|
||||
use borrow::*; |
||||
|
||||
fn main() { |
||||
let s = "hello"; |
||||
let s1 = "camelCase".to_string(); |
||||
|
||||
println!("\tstr_len(\"{}\") = {}", s, str_len(s)); |
||||
println!("\tstr_len(\"{}\") = {}", s1, str_len(&s1)); |
||||
} |
||||
|
||||
#[test] |
||||
// maybe not the best way to make the test, but I wanted to use
|
||||
// lifetimes
|
||||
fn str_len_test() { |
||||
struct TstLit<'a> { |
||||
str: &'a str, |
||||
l: usize, |
||||
} |
||||
|
||||
struct TstString { |
||||
str: String, |
||||
l: usize, |
||||
} |
||||
|
||||
let tsts = vec![ |
||||
TstLit { str: "hello", l: 5 }, |
||||
TstLit { str: "how", l: 3 }, |
||||
TstLit { |
||||
str: "are you", |
||||
l: 7, |
||||
}, |
||||
TstLit { |
||||
str: "change", |
||||
l: 6, |
||||
}, |
||||
]; |
||||
let o_tsts = vec![ |
||||
TstString { |
||||
str: "hello".to_string(), |
||||
l: 5, |
||||
}, |
||||
TstString { |
||||
str: "how".to_string(), |
||||
l: 3, |
||||
}, |
||||
TstString { |
||||
str: "are you".to_string(), |
||||
l: 7, |
||||
}, |
||||
TstString { |
||||
str: "change".to_string(), |
||||
l: 6, |
||||
}, |
||||
]; |
||||
|
||||
for t in tsts.iter() { |
||||
assert_eq!(t.l, str_len(t.str)); |
||||
} |
||||
|
||||
for t in o_tsts.iter() { |
||||
assert_eq!(t.l, str_len(&t.str)); |
||||
} |
||||
} |
@ -0,0 +1,35 @@
|
||||
## borrow |
||||
|
||||
### Instructions |
||||
|
||||
Complete the signature and the body of the `str_len` that receives a string or a string literal and returns its length without taking ownership of the value (i.e, borrowing the value) |
||||
|
||||
### Expected Function |
||||
|
||||
```rust |
||||
pub fn str_len(s: ) -> usize { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```rust |
||||
fn main() { |
||||
let s = "hello"; |
||||
let s1 = "camelCase".to_string(); |
||||
|
||||
println!("\tstr_len(\"{}\") = {}", s, str_len(s)); |
||||
println!("\tstr_len(\"{}\") = {}", s1, str_len(&s1)); |
||||
} |
||||
``` |
||||
|
||||
And its output: |
||||
|
||||
```rust |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
str_len("hello") = 5 |
||||
str_len("camelCase") = 9 |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue