forked from root/public
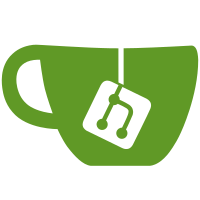
4 changed files with 160 additions and 0 deletions
@ -0,0 +1,12 @@
|
||||
# This file is automatically @generated by Cargo. |
||||
# It is not intended for manual editing. |
||||
[[package]] |
||||
name = "step_iterator" |
||||
version = "0.1.0" |
||||
|
||||
[[package]] |
||||
name = "step_iterator_test" |
||||
version = "0.1.0" |
||||
dependencies = [ |
||||
"step_iterator", |
||||
] |
@ -0,0 +1,10 @@
|
||||
[package] |
||||
name = "step_iterator_test" |
||||
version = "0.1.0" |
||||
authors = ["Augusto <aug.ornelas@gmail.com>"] |
||||
edition = "2018" |
||||
|
||||
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html |
||||
|
||||
[dependencies] |
||||
step_iterator = { path = "../../../../rust-piscine-solutions/step_iterator"} |
@ -0,0 +1,83 @@
|
||||
// Create an Iterator (by implementing the std::iter::Iterator trait)
|
||||
// that iterates through the values from beg to end (including end) in
|
||||
// the indicated steps
|
||||
|
||||
// The name of you're iterator will be StepIterator and it must be
|
||||
// generic so you can use any integer value: i8,..,i64 of floating
|
||||
// point number f32,..,f64
|
||||
|
||||
// Define the associated function: `new` that creates a new Step iterator:
|
||||
// fn new(beg: T, end: T, step: T) -> StepIterator
|
||||
|
||||
// For example:
|
||||
// for v in StepIterator::new(0, 100, 10) {
|
||||
// println!("{}", v);
|
||||
// }
|
||||
// must print:
|
||||
// 0
|
||||
// 10
|
||||
// 20
|
||||
// 30
|
||||
// 40
|
||||
// 50
|
||||
// 60
|
||||
// 70
|
||||
// 80
|
||||
// 90
|
||||
// 100
|
||||
|
||||
// If the steps don't allow to arrive until the end of the sequence
|
||||
// only the last value inferior than the end of the series will be returned
|
||||
// Like beg: 0, end: 100, steps: 7 the last number returned will be
|
||||
// the last number returned will be 98
|
||||
|
||||
use step_iterator::StepIterator; |
||||
|
||||
#[allow(dead_code)] |
||||
fn main() { |
||||
for v in StepIterator::new(0, 100, 10) { |
||||
print!("{},", v); |
||||
} |
||||
println!(); |
||||
|
||||
for v in StepIterator::new(0, 100, 12) { |
||||
print!("{},", v) |
||||
} |
||||
println!(); |
||||
} |
||||
|
||||
#[cfg(test)] |
||||
mod tests { |
||||
use super::*; |
||||
|
||||
#[test] |
||||
fn test_next() { |
||||
let mut step_iterator = StepIterator::new(0, 100, 10); |
||||
assert_eq!(step_iterator.next(), Some(0)); |
||||
assert_eq!(step_iterator.next(), Some(10)); |
||||
} |
||||
|
||||
#[test] |
||||
fn until_the_end() { |
||||
for (i, v) in StepIterator::new(0, 100, 10).enumerate() { |
||||
println!("position: {}, value: {}, ", i, v); |
||||
assert_eq!(i * 10, v); |
||||
} |
||||
} |
||||
|
||||
#[test] |
||||
fn test_with_floats() { |
||||
for (i, v) in StepIterator::new(0.0, 10.0, 0.5).enumerate() { |
||||
println!("position: {}, value: {}, ", i, v); |
||||
assert_eq!(i as f64 * 0.5, v); |
||||
} |
||||
} |
||||
|
||||
#[test] |
||||
fn test_with_floats_with_imperfect_range() { |
||||
for (i, v) in StepIterator::new(0.3, 10.0, 0.5).enumerate() { |
||||
println!("position: {}, value: {}, ", i, v); |
||||
assert_eq!(i as f64 * 0.5 + 0.3, v); |
||||
} |
||||
} |
||||
} |
@ -0,0 +1,55 @@
|
||||
## step_iterator |
||||
|
||||
### Instructions |
||||
|
||||
- Create an Iterator (by implementing the `std::iter::Iterator` trait) that iterates through the values from `beg` to `end` (including end) in the indicated `steps`. |
||||
|
||||
- The name of you're iterator will be `StepIterator` and it must be generic so you can use any integer value: i8,..,i64, u8,..,u64 or floating point number f32,..,f64 |
||||
|
||||
- If the steps don't allow to arrive until the end of the sequence only the last value inferior to the end of the series will be returned (See Usage) |
||||
|
||||
- Define the associated function: `new` that creates a new Step iterator: |
||||
|
||||
### Expected Functions and Structures |
||||
|
||||
```rust |
||||
pub struct StepIterator<T> { |
||||
... |
||||
} |
||||
|
||||
use std::ops::Add; |
||||
impl StepIterator<T> { |
||||
pub fn new(beg: T, end: T, step: T) -> Self { |
||||
} |
||||
} |
||||
|
||||
impl std::iter::Iterator for StepIterator<T> { |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a program to test your function. |
||||
|
||||
```rust |
||||
fn main() { |
||||
for v in StepIterator::new(0, 100, 10) { |
||||
print!("{},", v); |
||||
} |
||||
println!(); |
||||
|
||||
for v in StepIterator::new(0, 100, 12) { |
||||
print!("{},", v) |
||||
} |
||||
println!(); |
||||
} |
||||
``` |
||||
|
||||
And its output |
||||
|
||||
```console |
||||
student@ubuntu:~/[[ROOT]]/test$ cargo run |
||||
0,10,20,30,40,50,60,70,80,90,100, |
||||
0,12,24,36,48,60,72,84,96, |
||||
student@ubuntu:~/[[ROOT]]/test$ |
||||
``` |
Loading…
Reference in new issue