mirror of https://github.com/01-edu/public.git
Browse Source
- fix file name - format exercise title - upgrade instructions - add missing import piscine - fix white-sapces and indentationpull/2061/head
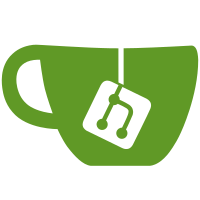
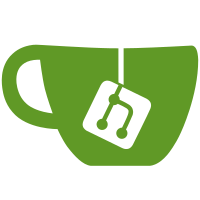
2 changed files with 46 additions and 44 deletions
@ -0,0 +1,46 @@ |
|||||||
|
## digitlen |
||||||
|
|
||||||
|
### Instructions |
||||||
|
|
||||||
|
Write a function `DigitLen()` that takes two integers as arguments and returns the number of digits in the first `int` by the base of the second `int`. |
||||||
|
|
||||||
|
- The second `int` must be between ***2*** and ***36***. If not, the function returns `-1`. |
||||||
|
- If the first `int` is negative, reverse the sign and count the digits. |
||||||
|
|
||||||
|
### Expected function |
||||||
|
|
||||||
|
```go |
||||||
|
func DigitLen(n, base int) int { |
||||||
|
|
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
### Usage |
||||||
|
|
||||||
|
Here is a possible program to test your function: |
||||||
|
|
||||||
|
```go |
||||||
|
package main |
||||||
|
|
||||||
|
import ( |
||||||
|
"fmt" |
||||||
|
"piscine" |
||||||
|
) |
||||||
|
|
||||||
|
func main() { |
||||||
|
fmt.Println(piscine.DigitLen(100, 10)) |
||||||
|
fmt.Println(piscine.DigitLen(100, 2)) |
||||||
|
fmt.Println(piscine.DigitLen(-100, 16)) |
||||||
|
fmt.Println(piscine.DigitLen(100, -1)) |
||||||
|
} |
||||||
|
``` |
||||||
|
|
||||||
|
And its output: |
||||||
|
|
||||||
|
```console |
||||||
|
$ go run . | cat -e |
||||||
|
3 |
||||||
|
7 |
||||||
|
2 |
||||||
|
-1 |
||||||
|
``` |
@ -1,44 +0,0 @@ |
|||||||
## digit-len |
|
||||||
|
|
||||||
### Instructions |
|
||||||
|
|
||||||
Write a function that takes two integers and returns the number of digits in the first integer by the base of the second integer. |
|
||||||
- The second integer must be between 2 and 36. if not, the function returns `-1`. |
|
||||||
- If the first integer is negative , reverse the sign and count the digits. |
|
||||||
|
|
||||||
|
|
||||||
### Expected function |
|
||||||
|
|
||||||
```go |
|
||||||
func DigitLen(n, base int) int { |
|
||||||
|
|
||||||
} |
|
||||||
``` |
|
||||||
|
|
||||||
### Usage |
|
||||||
|
|
||||||
Here is a possible program to test your function : |
|
||||||
|
|
||||||
```go |
|
||||||
package main |
|
||||||
|
|
||||||
import "fmt" |
|
||||||
|
|
||||||
func main(){ |
|
||||||
fmt.Println(DigitLen(100, 10)) |
|
||||||
fmt.Println(DigitLen(100, 2)) |
|
||||||
fmt.Println(DigitLen(-100, 16)) |
|
||||||
fmt.Println(DigitLen(100, -1)) |
|
||||||
|
|
||||||
} |
|
||||||
``` |
|
||||||
|
|
||||||
And its output : |
|
||||||
|
|
||||||
```console |
|
||||||
$ go run . | cat -e |
|
||||||
3 |
|
||||||
7 |
|
||||||
2 |
|
||||||
-1 |
|
||||||
``` |
|
Loading…
Reference in new issue