mirror of https://github.com/01-edu/public.git
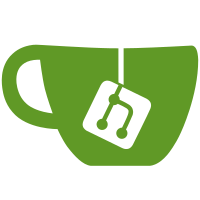
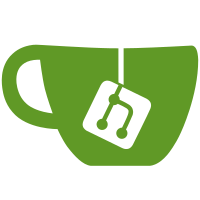
2 changed files with 66 additions and 36 deletions
@ -1,41 +1,54 @@ |
|||||||
/* |
/* |
||||||
## error types |
## changes |
||||||
|
|
||||||
### Instructions |
### Instructions |
||||||
|
|
||||||
Make this code compile |
Imagine you are working in a software to control smart lights in a |
||||||
|
house. You have access to an array of all the lights in a house. |
||||||
|
|
||||||
|
Define the associated function `new` to the data structure `Light` |
||||||
|
which creates a new light with the alias passed in the arguments and |
||||||
|
a brightness of 0. |
||||||
|
|
||||||
|
Define the function `change_brightness` that receives a Vec of lights, |
||||||
|
an alias and a u8 value and sets the u8 value as the new brightness of the light |
||||||
|
identified by the alias in the Vec of lights. |
||||||
*/ |
*/ |
||||||
|
|
||||||
use changes::*; |
use changes::*; |
||||||
|
|
||||||
fn main() { |
fn main() { |
||||||
let mut a = String::from("Hello"); |
// bedroom
|
||||||
|
let mut lights = vec![ |
||||||
println!("the value of `a` before changing {}", a); |
Light::new("living_room"), |
||||||
|
Light::new("bedroom"), |
||||||
add_excitement(&mut a); |
Light::new("rest_room"), |
||||||
|
]; |
||||||
println!("The value of `a` after changing {}", a); |
println!("brightness = {}", lights[0].brightness); |
||||||
|
change_brightness(&mut lights, "living_room", 200); |
||||||
|
println!("new brightness = {}", lights[0].brightness); |
||||||
} |
} |
||||||
|
|
||||||
// fn add_excitement(s: &mut String) {
|
|
||||||
// s.push_str("!");
|
|
||||||
// }
|
|
||||||
|
|
||||||
#[test] |
#[test] |
||||||
fn test_ascii() { |
fn test_unexistente_alias() { |
||||||
let mut expected = "hello".to_string(); |
let mut lights = Vec::new(); |
||||||
add_excitement(&mut expected); |
for i in 0..5 { |
||||||
assert_eq!("hello!", &expected); |
let alias = format!("light-{}", i); |
||||||
let mut expected = "go on".to_string(); |
lights.push(Light::new(&alias)); |
||||||
add_excitement(&mut expected); |
} |
||||||
assert_eq!("go on!", &expected); |
let copy = lights.clone(); |
||||||
|
change_brightness(&mut lights, "light-6", 100); |
||||||
|
assert_eq!(copy, lights); |
||||||
} |
} |
||||||
|
|
||||||
#[test] |
#[test] |
||||||
fn test_unicode() { |
fn test_alias() { |
||||||
let mut expected = "↕".to_string(); |
let mut lights = Vec::new(); |
||||||
add_excitement(&mut expected); |
for i in 0..5 { |
||||||
assert_eq!(expected, "↕!"); |
let alias = format!("light-{}", i); |
||||||
|
lights.push(Light::new(&alias)); |
||||||
|
} |
||||||
|
let alias = "light-3"; |
||||||
|
change_brightness(&mut lights, alias, 100); |
||||||
|
assert_eq!(lights[3].brightness, 100); |
||||||
} |
} |
||||||
|
Loading…
Reference in new issue